Create, Read and Edit Excel Files in .NET MAUI
Introduction
This How-To Guide explains how to create and read Excel files in .NET MAUI apps for Windows using IronXL. Let's get started.
IronXL: C# Excel Library
IronXL is a C# .NET library for reading, writing and manipulating Excel files. It lets users create Excel documents from scratch, including the content and appearance of Excel, as well as metadata such as the title and author. The library also supports customization features for user interface like setting margins, orientation, page size, images and so on. It does not require any external frameworks, platform integration, or other third-party libraries for generating Excel files. It is self-contained and stand-alone.
How to Read Excel Files in .NET Maui
- Install C# library to read Excel file
- Ensure that all packages required to run MAUI applications are installed
- Create Excel files with intuitive APIs in Maui
- Load and view Excel files in the browser
- Save and export Excel files
Install IronXL
Install with NuGet
Install-Package IronXL.Excel
You can install IronXL using the NuGet Package Manager Console in Visual Studio. Open the Console and enter the following command to install the IronXL library.
Install-Package IronXL.Excel
How To Guide
Creating Excel Files in C# using IronXL
Design the Application Frontend
Open the XAML page named **MainPage.xaml**
and replace the code in it with the following snippet of code.
<?xml version="1.0" encoding="utf-8" ?>
<ContentPage xmlns="http://schemas.microsoft.com/dotnet/2021/maui"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
x:Class="MAUI_IronXL.MainPage">
<ScrollView>
<VerticalStackLayout
Spacing="25"
Padding="30,0"
VerticalOptions="Center">
<Label
Text="Welcome to .NET Multi-platform App UI"
SemanticProperties.HeadingLevel="Level2"
SemanticProperties.Description="Welcome Multi-platform App UI"
FontSize="18"
HorizontalOptions="Center" />
<Button
x:Name="createBtn"
Text="Create Excel File"
SemanticProperties.Hint="Click on the button to create Excel file"
Clicked="CreateExcel"
HorizontalOptions="Center" />
<Button
x:Name="readExcel"
Text="Read and Modify Excel file"
SemanticProperties.Hint="Click on the button to read Excel file"
Clicked="ReadExcel"
HorizontalOptions="Center" />
</VerticalStackLayout>
</ScrollView>
</ContentPage>
The code above creates the layout for our basic .NET MAUI application. It creates one label and two buttons. One button is for creating an Excel file, and the second button provides support to read and modify the Excel file. Both elements are nested in a VerticalStackLayout parent element so that they will appear vertically aligned on all supported devices.
Create Excel Files
It's time to create the Excel file using IronXL. Open the MainPage.xaml.cs
file and write the following method in the file.
private void CreateExcel(object sender, EventArgs e)
{
//Create Workbook
WorkBook workbook = WorkBook.Create(ExcelFileFormat.XLSX);
//Create Worksheet
var sheet = workbook.CreateWorkSheet("2022 Budget");
//Set Cell values
sheet ["A1"].Value = "January";
sheet ["B1"].Value = "February";
sheet ["C1"].Value = "March";
sheet ["D1"].Value = "April";
sheet ["E1"].Value = "May";
sheet ["F1"].Value = "June";
sheet ["G1"].Value = "July";
sheet ["H1"].Value = "August";
//Set Cell input Dynamically
Random r = new Random();
for (int i = 2; i <= 11; i++)
{
sheet ["A" + i].Value = r.Next(1, 1000);
sheet ["B" + i].Value = r.Next(1000, 2000);
sheet ["C" + i].Value = r.Next(2000, 3000);
sheet ["D" + i].Value = r.Next(3000, 4000);
sheet ["E" + i].Value = r.Next(4000, 5000);
sheet ["F" + i].Value = r.Next(5000, 6000);
sheet ["G" + i].Value = r.Next(6000, 7000);
sheet ["H" + i].Value = r.Next(7000, 8000);
}
//Apply formatting like background and border
sheet ["A1:H1"].Style.SetBackgroundColor("#d3d3d3");
sheet ["A1:H1"].Style.TopBorder.SetColor("#000000");
sheet ["A1:H1"].Style.BottomBorder.SetColor("#000000");
sheet ["H2:H11"].Style.RightBorder.SetColor("#000000");
sheet ["H2:H11"].Style.RightBorder.Type = IronXL.Styles.BorderType.Medium;
sheet ["A11:H11"].Style.BottomBorder.SetColor("#000000");
sheet ["A11:H11"].Style.BottomBorder.Type = IronXL.Styles.BorderType.Medium;
//Apply Formulas
decimal sum = sheet ["A2:A11"].Sum();
decimal avg = sheet ["B2:B11"].Avg();
decimal max = sheet ["C2:C11"].Max();
decimal min = sheet ["D2:D11"].Min();
sheet ["A12"].Value = "Sum";
sheet ["B12"].Value = sum;
sheet ["C12"].Value = "Avg";
sheet ["D12"].Value = avg;
sheet ["E12"].Value = "Max";
sheet ["F12"].Value = max;
sheet ["G12"].Value = "Min";
sheet ["H12"].Value = min;
//Save and Open Excel File
SaveService saveService = new SaveService();
saveService.SaveAndView("Budget.xlsx", "application/octet-stream", workbook.ToStream());
}
private void CreateExcel(object sender, EventArgs e)
{
//Create Workbook
WorkBook workbook = WorkBook.Create(ExcelFileFormat.XLSX);
//Create Worksheet
var sheet = workbook.CreateWorkSheet("2022 Budget");
//Set Cell values
sheet ["A1"].Value = "January";
sheet ["B1"].Value = "February";
sheet ["C1"].Value = "March";
sheet ["D1"].Value = "April";
sheet ["E1"].Value = "May";
sheet ["F1"].Value = "June";
sheet ["G1"].Value = "July";
sheet ["H1"].Value = "August";
//Set Cell input Dynamically
Random r = new Random();
for (int i = 2; i <= 11; i++)
{
sheet ["A" + i].Value = r.Next(1, 1000);
sheet ["B" + i].Value = r.Next(1000, 2000);
sheet ["C" + i].Value = r.Next(2000, 3000);
sheet ["D" + i].Value = r.Next(3000, 4000);
sheet ["E" + i].Value = r.Next(4000, 5000);
sheet ["F" + i].Value = r.Next(5000, 6000);
sheet ["G" + i].Value = r.Next(6000, 7000);
sheet ["H" + i].Value = r.Next(7000, 8000);
}
//Apply formatting like background and border
sheet ["A1:H1"].Style.SetBackgroundColor("#d3d3d3");
sheet ["A1:H1"].Style.TopBorder.SetColor("#000000");
sheet ["A1:H1"].Style.BottomBorder.SetColor("#000000");
sheet ["H2:H11"].Style.RightBorder.SetColor("#000000");
sheet ["H2:H11"].Style.RightBorder.Type = IronXL.Styles.BorderType.Medium;
sheet ["A11:H11"].Style.BottomBorder.SetColor("#000000");
sheet ["A11:H11"].Style.BottomBorder.Type = IronXL.Styles.BorderType.Medium;
//Apply Formulas
decimal sum = sheet ["A2:A11"].Sum();
decimal avg = sheet ["B2:B11"].Avg();
decimal max = sheet ["C2:C11"].Max();
decimal min = sheet ["D2:D11"].Min();
sheet ["A12"].Value = "Sum";
sheet ["B12"].Value = sum;
sheet ["C12"].Value = "Avg";
sheet ["D12"].Value = avg;
sheet ["E12"].Value = "Max";
sheet ["F12"].Value = max;
sheet ["G12"].Value = "Min";
sheet ["H12"].Value = min;
//Save and Open Excel File
SaveService saveService = new SaveService();
saveService.SaveAndView("Budget.xlsx", "application/octet-stream", workbook.ToStream());
}
Private Sub CreateExcel(ByVal sender As Object, ByVal e As EventArgs)
'Create Workbook
Dim workbook As WorkBook = WorkBook.Create(ExcelFileFormat.XLSX)
'Create Worksheet
Dim sheet = workbook.CreateWorkSheet("2022 Budget")
'Set Cell values
sheet ("A1").Value = "January"
sheet ("B1").Value = "February"
sheet ("C1").Value = "March"
sheet ("D1").Value = "April"
sheet ("E1").Value = "May"
sheet ("F1").Value = "June"
sheet ("G1").Value = "July"
sheet ("H1").Value = "August"
'Set Cell input Dynamically
Dim r As New Random()
For i As Integer = 2 To 11
sheet ("A" & i).Value = r.Next(1, 1000)
sheet ("B" & i).Value = r.Next(1000, 2000)
sheet ("C" & i).Value = r.Next(2000, 3000)
sheet ("D" & i).Value = r.Next(3000, 4000)
sheet ("E" & i).Value = r.Next(4000, 5000)
sheet ("F" & i).Value = r.Next(5000, 6000)
sheet ("G" & i).Value = r.Next(6000, 7000)
sheet ("H" & i).Value = r.Next(7000, 8000)
Next i
'Apply formatting like background and border
sheet ("A1:H1").Style.SetBackgroundColor("#d3d3d3")
sheet ("A1:H1").Style.TopBorder.SetColor("#000000")
sheet ("A1:H1").Style.BottomBorder.SetColor("#000000")
sheet ("H2:H11").Style.RightBorder.SetColor("#000000")
sheet ("H2:H11").Style.RightBorder.Type = IronXL.Styles.BorderType.Medium
sheet ("A11:H11").Style.BottomBorder.SetColor("#000000")
sheet ("A11:H11").Style.BottomBorder.Type = IronXL.Styles.BorderType.Medium
'Apply Formulas
Dim sum As Decimal = sheet ("A2:A11").Sum()
Dim avg As Decimal = sheet ("B2:B11").Avg()
Dim max As Decimal = sheet ("C2:C11").Max()
Dim min As Decimal = sheet ("D2:D11").Min()
sheet ("A12").Value = "Sum"
sheet ("B12").Value = sum
sheet ("C12").Value = "Avg"
sheet ("D12").Value = avg
sheet ("E12").Value = "Max"
sheet ("F12").Value = max
sheet ("G12").Value = "Min"
sheet ("H12").Value = min
'Save and Open Excel File
Dim saveService As New SaveService()
saveService.SaveAndView("Budget.xlsx", "application/octet-stream", workbook.ToStream())
End Sub
The source code creates a workbook containing one worksheet using IronXL, and then sets the cell values using the "Value" property for each cell.
The style property allows us to add styling and borders to the cells. As shown above, we can apply stylings to a single cell or to a range of cells.
IronXL also supports Excel formulas. Custom Excel formulas can be crafted one or more cells. Furthermore, the values resulting from any Excel formula can be stored in a variable for use later.
The SaveService
class will be used to save and view generated Excel files. This class is declared in the code above, and will be defined formally in a later section.
View Excel Files in the Browser
Open the MainPage.xaml.cs
file and write the following code.
private void ReadExcel(object sender, EventArgs e)
{
//store the path of a file
string filepath="C:\Files\Customer Data.xlsx";
WorkBook workbook = WorkBook.Load(filepath);
WorkSheet sheet = workbook.WorkSheets.First();
decimal sum = sheet ["B2:B10"].Sum();
sheet ["B11"].Value = sum;
sheet ["B11"].Style.SetBackgroundColor("#808080");
sheet ["B11"].Style.Font.SetColor("#ffffff");
//Save and Open Excel File
SaveService saveService = new SaveService();
saveService.SaveAndView("Modified Data.xlsx", "application/octet-stream", workbook.ToStream());
DisplayAlert("Notification", "Excel file has been modified!", "OK");
}
private void ReadExcel(object sender, EventArgs e)
{
//store the path of a file
string filepath="C:\Files\Customer Data.xlsx";
WorkBook workbook = WorkBook.Load(filepath);
WorkSheet sheet = workbook.WorkSheets.First();
decimal sum = sheet ["B2:B10"].Sum();
sheet ["B11"].Value = sum;
sheet ["B11"].Style.SetBackgroundColor("#808080");
sheet ["B11"].Style.Font.SetColor("#ffffff");
//Save and Open Excel File
SaveService saveService = new SaveService();
saveService.SaveAndView("Modified Data.xlsx", "application/octet-stream", workbook.ToStream());
DisplayAlert("Notification", "Excel file has been modified!", "OK");
}
Private Sub ReadExcel(ByVal sender As Object, ByVal e As EventArgs)
'store the path of a file
Dim filepath As String="C:\Files\Customer Data.xlsx"
Dim workbook As WorkBook = WorkBook.Load(filepath)
Dim sheet As WorkSheet = workbook.WorkSheets.First()
Dim sum As Decimal = sheet ("B2:B10").Sum()
sheet ("B11").Value = sum
sheet ("B11").Style.SetBackgroundColor("#808080")
sheet ("B11").Style.Font.SetColor("#ffffff")
'Save and Open Excel File
Dim saveService As New SaveService()
saveService.SaveAndView("Modified Data.xlsx", "application/octet-stream", workbook.ToStream())
DisplayAlert("Notification", "Excel file has been modified!", "OK")
End Sub
The source code loads the Excel file, applies the formula on a range of cells, and formats it with custom background and text colorings. Afterwards, the Excel file is transferred to the user's browser as a a stream of bytes. Additionally, the DisplayAlert
method presents a message indicating that the file has been opened and modified.
Save Excel Files
In this section, we will now define the SaveService
class that we referenced in the previous two sections that will save our Excel files in local storage.
Create a "SaveService.cs" class and write the following code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace MAUI_IronXL
{
public partial class SaveService
{
public partial void SaveAndView(string fileName, string contentType, MemoryStream stream);
}
}
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace MAUI_IronXL
{
public partial class SaveService
{
public partial void SaveAndView(string fileName, string contentType, MemoryStream stream);
}
}
Imports System
Imports System.Collections.Generic
Imports System.Linq
Imports System.Text
Imports System.Threading.Tasks
Namespace MAUI_IronXL
Partial Public Class SaveService
Public Partial Private Sub SaveAndView(ByVal fileName As String, ByVal contentType As String, ByVal stream As MemoryStream)
End Sub
End Class
End Namespace
Next, create a class named "SaveWindows.cs" inside the Platforms > Windows folder, and add the code shown below to it.
using Windows.Storage;
using Windows.Storage.Pickers;
using Windows.Storage.Streams;
using Windows.UI.Popups;
namespace MAUI_IronXL
{
public partial class SaveService
{
public async partial void SaveAndView(string fileName, string contentType, MemoryStream stream)
{
StorageFile stFile;
string extension = Path.GetExtension(fileName);
//Gets process windows handle to open the dialog in application process.
IntPtr windowHandle = System.Diagnostics.Process.GetCurrentProcess().MainWindowHandle;
if (!Windows.Foundation.Metadata.ApiInformation.IsTypePresent("Windows.Phone.UI.Input.HardwareButtons"))
{
//Creates file save picker to save a file.
FileSavePicker savePicker = new FileSavePicker();
savePicker.DefaultFileExtension = ".xlsx";
savePicker.SuggestedFileName = fileName;
//Saves the file as Excel file.
savePicker.FileTypeChoices.Add("XLSX", new List<string>() { ".xlsx" });
WinRT.Interop.InitializeWithWindow.Initialize(savePicker, windowHandle);
stFile = await savePicker.PickSaveFileAsync();
}
else
{
StorageFolder local = ApplicationData.Current.LocalFolder;
stFile = await local.CreateFileAsync(fileName, CreationCollisionOption.ReplaceExisting);
}
if (stFile != null)
{
using (IRandomAccessStream zipStream = await stFile.OpenAsync(FileAccessMode.ReadWrite))
{
//Writes compressed data from memory to file.
using(Stream outstream = zipStream.AsStreamForWrite())
{
outstream.SetLength(0);
//Saves the stream as file.
byte [] buffer = outstream.ToArray();
outstream.Write(buffer, 0, buffer.Length);
outstream.Flush();
}
}
//Create message dialog box.
MessageDialog msgDialog = new("Do you want to view the document?", "File has been created successfully");
UICommand yesCmd = new("Yes");
msgDialog.Commands.Add(yesCmd);
UICommand noCmd = new("No");
msgDialog.Commands.Add(noCmd);
WinRT.Interop.InitializeWithWindow.Initialize(msgDialog, windowHandle);
//Showing a dialog box.
IUICommand cmd = await msgDialog.ShowAsync();
if (cmd.Label == yesCmd.Label)
{
//Launch the saved file.
await Windows.System.Launcher.LaunchFileAsync(stFile);
}
}
}
}
}
using Windows.Storage;
using Windows.Storage.Pickers;
using Windows.Storage.Streams;
using Windows.UI.Popups;
namespace MAUI_IronXL
{
public partial class SaveService
{
public async partial void SaveAndView(string fileName, string contentType, MemoryStream stream)
{
StorageFile stFile;
string extension = Path.GetExtension(fileName);
//Gets process windows handle to open the dialog in application process.
IntPtr windowHandle = System.Diagnostics.Process.GetCurrentProcess().MainWindowHandle;
if (!Windows.Foundation.Metadata.ApiInformation.IsTypePresent("Windows.Phone.UI.Input.HardwareButtons"))
{
//Creates file save picker to save a file.
FileSavePicker savePicker = new FileSavePicker();
savePicker.DefaultFileExtension = ".xlsx";
savePicker.SuggestedFileName = fileName;
//Saves the file as Excel file.
savePicker.FileTypeChoices.Add("XLSX", new List<string>() { ".xlsx" });
WinRT.Interop.InitializeWithWindow.Initialize(savePicker, windowHandle);
stFile = await savePicker.PickSaveFileAsync();
}
else
{
StorageFolder local = ApplicationData.Current.LocalFolder;
stFile = await local.CreateFileAsync(fileName, CreationCollisionOption.ReplaceExisting);
}
if (stFile != null)
{
using (IRandomAccessStream zipStream = await stFile.OpenAsync(FileAccessMode.ReadWrite))
{
//Writes compressed data from memory to file.
using(Stream outstream = zipStream.AsStreamForWrite())
{
outstream.SetLength(0);
//Saves the stream as file.
byte [] buffer = outstream.ToArray();
outstream.Write(buffer, 0, buffer.Length);
outstream.Flush();
}
}
//Create message dialog box.
MessageDialog msgDialog = new("Do you want to view the document?", "File has been created successfully");
UICommand yesCmd = new("Yes");
msgDialog.Commands.Add(yesCmd);
UICommand noCmd = new("No");
msgDialog.Commands.Add(noCmd);
WinRT.Interop.InitializeWithWindow.Initialize(msgDialog, windowHandle);
//Showing a dialog box.
IUICommand cmd = await msgDialog.ShowAsync();
if (cmd.Label == yesCmd.Label)
{
//Launch the saved file.
await Windows.System.Launcher.LaunchFileAsync(stFile);
}
}
}
}
}
Imports Windows.Storage
Imports Windows.Storage.Pickers
Imports Windows.Storage.Streams
Imports Windows.UI.Popups
Namespace MAUI_IronXL
Partial Public Class SaveService
Public Async Sub SaveAndView(ByVal fileName As String, ByVal contentType As String, ByVal stream As MemoryStream)
Dim stFile As StorageFile
Dim extension As String = Path.GetExtension(fileName)
'Gets process windows handle to open the dialog in application process.
Dim windowHandle As IntPtr = System.Diagnostics.Process.GetCurrentProcess().MainWindowHandle
If Not Windows.Foundation.Metadata.ApiInformation.IsTypePresent("Windows.Phone.UI.Input.HardwareButtons") Then
'Creates file save picker to save a file.
Dim savePicker As New FileSavePicker()
savePicker.DefaultFileExtension = ".xlsx"
savePicker.SuggestedFileName = fileName
'Saves the file as Excel file.
savePicker.FileTypeChoices.Add("XLSX", New List(Of String)() From {".xlsx"})
WinRT.Interop.InitializeWithWindow.Initialize(savePicker, windowHandle)
stFile = Await savePicker.PickSaveFileAsync()
Else
Dim local As StorageFolder = ApplicationData.Current.LocalFolder
stFile = Await local.CreateFileAsync(fileName, CreationCollisionOption.ReplaceExisting)
End If
If stFile IsNot Nothing Then
Using zipStream As IRandomAccessStream = Await stFile.OpenAsync(FileAccessMode.ReadWrite)
'Writes compressed data from memory to file.
Using outstream As Stream = zipStream.AsStreamForWrite()
outstream.SetLength(0)
'Saves the stream as file.
Dim buffer() As Byte = outstream.ToArray()
outstream.Write(buffer, 0, buffer.Length)
outstream.Flush()
End Using
End Using
'Create message dialog box.
Dim msgDialog As New MessageDialog("Do you want to view the document?", "File has been created successfully")
Dim yesCmd As New UICommand("Yes")
msgDialog.Commands.Add(yesCmd)
Dim noCmd As New UICommand("No")
msgDialog.Commands.Add(noCmd)
WinRT.Interop.InitializeWithWindow.Initialize(msgDialog, windowHandle)
'Showing a dialog box.
Dim cmd As IUICommand = Await msgDialog.ShowAsync()
If cmd.Label = yesCmd.Label Then
'Launch the saved file.
Await Windows.System.Launcher.LaunchFileAsync(stFile)
End If
End If
End Sub
End Class
End Namespace
Output
Build and run the MAUI project. On successful execution, a window will open showing the content depicted in the image below.
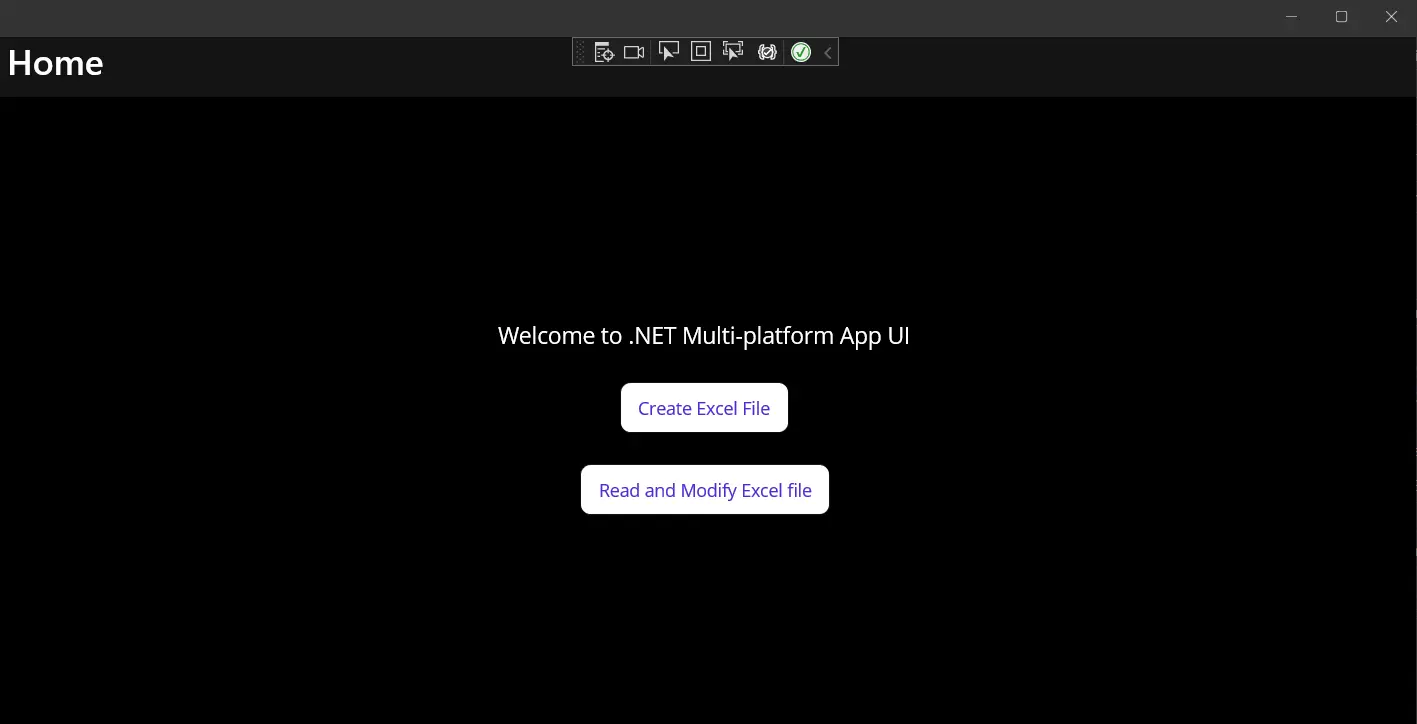
Figure 1 - Output
Clicking on the "Create Excel File" button will open a separate dialog window. This window prompts users to choose a location and a filename by which to save a new (generated) Excel file. Specify the location and filename as directed, and click OK. Afterward, another dialog window will appear.
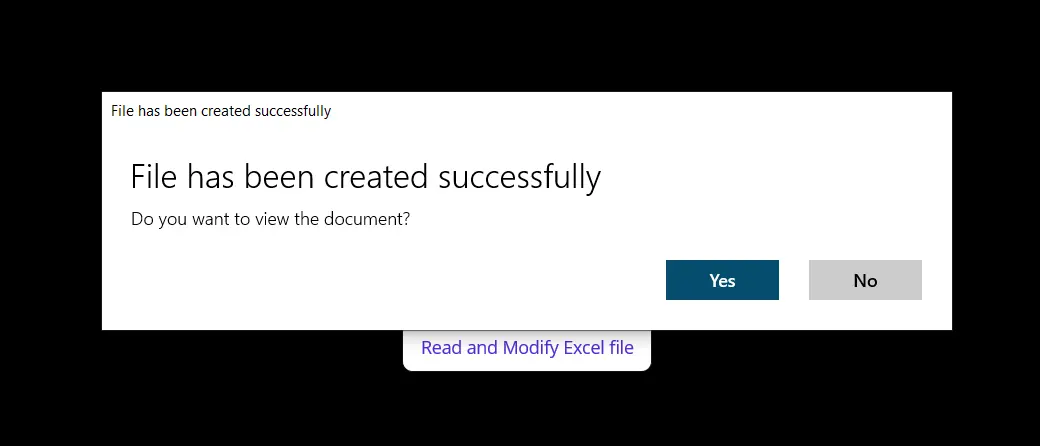
Figure 2 - Create Excel Popup
Opening the Excel file as directed in the popup will bring up a document as shown in the screenshot below.
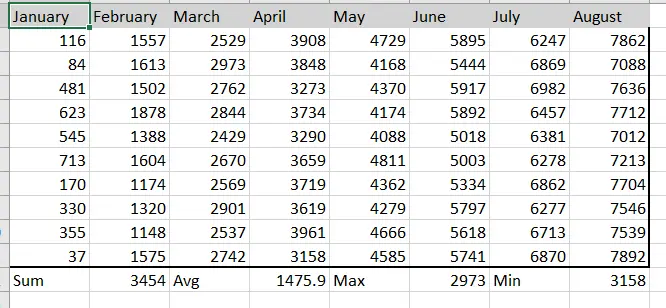
Figure 3 - Read and Modify Excel Popup
Clicking on the "Read and Modify Excel File" button will load the previously generated Excel file and modify it with the custom background and text colors that we defined in an earlier section.
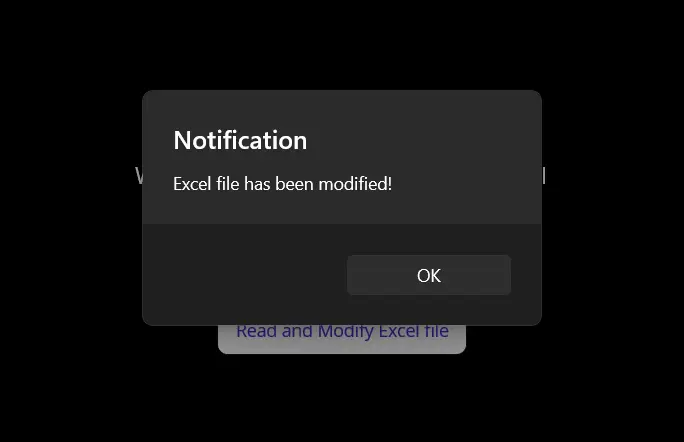
Figure 4 - Excel Output
When you open the modified file, you'll see the following output with table of contents.
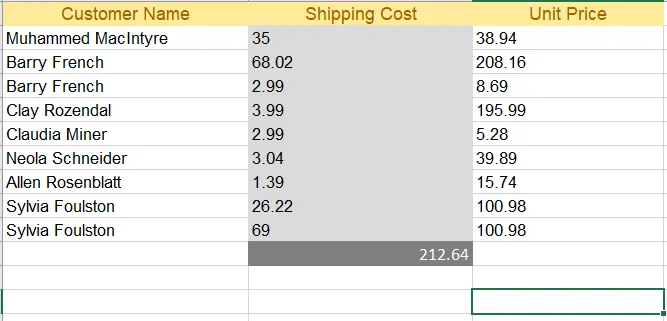
Figure 5 - Modified Excel Output
Conclusion
This explained how we can create, read and modify the Excel files in the .NET MAUI application using the IronXL library. IronXL performs very well and does all operations with speed and accuracy. IronXL is an excellent library for Excel operations. It is far better than Microsoft Interop as it doesn't require any installation of the Microsoft Office Suite on the machine. In addition, IronXL supports multiple operations like creating workbooks and worksheets, working with cell ranges, formatting, and exporting to various document types like CSV, TSV, and many more.
IronXL supports all project templates like Windows Form, WPF, ASP.NET Core and many others. Refer to our tutorials for creating Excel files and reading Excel files for additional information about how to use IronXL.
Quick Access Links
Explore this How-To Guide on GitHub
The source code for this project is available on GitHub.
Use this code as an easy way to get up and running in just a few minutes. The project is saved as a Microsoft Visual Studio 2022 project, but is compatible with any .NET IDE.
How to Read, Create, and Edit Excel Files in .NET MAUI AppsView the API Reference
Explore the API Reference for IronXL, outlining the details of all of IronXL’s features, namespaces, classes, methods fields and enums.
View the API Reference