Read Excel Files in ASP.NET MVC Using IronXL
This tutorial guides developers on implementing Excel file parsing in ASP.NET MVC apps using IronXL.
How to Read Excel File in ASP.NET
Create an ASP.NET Project
Using Visual Studio 2022 (or similar product versions), create a new ASP.NET project. Add additional NuGet packages and source code as needed for the particular project.
Install IronXL Library
Start using IronXL in your project today with a free trial.
After creating the new project, we have to install the IronXL library. Follow the steps below to install the IronXL library. Open the NuGet Package Manager Console and run the following command:
Install-Package IronXL.Excel
Reading Excel file
Open the default controller in your ASP.NET project (e.g., HomeController.cs) and replace the Index
method with the following code:
public ActionResult Index()
{
// Load the Excel workbook from a specified path.
WorkBook workbook = WorkBook.Load(@"C:\Files\Customer Data.xlsx");
// Access the first worksheet from the workbook.
WorkSheet sheet = workbook.WorkSheets.First();
// Convert the worksheet data to a DataTable object.
var dataTable = sheet.ToDataTable();
// Send the DataTable to the view for rendering.
return View(dataTable);
}
public ActionResult Index()
{
// Load the Excel workbook from a specified path.
WorkBook workbook = WorkBook.Load(@"C:\Files\Customer Data.xlsx");
// Access the first worksheet from the workbook.
WorkSheet sheet = workbook.WorkSheets.First();
// Convert the worksheet data to a DataTable object.
var dataTable = sheet.ToDataTable();
// Send the DataTable to the view for rendering.
return View(dataTable);
}
Public Function Index() As ActionResult
' Load the Excel workbook from a specified path.
Dim workbook As WorkBook = WorkBook.Load("C:\Files\Customer Data.xlsx")
' Access the first worksheet from the workbook.
Dim sheet As WorkSheet = workbook.WorkSheets.First()
' Convert the worksheet data to a DataTable object.
Dim dataTable = sheet.ToDataTable()
' Send the DataTable to the view for rendering.
Return View(dataTable)
End Function
In the Index
action method, we load the Excel file using IronXL's Load
method. The path of the Excel file (including the filename) is provided as a parameter to the method call. Next, we select the first Excel sheet as the working sheet and load the data contained in it into a DataTable
object. Lastly, the DataTable
is sent to the frontend.
Display Excel Data on a Web Page
The next example shows how to display the DataTable returned in the previous example in a web browser.
The working Excel file that will be used in this example is depicted below:
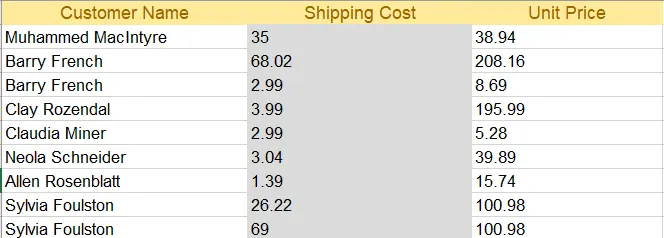
Excel file
Open the index.cshtml (index view) and replace the code with the following HTML code.
@{
ViewData["Title"] = "Home Page";
}
@using System.Data
@model DataTable
<div class="text-center">
<h1 class="display-4">Welcome to IronXL Read Excel MVC</h1>
</div>
<table class="table table-dark">
<tbody>
@foreach (DataRow row in Model.Rows)
{
<tr>
@for (int i = 0; i < Model.Columns.Count; i++)
{
<td>@row[i]</td>
}
</tr>
}
</tbody>
</table>
@{
ViewData["Title"] = "Home Page";
}
@using System.Data
@model DataTable
<div class="text-center">
<h1 class="display-4">Welcome to IronXL Read Excel MVC</h1>
</div>
<table class="table table-dark">
<tbody>
@foreach (DataRow row in Model.Rows)
{
<tr>
@for (int i = 0; i < Model.Columns.Count; i++)
{
<td>@row[i]</td>
}
</tr>
}
</tbody>
</table>
@
If True Then
ViewData("Title") = "Home Page"
End If
'INSTANT VB WARNING: An assignment within expression was extracted from the following statement:
'ORIGINAL LINE: using System.Data model DataTable <div class="text-center"> <h1 class="display-4"> Welcome to IronXL Read Excel MVC</h1> </div> <table class="table table-dark"> <tbody> foreach(DataRow row in Model.Rows)
"display-4"> Welcome [to] IronXL Read Excel MVC</h1> </div> <table class="table table-dark"> (Of tbody) foreach(DataRow row in Model.Rows)
If True Then
'INSTANT VB WARNING: An assignment within expression was extracted from the following statement:
'ORIGINAL LINE: using System.Data model DataTable <div class="text-center"> <h1 class="display-4"> Welcome to IronXL Read Excel MVC</h1> </div> <table class
"text-center"> <h1 class="display-4"> Welcome [to] IronXL Read Excel MVC</h1> </div> <table class
[using] System.Data model DataTable <div class="text-center"> <h1 class
'INSTANT VB TODO TASK: Local functions are not converted by Instant VB:
' (Of tr) @for(int i = 0; i < Model.Columns.Count; i++)
' {
' <td> @row[i]</td>
' }
</tr>
End If
'INSTANT VB TODO TASK: The following line uses invalid syntax:
' </tbody> </table>
The above code uses the DataTable
returned from the Index
method as a model. Each row from the table is printed on the webpage using a @for
loop, including Bootstrap formatting for decoration.
Running the project will produce the results shown below.
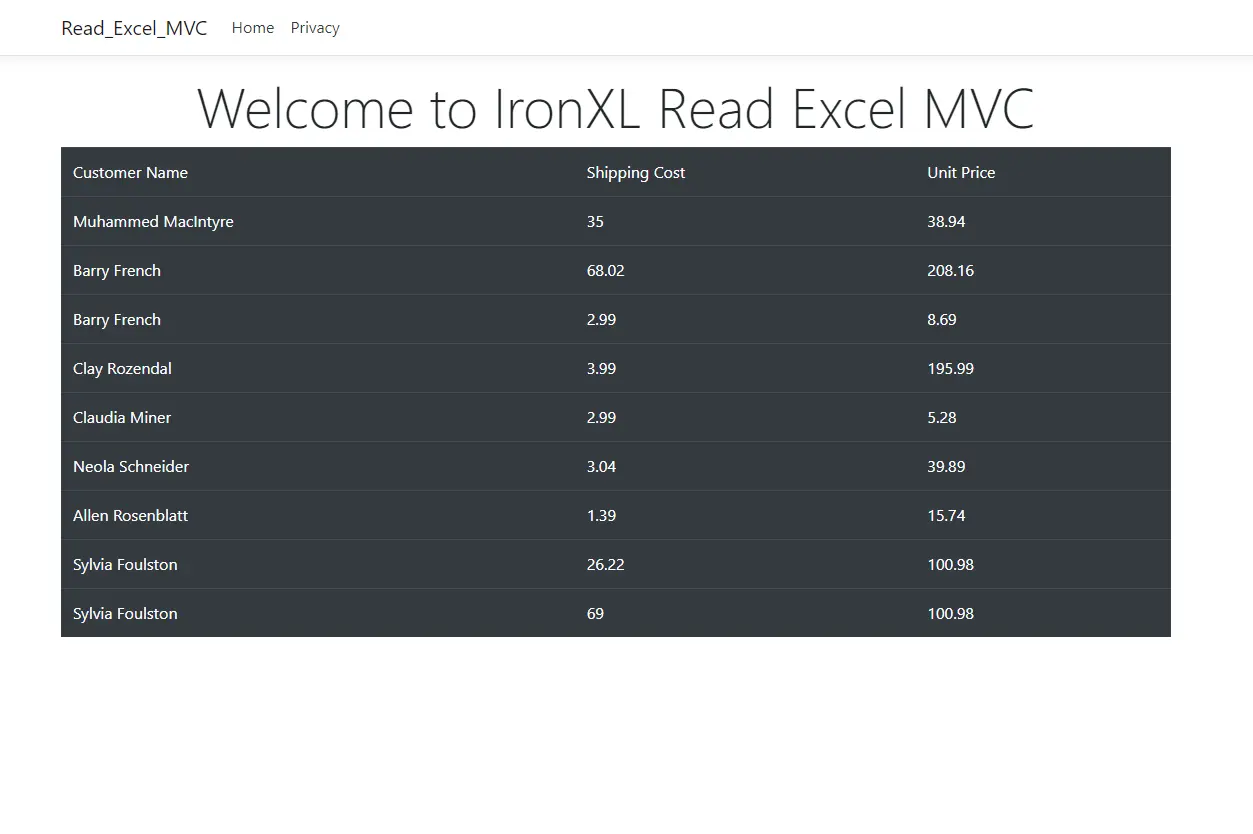
Bootstrap Table
Frequently Asked Questions
What is the purpose of using a library in ASP.NET MVC for Excel operations?
IronXL is used to read and manipulate Excel files in ASP.NET MVC applications without the need for Excel Interop, offering a more efficient and straightforward approach.
How do I install a library for Excel file handling in an ASP.NET project?
To install IronXL, open the NuGet Package Manager Console in Visual Studio and run the command 'Install-Package IronXL.Excel'.
How do I load an Excel file in ASP.NET?
You can load an Excel file using IronXL by calling the 'WorkBook.Load' method with the file path as a parameter.
How can I convert an Excel worksheet to a DataTable in ASP.NET MVC?
After accessing the worksheet with IronXL, you can convert it to a DataTable using the 'ToDataTable' method.
How is Excel data displayed on a web page using ASP.NET MVC?
Excel data is displayed on a web page by iterating over the DataTable rows and columns in a Razor view, and rendering it into an HTML table.
What is the role of the Index method in reading Excel files?
The Index method loads the Excel file, accesses the first worksheet, converts it to a DataTable, and returns it to the view for rendering.
Can I use a library with ASP.NET Core MVC for Excel file operations?
Yes, IronXL can be used with ASP.NET Core MVC to read and manipulate Excel files efficiently.
What are the prerequisites for setting up an ASP.NET MVC project to handle Excel files?
You need to set up a new ASP.NET project in Visual Studio, install the IronXL library via NuGet, and add necessary source code to handle Excel file operations.
How does a library improve Excel file handling in ASP.NET applications?
IronXL simplifies Excel file handling by providing a straightforward API to read, write, and manipulate Excel files without relying on Excel Interop.