Read Barcodes in C#
IronBarcode provides a versatile, advanced, and efficient library for reading barcodes in .NET.
How to Read Barcodes in C# and VB.NET
- Install IronBarcode from NuGet or via DLL download
- Use the
BarcodeReader.Read
method to read any barcode or QR - Read Multiple Barcodes or QRs in a single scan, PDF, or a multiframe Tiff file
- Allow IronBarcode to read from imperfect scans and photos
- Download the tutorial project and get scanning now
Installation
Install with NuGet
Install-Package BarCode
Install with NuGet
Install-Package BarCode
Start using IronPDF in your project today with a free trial.
Check out IronBarcode on Nuget for quick installation and deployment. With over 8 million downloads, it's transforming with C#.
Consider installing the IronBarcode DLL directly. Download and manually install it for your project or GAC form: IronBarCode.zip
IronBarcode provides a versatile, advanced, and efficient library for reading barcodes in .NET.
The first step will be to install Iron Barcode, and this is most easily achieved using our NuGet package, although you may also choose to manually install the DLL to your project or to your global assembly cache. IronBarcode works well to produce a C# Barcode Scanner application.
Install-Package BarCode
Read Your First Barcode
Reading a Barcode or QR Code in .NET is incredibly easy using the IronBarcode class library with .NET Barcode Reader. In our first example, we can see how to read this Barcode with one line of code.
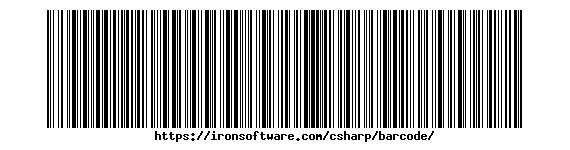
We can extract its value, its image, its encoding type, its binary data (if any), and we can then output that to the console.
:path=/static-assets/barcode/content-code-examples/tutorials/reading-barcodes-1.cs
using IronBarCode;
using System;
// Read barcode
BarcodeResults results = BarcodeReader.Read("GetStarted.png");
// Log the result to Console Window
foreach (BarcodeResult result in results)
{
if (result != null)
{
Console.WriteLine("GetStarted was a success. Read Value: " + result.Text);
}
}
Imports IronBarCode
Imports System
' Read barcode
Private results As BarcodeResults = BarcodeReader.Read("GetStarted.png")
' Log the result to Console Window
For Each result As BarcodeResult In results
If result IsNot Nothing Then
Console.WriteLine("GetStarted was a success. Read Value: " & result.Text)
End If
Next result
Try Harder and Be Specific
In this next example, we will add a barcode scanning option to read a challenging image. The ExtremeDetail value of the Speed enum allows deeper scanning for a barcode that might be obscured, corrupted, or at a skewed angle.
:path=/static-assets/barcode/content-code-examples/tutorials/reading-barcodes-2.cs
using IronBarCode;
BarcodeReaderOptions options = new BarcodeReaderOptions()
{
// Choose a speed from: Faster, Balanced, Detailed, ExtremeDetail
// There is a tradeoff in performance as more Detail is set
Speed = ReadingSpeed.ExtremeDetail,
// By default, all barcode formats are scanned for.
// Specifying one or more, performance will increase.
ExpectBarcodeTypes = BarcodeEncoding.QRCode | BarcodeEncoding.Code128,
};
// Read barcode
BarcodeResults results = BarcodeReader.Read("TryHarderQR.png", options);
Imports IronBarCode
Private options As New BarcodeReaderOptions() With {
.Speed = ReadingSpeed.ExtremeDetail,
.ExpectBarcodeTypes = BarcodeEncoding.QRCode Or BarcodeEncoding.Code128
}
' Read barcode
Private results As BarcodeResults = BarcodeReader.Read("TryHarderQR.png", options)
Which will read this skewed QR Code:
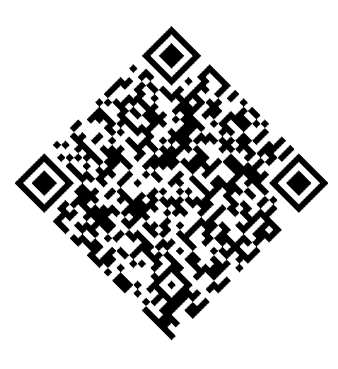
In our example, you can see that we can specify the barcode encoding(s) we are looking for or even multiple formats. Doing so greatly improves barcode reading performance and accuracy. The |
pipe character, or 'Bitwise OR,' is used to specify multiple formats simultaneously.
The same can be achieved, but with a higher degree of specificity, if we move forward and use the ImageFilters and AutoRotate properties.
:path=/static-assets/barcode/content-code-examples/tutorials/reading-barcodes-3.cs
using IronBarCode;
BarcodeReaderOptions options = new BarcodeReaderOptions()
{
// Choose which filters are to be applied (in order)
ImageFilters = new ImageFilterCollection() {
new AdaptiveThresholdFilter(),
},
// Uses machine learning to auto rotate the barcode
AutoRotate = true,
};
// Read barcode
BarcodeResults results = BarcodeReader.Read("TryHarderQR.png", options);
Imports IronBarCode
Private options As New BarcodeReaderOptions() With {
.ImageFilters = New ImageFilterCollection() From {New AdaptiveThresholdFilter()},
.AutoRotate = True
}
' Read barcode
Private results As BarcodeResults = BarcodeReader.Read("TryHarderQR.png", options)
Reading Multiple Barcodes
PDF Documents
In our next example, we are going to look at reading a scanned PDF document and find all of the barcodes of one-dimensional format in very few lines of code.
As you can see, it is very similar to reading a single barcode from a single document, except we now have new information about which page number the barcode was found on.
:path=/static-assets/barcode/content-code-examples/tutorials/reading-barcodes-4.cs
using IronBarCode;
using System;
// Multiple barcodes may be scanned up from a single document or image. A PDF document may also used as the input image
BarcodeResults results = BarcodeReader.ReadPdf("MultipleBarcodes.pdf");
// Work with the results
foreach (var pageResult in results)
{
string Value = pageResult.Value;
int PageNum = pageResult.PageNumber;
System.Drawing.Bitmap Img = pageResult.BarcodeImage;
BarcodeEncoding BarcodeType = pageResult.BarcodeType;
byte[] Binary = pageResult.BinaryValue;
Console.WriteLine(pageResult.Value + " on page " + PageNum);
}
Imports IronBarCode
Imports System
' Multiple barcodes may be scanned up from a single document or image. A PDF document may also used as the input image
Private results As BarcodeResults = BarcodeReader.ReadPdf("MultipleBarcodes.pdf")
' Work with the results
For Each pageResult In results
Dim Value As String = pageResult.Value
Dim PageNum As Integer = pageResult.PageNumber
Dim Img As System.Drawing.Bitmap = pageResult.BarcodeImage
Dim BarcodeType As BarcodeEncoding = pageResult.BarcodeType
Dim Binary() As Byte = pageResult.BinaryValue
Console.WriteLine(pageResult.Value & " on page " & PageNum)
Next pageResult
We find the following barcodes on different pages.
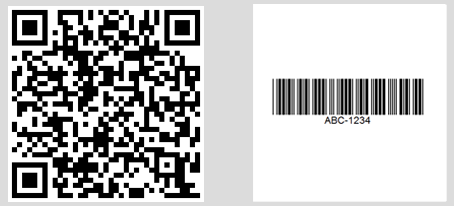
Scans TIFFs
In our next example, we can see that the same result can be obtained from a multi-frame TIFF, which will be treated similarly to a PDF in this context.
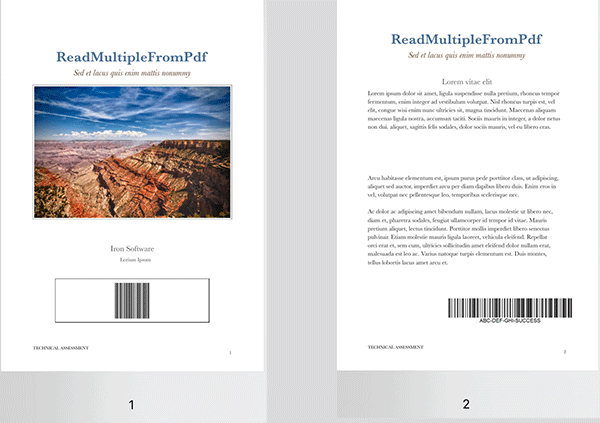
:path=/static-assets/barcode/content-code-examples/tutorials/reading-barcodes-5.cs
using IronBarCode;
// Multi frame TIFF and GIF images can also be scanned
BarcodeResults multiFrameResults = BarcodeReader.Read("Multiframe.tiff");
foreach (var pageResult in multiFrameResults)
{
//...
}
Imports IronBarCode
' Multi frame TIFF and GIF images can also be scanned
Private multiFrameResults As BarcodeResults = BarcodeReader.Read("Multiframe.tiff")
For Each pageResult In multiFrameResults
'...
Next pageResult
MultiThreading
To read multiple documents, we can get better results from IronBarcode by creating a list of the documents and using the BarcodeReader.Read
method. This uses multiple threads and potentially all cores of your CPU for the barcode scanning process and can be exponentially faster than reading barcodes one at a time.
:path=/static-assets/barcode/content-code-examples/tutorials/reading-barcodes-6.cs
using IronBarCode;
// The Multithreaded property allows for faster barcode scanning across multiple images or PDFs. All threads are automatically managed by IronBarCode.
var ListOfDocuments = new[] { "image1.png", "image2.JPG", "image3.pdf" };
BarcodeReaderOptions options = new BarcodeReaderOptions()
{
// Enable multithreading
Multithreaded = true,
};
BarcodeResults batchResults = BarcodeReader.Read(ListOfDocuments, options);
Imports IronBarCode
' The Multithreaded property allows for faster barcode scanning across multiple images or PDFs. All threads are automatically managed by IronBarCode.
Private ListOfDocuments = { "image1.png", "image2.JPG", "image3.pdf" }
Private options As New BarcodeReaderOptions() With {.Multithreaded = True}
Private batchResults As BarcodeResults = BarcodeReader.Read(ListOfDocuments, options)
Summary
In summary, IronBarcode is a versatile .NET software library and C# QR code generator capable of reading a wide range of barcode formats. It can do so regardless of whether the barcodes are perfect screen captures or imperfect real-world images, such as photographs or scans.
Further Reading
To learn more about working with IronBarcode, you may want to explore the other tutorials in this section, as well as the examples on our homepage, which most developers will find sufficient to get started.
Our API Reference, specifically the BarcodeReader class and the BarcodeEncoding enum, will provide detailed information on what you can achieve with this C# barcode library.
Source Code Downloads
We also highly encourage you to download this tutorial and run it yourself. You can do this by downloading the source code or by forking us on GitHub. The source for this .NET barcode reader tutorial is available as a Visual Studio 2017 Console Application project written in C#.