Crear, Leer y Editar Archivos Excel en .NET MAUI
Introducción
*Esta guía explica cómo crear y leer archivos Excel en aplicaciones .NET MAUI para Windows usando IronXL. Empecemos.
IronXL: C# Biblioteca Excel
IronXL es una biblioteca C# .NET para leer, escribir y manipular archivos Excel. Permite a los usuarios crear documentos Excel desde cero, incluyendo su contenido y apariencia, así como metadatos como el título y el autor. La biblioteca también admite funciones de personalización de la interfaz de usuario, como la configuración de márgenes, orientación, tamaño de página, imágenes, etc. No requiere ningún marco externo, integración de plataformas u otras bibliotecas de terceros para generar archivos Excel. Es autónomo e independiente.
Cómo leer archivos Excel en .NET Maui
- Instalar la biblioteca C# para leer archivos Excel
- Asegúrese de que todos los paquetes necesarios para ejecutar aplicaciones MAUI están instalados
- Crea archivos de Excel con API intuitivas en Maui
- Cargar y ver archivos Excel en el navegador
- Guardar y exportar archivos Excel
Instalar IronXL
Comience a usar IronXL en su proyecto hoy con una prueba gratuita.
Puedes instalar IronXL utilizando la consola del gestor de paquetes NuGet en Visual Studio. Abra la consola e introduzca el siguiente comando para instalar la biblioteca IronXL.
Install-Package IronXL.Excel
Guía práctica
Creación de archivos Excel en C# usando IronXL
Diseñar el frontend de la aplicación
Abra la página XAML llamada **MainPage.xaml**
y reemplace el código en ella con el siguiente fragmento de código.
<?xml version="1.0" encoding="utf-8" ?>
<ContentPage xmlns="http://schemas.microsoft.com/dotnet/2021/maui"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
x:Class="MAUI_IronXL.MainPage">
<ScrollView>
<VerticalStackLayout
Spacing="25"
Padding="30,0"
VerticalOptions="Center">
<Label
Text="Welcome to .NET Multi-platform App UI"
SemanticProperties.HeadingLevel="Level2"
SemanticProperties.Description="Welcome Multi-platform App UI"
FontSize="18"
HorizontalOptions="Center" />
<Button
x:Name="createBtn"
Text="Create Excel File"
SemanticProperties.Hint="Click on the button to create Excel file"
Clicked="CreateExcel"
HorizontalOptions="Center" />
<Button
x:Name="readExcel"
Text="Read and Modify Excel file"
SemanticProperties.Hint="Click on the button to read Excel file"
Clicked="ReadExcel"
HorizontalOptions="Center" />
</VerticalStackLayout>
</ScrollView>
</ContentPage>
<?xml version="1.0" encoding="utf-8" ?>
<ContentPage xmlns="http://schemas.microsoft.com/dotnet/2021/maui"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
x:Class="MAUI_IronXL.MainPage">
<ScrollView>
<VerticalStackLayout
Spacing="25"
Padding="30,0"
VerticalOptions="Center">
<Label
Text="Welcome to .NET Multi-platform App UI"
SemanticProperties.HeadingLevel="Level2"
SemanticProperties.Description="Welcome Multi-platform App UI"
FontSize="18"
HorizontalOptions="Center" />
<Button
x:Name="createBtn"
Text="Create Excel File"
SemanticProperties.Hint="Click on the button to create Excel file"
Clicked="CreateExcel"
HorizontalOptions="Center" />
<Button
x:Name="readExcel"
Text="Read and Modify Excel file"
SemanticProperties.Hint="Click on the button to read Excel file"
Clicked="ReadExcel"
HorizontalOptions="Center" />
</VerticalStackLayout>
</ScrollView>
</ContentPage>
El código anterior crea el diseño para nuestra aplicación básica .NET MAUI. Crea una etiqueta y dos botones. Un botón sirve para crear un archivo Excel y el segundo permite leer y modificar el archivo Excel. Ambos elementos están anidados en un elemento padre VerticalStackLayout para que aparezcan alineados verticalmente en todos los dispositivos compatibles.
Crear archivos Excel
Es hora de crear el archivo Excel utilizando IronXL. Abra el archivo MainPage.xaml.cs
y escriba el siguiente método en el archivo.
private void CreateExcel(object sender, EventArgs e)
{
//Create Workbook
WorkBook workbook = WorkBook.Create(ExcelFileFormat.XLSX);
//Create Worksheet
var sheet = workbook.CreateWorkSheet("2022 Budget");
//Set Cell values
sheet ["A1"].Value = "January";
sheet ["B1"].Value = "February";
sheet ["C1"].Value = "March";
sheet ["D1"].Value = "April";
sheet ["E1"].Value = "May";
sheet ["F1"].Value = "June";
sheet ["G1"].Value = "July";
sheet ["H1"].Value = "August";
//Set Cell input Dynamically
Random r = new Random();
for (int i = 2; i <= 11; i++)
{
sheet ["A" + i].Value = r.Next(1, 1000);
sheet ["B" + i].Value = r.Next(1000, 2000);
sheet ["C" + i].Value = r.Next(2000, 3000);
sheet ["D" + i].Value = r.Next(3000, 4000);
sheet ["E" + i].Value = r.Next(4000, 5000);
sheet ["F" + i].Value = r.Next(5000, 6000);
sheet ["G" + i].Value = r.Next(6000, 7000);
sheet ["H" + i].Value = r.Next(7000, 8000);
}
//Apply formatting like background and border
sheet ["A1:H1"].Style.SetBackgroundColor("#d3d3d3");
sheet ["A1:H1"].Style.TopBorder.SetColor("#000000");
sheet ["A1:H1"].Style.BottomBorder.SetColor("#000000");
sheet ["H2:H11"].Style.RightBorder.SetColor("#000000");
sheet ["H2:H11"].Style.RightBorder.Type = IronXL.Styles.BorderType.Medium;
sheet ["A11:H11"].Style.BottomBorder.SetColor("#000000");
sheet ["A11:H11"].Style.BottomBorder.Type = IronXL.Styles.BorderType.Medium;
//Apply Formulas
decimal sum = sheet ["A2:A11"].Sum();
decimal avg = sheet ["B2:B11"].Avg();
decimal max = sheet ["C2:C11"].Max();
decimal min = sheet ["D2:D11"].Min();
sheet ["A12"].Value = "Sum";
sheet ["B12"].Value = sum;
sheet ["C12"].Value = "Avg";
sheet ["D12"].Value = avg;
sheet ["E12"].Value = "Max";
sheet ["F12"].Value = max;
sheet ["G12"].Value = "Min";
sheet ["H12"].Value = min;
//Save and Open Excel File
SaveService saveService = new SaveService();
saveService.SaveAndView("Budget.xlsx", "application/octet-stream", workbook.ToStream());
}
private void CreateExcel(object sender, EventArgs e)
{
//Create Workbook
WorkBook workbook = WorkBook.Create(ExcelFileFormat.XLSX);
//Create Worksheet
var sheet = workbook.CreateWorkSheet("2022 Budget");
//Set Cell values
sheet ["A1"].Value = "January";
sheet ["B1"].Value = "February";
sheet ["C1"].Value = "March";
sheet ["D1"].Value = "April";
sheet ["E1"].Value = "May";
sheet ["F1"].Value = "June";
sheet ["G1"].Value = "July";
sheet ["H1"].Value = "August";
//Set Cell input Dynamically
Random r = new Random();
for (int i = 2; i <= 11; i++)
{
sheet ["A" + i].Value = r.Next(1, 1000);
sheet ["B" + i].Value = r.Next(1000, 2000);
sheet ["C" + i].Value = r.Next(2000, 3000);
sheet ["D" + i].Value = r.Next(3000, 4000);
sheet ["E" + i].Value = r.Next(4000, 5000);
sheet ["F" + i].Value = r.Next(5000, 6000);
sheet ["G" + i].Value = r.Next(6000, 7000);
sheet ["H" + i].Value = r.Next(7000, 8000);
}
//Apply formatting like background and border
sheet ["A1:H1"].Style.SetBackgroundColor("#d3d3d3");
sheet ["A1:H1"].Style.TopBorder.SetColor("#000000");
sheet ["A1:H1"].Style.BottomBorder.SetColor("#000000");
sheet ["H2:H11"].Style.RightBorder.SetColor("#000000");
sheet ["H2:H11"].Style.RightBorder.Type = IronXL.Styles.BorderType.Medium;
sheet ["A11:H11"].Style.BottomBorder.SetColor("#000000");
sheet ["A11:H11"].Style.BottomBorder.Type = IronXL.Styles.BorderType.Medium;
//Apply Formulas
decimal sum = sheet ["A2:A11"].Sum();
decimal avg = sheet ["B2:B11"].Avg();
decimal max = sheet ["C2:C11"].Max();
decimal min = sheet ["D2:D11"].Min();
sheet ["A12"].Value = "Sum";
sheet ["B12"].Value = sum;
sheet ["C12"].Value = "Avg";
sheet ["D12"].Value = avg;
sheet ["E12"].Value = "Max";
sheet ["F12"].Value = max;
sheet ["G12"].Value = "Min";
sheet ["H12"].Value = min;
//Save and Open Excel File
SaveService saveService = new SaveService();
saveService.SaveAndView("Budget.xlsx", "application/octet-stream", workbook.ToStream());
}
Private Sub CreateExcel(ByVal sender As Object, ByVal e As EventArgs)
'Create Workbook
Dim workbook As WorkBook = WorkBook.Create(ExcelFileFormat.XLSX)
'Create Worksheet
Dim sheet = workbook.CreateWorkSheet("2022 Budget")
'Set Cell values
sheet ("A1").Value = "January"
sheet ("B1").Value = "February"
sheet ("C1").Value = "March"
sheet ("D1").Value = "April"
sheet ("E1").Value = "May"
sheet ("F1").Value = "June"
sheet ("G1").Value = "July"
sheet ("H1").Value = "August"
'Set Cell input Dynamically
Dim r As New Random()
For i As Integer = 2 To 11
sheet ("A" & i).Value = r.Next(1, 1000)
sheet ("B" & i).Value = r.Next(1000, 2000)
sheet ("C" & i).Value = r.Next(2000, 3000)
sheet ("D" & i).Value = r.Next(3000, 4000)
sheet ("E" & i).Value = r.Next(4000, 5000)
sheet ("F" & i).Value = r.Next(5000, 6000)
sheet ("G" & i).Value = r.Next(6000, 7000)
sheet ("H" & i).Value = r.Next(7000, 8000)
Next i
'Apply formatting like background and border
sheet ("A1:H1").Style.SetBackgroundColor("#d3d3d3")
sheet ("A1:H1").Style.TopBorder.SetColor("#000000")
sheet ("A1:H1").Style.BottomBorder.SetColor("#000000")
sheet ("H2:H11").Style.RightBorder.SetColor("#000000")
sheet ("H2:H11").Style.RightBorder.Type = IronXL.Styles.BorderType.Medium
sheet ("A11:H11").Style.BottomBorder.SetColor("#000000")
sheet ("A11:H11").Style.BottomBorder.Type = IronXL.Styles.BorderType.Medium
'Apply Formulas
Dim sum As Decimal = sheet ("A2:A11").Sum()
Dim avg As Decimal = sheet ("B2:B11").Avg()
Dim max As Decimal = sheet ("C2:C11").Max()
Dim min As Decimal = sheet ("D2:D11").Min()
sheet ("A12").Value = "Sum"
sheet ("B12").Value = sum
sheet ("C12").Value = "Avg"
sheet ("D12").Value = avg
sheet ("E12").Value = "Max"
sheet ("F12").Value = max
sheet ("G12").Value = "Min"
sheet ("H12").Value = min
'Save and Open Excel File
Dim saveService As New SaveService()
saveService.SaveAndView("Budget.xlsx", "application/octet-stream", workbook.ToStream())
End Sub
El código fuente crea un libro de trabajo que contiene una hoja de cálculo utilizando IronXL, y luego establece los valores de las celdas utilizando la propiedad "Valor" para cada celda.
La propiedad style nos permite añadir estilos y bordes a las celdas. Como se muestra arriba, podemos aplicar estilos a una sola celda o a un rango de celdas.
IronXL también admite fórmulas de Excel. Las fórmulas personalizadas de Excel pueden elaborarse en una o varias celdas. Además, los valores resultantes de cualquier fórmula de Excel pueden almacenarse en una variable para su uso posterior.
La clase SaveService
se utilizará para guardar y ver archivos de Excel generados. Esta clase se declara en el código anterior y se definirá formalmente en una sección posterior.
Ver archivos Excel en el navegador
Abre el archivo MainPage.xaml.cs
y escribe el siguiente código.
private void ReadExcel(object sender, EventArgs e)
{
//store the path of a file
string filepath="C:\Files\Customer Data.xlsx";
WorkBook workbook = WorkBook.Load(filepath);
WorkSheet sheet = workbook.WorkSheets.First();
decimal sum = sheet ["B2:B10"].Sum();
sheet ["B11"].Value = sum;
sheet ["B11"].Style.SetBackgroundColor("#808080");
sheet ["B11"].Style.Font.SetColor("#ffffff");
//Save and Open Excel File
SaveService saveService = new SaveService();
saveService.SaveAndView("Modified Data.xlsx", "application/octet-stream", workbook.ToStream());
DisplayAlert("Notification", "Excel file has been modified!", "OK");
}
private void ReadExcel(object sender, EventArgs e)
{
//store the path of a file
string filepath="C:\Files\Customer Data.xlsx";
WorkBook workbook = WorkBook.Load(filepath);
WorkSheet sheet = workbook.WorkSheets.First();
decimal sum = sheet ["B2:B10"].Sum();
sheet ["B11"].Value = sum;
sheet ["B11"].Style.SetBackgroundColor("#808080");
sheet ["B11"].Style.Font.SetColor("#ffffff");
//Save and Open Excel File
SaveService saveService = new SaveService();
saveService.SaveAndView("Modified Data.xlsx", "application/octet-stream", workbook.ToStream());
DisplayAlert("Notification", "Excel file has been modified!", "OK");
}
Private Sub ReadExcel(ByVal sender As Object, ByVal e As EventArgs)
'store the path of a file
Dim filepath As String="C:\Files\Customer Data.xlsx"
Dim workbook As WorkBook = WorkBook.Load(filepath)
Dim sheet As WorkSheet = workbook.WorkSheets.First()
Dim sum As Decimal = sheet ("B2:B10").Sum()
sheet ("B11").Value = sum
sheet ("B11").Style.SetBackgroundColor("#808080")
sheet ("B11").Style.Font.SetColor("#ffffff")
'Save and Open Excel File
Dim saveService As New SaveService()
saveService.SaveAndView("Modified Data.xlsx", "application/octet-stream", workbook.ToStream())
DisplayAlert("Notification", "Excel file has been modified!", "OK")
End Sub
El código fuente carga el archivo de Excel, aplica la fórmula a un rango de celdas y le da formato con colores de fondo y texto personalizados. Después, el archivo Excel se transfiere al navegador del usuario como un flujo de bytes. Además, el método DisplayAlert
presenta un mensaje indicando que el archivo ha sido abierto y modificado.
Guardar archivos Excel
En esta sección, ahora definiremos la clase SaveService
que mencionamos en las dos secciones anteriores, la cual guardará nuestros archivos de Excel en el almacenamiento local.
Crea una clase "SaveService.cs" y escribe el siguiente código:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace MAUI_IronXL
{
public partial class SaveService
{
public partial void SaveAndView(string fileName, string contentType, MemoryStream stream);
}
}
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace MAUI_IronXL
{
public partial class SaveService
{
public partial void SaveAndView(string fileName, string contentType, MemoryStream stream);
}
}
Imports System
Imports System.Collections.Generic
Imports System.Linq
Imports System.Text
Imports System.Threading.Tasks
Namespace MAUI_IronXL
Partial Public Class SaveService
Public Partial Private Sub SaveAndView(ByVal fileName As String, ByVal contentType As String, ByVal stream As MemoryStream)
End Sub
End Class
End Namespace
A continuación, cree una clase llamada "SaveWindows.cs" dentro de la carpeta Platforms > Windows, y añádale el código que se muestra a continuación.
using Windows.Storage;
using Windows.Storage.Pickers;
using Windows.Storage.Streams;
using Windows.UI.Popups;
namespace MAUI_IronXL
{
public partial class SaveService
{
public async partial void SaveAndView(string fileName, string contentType, MemoryStream stream)
{
StorageFile stFile;
string extension = Path.GetExtension(fileName);
//Gets process windows handle to open the dialog in application process.
IntPtr windowHandle = System.Diagnostics.Process.GetCurrentProcess().MainWindowHandle;
if (!Windows.Foundation.Metadata.ApiInformation.IsTypePresent("Windows.Phone.UI.Input.HardwareButtons"))
{
//Creates file save picker to save a file.
FileSavePicker savePicker = new FileSavePicker();
savePicker.DefaultFileExtension = ".xlsx";
savePicker.SuggestedFileName = fileName;
//Saves the file as Excel file.
savePicker.FileTypeChoices.Add("XLSX", new List<string>() { ".xlsx" });
WinRT.Interop.InitializeWithWindow.Initialize(savePicker, windowHandle);
stFile = await savePicker.PickSaveFileAsync();
}
else
{
StorageFolder local = ApplicationData.Current.LocalFolder;
stFile = await local.CreateFileAsync(fileName, CreationCollisionOption.ReplaceExisting);
}
if (stFile != null)
{
using (IRandomAccessStream zipStream = await stFile.OpenAsync(FileAccessMode.ReadWrite))
{
//Writes compressed data from memory to file.
using(Stream outstream = zipStream.AsStreamForWrite())
{
outstream.SetLength(0);
//Saves the stream as file.
byte [] buffer = outstream.ToArray();
outstream.Write(buffer, 0, buffer.Length);
outstream.Flush();
}
}
//Create message dialog box.
MessageDialog msgDialog = new("Do you want to view the document?", "File has been created successfully");
UICommand yesCmd = new("Yes");
msgDialog.Commands.Add(yesCmd);
UICommand noCmd = new("No");
msgDialog.Commands.Add(noCmd);
WinRT.Interop.InitializeWithWindow.Initialize(msgDialog, windowHandle);
//Showing a dialog box.
IUICommand cmd = await msgDialog.ShowAsync();
if (cmd.Label == yesCmd.Label)
{
//Launch the saved file.
await Windows.System.Launcher.LaunchFileAsync(stFile);
}
}
}
}
}
using Windows.Storage;
using Windows.Storage.Pickers;
using Windows.Storage.Streams;
using Windows.UI.Popups;
namespace MAUI_IronXL
{
public partial class SaveService
{
public async partial void SaveAndView(string fileName, string contentType, MemoryStream stream)
{
StorageFile stFile;
string extension = Path.GetExtension(fileName);
//Gets process windows handle to open the dialog in application process.
IntPtr windowHandle = System.Diagnostics.Process.GetCurrentProcess().MainWindowHandle;
if (!Windows.Foundation.Metadata.ApiInformation.IsTypePresent("Windows.Phone.UI.Input.HardwareButtons"))
{
//Creates file save picker to save a file.
FileSavePicker savePicker = new FileSavePicker();
savePicker.DefaultFileExtension = ".xlsx";
savePicker.SuggestedFileName = fileName;
//Saves the file as Excel file.
savePicker.FileTypeChoices.Add("XLSX", new List<string>() { ".xlsx" });
WinRT.Interop.InitializeWithWindow.Initialize(savePicker, windowHandle);
stFile = await savePicker.PickSaveFileAsync();
}
else
{
StorageFolder local = ApplicationData.Current.LocalFolder;
stFile = await local.CreateFileAsync(fileName, CreationCollisionOption.ReplaceExisting);
}
if (stFile != null)
{
using (IRandomAccessStream zipStream = await stFile.OpenAsync(FileAccessMode.ReadWrite))
{
//Writes compressed data from memory to file.
using(Stream outstream = zipStream.AsStreamForWrite())
{
outstream.SetLength(0);
//Saves the stream as file.
byte [] buffer = outstream.ToArray();
outstream.Write(buffer, 0, buffer.Length);
outstream.Flush();
}
}
//Create message dialog box.
MessageDialog msgDialog = new("Do you want to view the document?", "File has been created successfully");
UICommand yesCmd = new("Yes");
msgDialog.Commands.Add(yesCmd);
UICommand noCmd = new("No");
msgDialog.Commands.Add(noCmd);
WinRT.Interop.InitializeWithWindow.Initialize(msgDialog, windowHandle);
//Showing a dialog box.
IUICommand cmd = await msgDialog.ShowAsync();
if (cmd.Label == yesCmd.Label)
{
//Launch the saved file.
await Windows.System.Launcher.LaunchFileAsync(stFile);
}
}
}
}
}
Imports Windows.Storage
Imports Windows.Storage.Pickers
Imports Windows.Storage.Streams
Imports Windows.UI.Popups
Namespace MAUI_IronXL
Partial Public Class SaveService
Public Async Sub SaveAndView(ByVal fileName As String, ByVal contentType As String, ByVal stream As MemoryStream)
Dim stFile As StorageFile
Dim extension As String = Path.GetExtension(fileName)
'Gets process windows handle to open the dialog in application process.
Dim windowHandle As IntPtr = System.Diagnostics.Process.GetCurrentProcess().MainWindowHandle
If Not Windows.Foundation.Metadata.ApiInformation.IsTypePresent("Windows.Phone.UI.Input.HardwareButtons") Then
'Creates file save picker to save a file.
Dim savePicker As New FileSavePicker()
savePicker.DefaultFileExtension = ".xlsx"
savePicker.SuggestedFileName = fileName
'Saves the file as Excel file.
savePicker.FileTypeChoices.Add("XLSX", New List(Of String)() From {".xlsx"})
WinRT.Interop.InitializeWithWindow.Initialize(savePicker, windowHandle)
stFile = Await savePicker.PickSaveFileAsync()
Else
Dim local As StorageFolder = ApplicationData.Current.LocalFolder
stFile = Await local.CreateFileAsync(fileName, CreationCollisionOption.ReplaceExisting)
End If
If stFile IsNot Nothing Then
Using zipStream As IRandomAccessStream = Await stFile.OpenAsync(FileAccessMode.ReadWrite)
'Writes compressed data from memory to file.
Using outstream As Stream = zipStream.AsStreamForWrite()
outstream.SetLength(0)
'Saves the stream as file.
Dim buffer() As Byte = outstream.ToArray()
outstream.Write(buffer, 0, buffer.Length)
outstream.Flush()
End Using
End Using
'Create message dialog box.
Dim msgDialog As New MessageDialog("Do you want to view the document?", "File has been created successfully")
Dim yesCmd As New UICommand("Yes")
msgDialog.Commands.Add(yesCmd)
Dim noCmd As New UICommand("No")
msgDialog.Commands.Add(noCmd)
WinRT.Interop.InitializeWithWindow.Initialize(msgDialog, windowHandle)
'Showing a dialog box.
Dim cmd As IUICommand = Await msgDialog.ShowAsync()
If cmd.Label = yesCmd.Label Then
'Launch the saved file.
Await Windows.System.Launcher.LaunchFileAsync(stFile)
End If
End If
End Sub
End Class
End Namespace
Salida
Construye y ejecuta el proyecto MAUI. Si se ejecuta correctamente, se abrirá una ventana con el contenido que se muestra en la siguiente imagen.
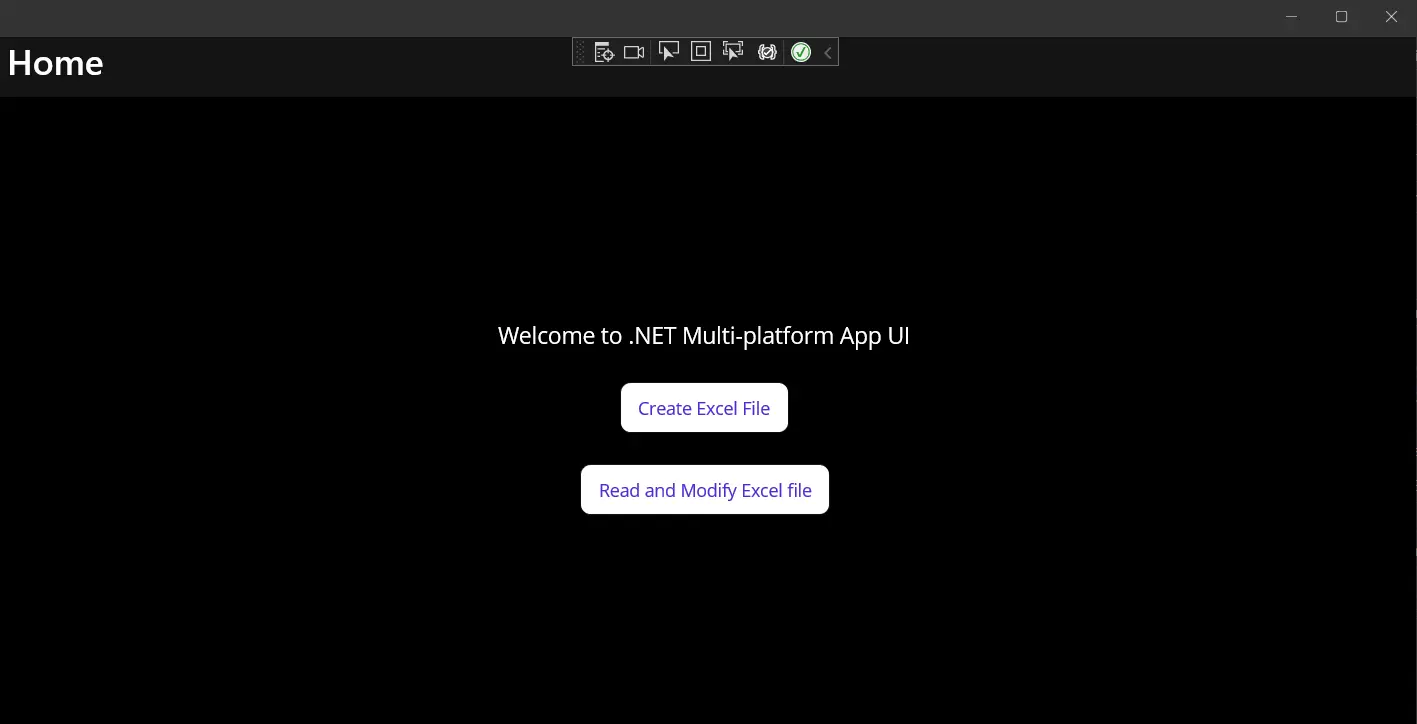
Figura 1 - Salida
Al hacer clic en el botón "Crear archivo Excel" se abrirá otra ventana de diálogo. Esta ventana solicita a los usuarios que elijan una ubicación y un nombre de archivo para guardar un nuevo archivo de Excel (generado). Especifique la ubicación y el nombre de archivo según lo indicado, y haga clic en OK. A continuación, aparecerá otra ventana de diálogo.
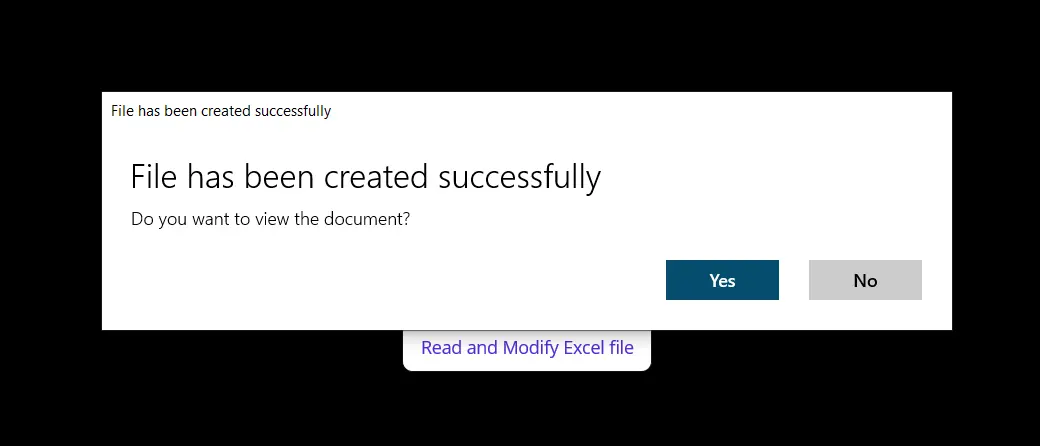
Figura 2 - Crear ventana emergente de Excel
Al abrir el archivo Excel como se indica en la ventana emergente, aparecerá un documento como el que se muestra en la siguiente captura de pantalla.
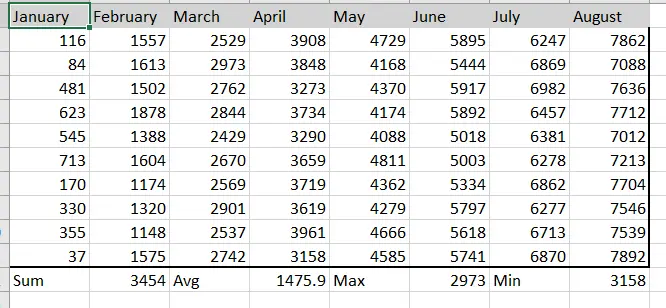
Figura 3 - Leer y Modificar Ventana Emergente de Excel
Al hacer clic en el botón "Leer y modificar archivo Excel" se cargará el archivo Excel generado previamente y se modificará con los colores de fondo y texto personalizados que definimos en una sección anterior.
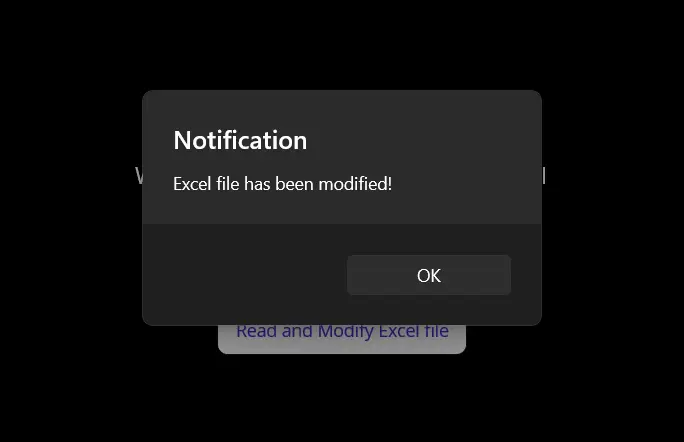
Figura 4 - Salida de Excel
Cuando abra el archivo modificado, verá la siguiente salida con la tabla de contenidos.
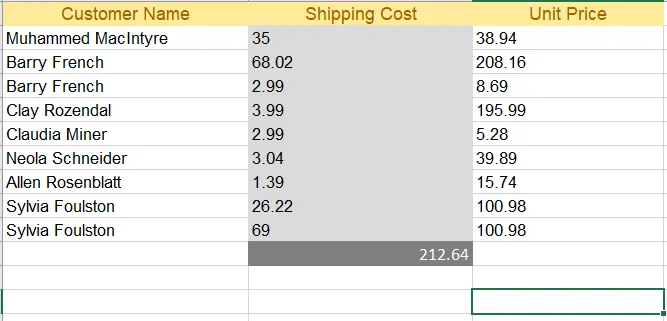
Figura 5 - Salida de Excel Modificada
Conclusión
Esto explica como podemos crear, leer y modificar los archivos Excel en la aplicación .NET MAUI usando la librería IronXL. IronXL funciona muy bien y realiza todas las operaciones con rapidez y precisión. IronXL es una excelente biblioteca para operaciones en Excel. Es mucho mejor que Microsoft Interop, ya que no requiere ninguna instalación del paquete Microsoft Office en la máquina. Además, IronXL soporta múltiples operaciones como crear libros y hojas de trabajo, trabajar con rangos de celdas, formatear y exportar a varios tipos de documentos como CSV, TSV y muchos más.
IronXL soporta todas las plantillas de proyecto como Windows Form, WPF, ASP.NET Core y muchas otras. Consulte nuestros tutoriales para leer archivos Excel para obtener información adicional sobre cómo usar IronXL.
Enlaces de acceso rápido
Explore esta guía práctica en GitHub
El código fuente de este proyecto está disponible en GitHub.
Utilice este código como una manera fácil de ponerse en marcha en tan sólo unos minutos. El proyecto se guarda como un proyecto de Microsoft Visual Studio 2022, pero es compatible con cualquier IDE .NET.
Cómo Leer, Crear y Editar Archivos de Excel en Aplicaciones .NET MAUIVer la referencia de la API
Explore la Referencia de la API para IronXL, que describe los detalles de todas las características, espacios de nombres, clases, métodos, campos y enums de IronXL.
Ver la referencia de la API