在 .NET MAUI 中建立、讀取和編輯 Excel 文件
介紹
*本操作指南解針如何在Windows的.NET MAUI應用中使用IronXL創建和讀取Excel文件。 讓我們開始吧。
IronXL:C# Excel 函式庫
IronXL 是一個用於讀取、寫入和操作 Excel 檔案的 C# .NET 函式庫。 它允許用戶從頭創建Excel文檔,包括Excel的內容和外觀,以及標題和作者等元數據。 此庫還支援用戶介面的自定義功能,例如設置邊距、方向、頁面大小、圖片等。 它不需要任何外部框架、平台整合或其他第三方庫來生成Excel文件。 它是自足且独立的。
如何在 .NET Maui 中閱讀 Excel 檔案
- 安裝C#程式庫以讀取Excel檔案
- 確保安裝所有運行 MAUI 應用所需的套件
- 使用直觀的 API 在 Maui 中創建 Excel 檔案
- 在瀏覽器中載入和查看 Excel 檔案
- 儲存和匯出 Excel 文件
安裝 IronXL
立即在您的專案中使用IronXL,並享受免費試用。
您可以在 Visual Studio 中使用 NuGet 包管理控制台安裝 IronXL。 打開控制台並輸入以下命令來安裝 IronXL 函式庫。
Install-Package IronXL.Excel
使用指南
使用 IronXL 在 C# 中創建 Excel 文件
設計應用程式前端
打開名為 **MainPage.xaml**
的 XAML 頁面,並將其中的代碼替換為以下代碼片段。
<?xml version="1.0" encoding="utf-8" ?>
<ContentPage xmlns="http://schemas.microsoft.com/dotnet/2021/maui"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
x:Class="MAUI_IronXL.MainPage">
<ScrollView>
<VerticalStackLayout
Spacing="25"
Padding="30,0"
VerticalOptions="Center">
<Label
Text="Welcome to .NET Multi-platform App UI"
SemanticProperties.HeadingLevel="Level2"
SemanticProperties.Description="Welcome Multi-platform App UI"
FontSize="18"
HorizontalOptions="Center" />
<Button
x:Name="createBtn"
Text="Create Excel File"
SemanticProperties.Hint="Click on the button to create Excel file"
Clicked="CreateExcel"
HorizontalOptions="Center" />
<Button
x:Name="readExcel"
Text="Read and Modify Excel file"
SemanticProperties.Hint="Click on the button to read Excel file"
Clicked="ReadExcel"
HorizontalOptions="Center" />
</VerticalStackLayout>
</ScrollView>
</ContentPage>
上述代碼建立了我們基本的 .NET MAUI 應用程式的版面配置。 它創建一個標籤和兩個按鈕。 一個按鈕用於創建Excel文件,另一個按鈕則提供支持讀取和修改Excel文件。這兩個元素都嵌套在VerticalStackLayout父元素中,因此它們將在所有支持的設備上垂直對齊。
建立 Excel 檔案
是時候使用IronXL創建Excel文件了。 打開 MainPage.xaml.cs
檔案並在檔案中寫下以下方法。
private void CreateExcel(object sender, EventArgs e)
{
//Create Workbook
WorkBook workbook = WorkBook.Create(ExcelFileFormat.XLSX);
//Create Worksheet
var sheet = workbook.CreateWorkSheet("2022 Budget");
//Set Cell values
sheet ["A1"].Value = "January";
sheet ["B1"].Value = "February";
sheet ["C1"].Value = "March";
sheet ["D1"].Value = "April";
sheet ["E1"].Value = "May";
sheet ["F1"].Value = "June";
sheet ["G1"].Value = "July";
sheet ["H1"].Value = "August";
//Set Cell input Dynamically
Random r = new Random();
for (int i = 2; i <= 11; i++)
{
sheet ["A" + i].Value = r.Next(1, 1000);
sheet ["B" + i].Value = r.Next(1000, 2000);
sheet ["C" + i].Value = r.Next(2000, 3000);
sheet ["D" + i].Value = r.Next(3000, 4000);
sheet ["E" + i].Value = r.Next(4000, 5000);
sheet ["F" + i].Value = r.Next(5000, 6000);
sheet ["G" + i].Value = r.Next(6000, 7000);
sheet ["H" + i].Value = r.Next(7000, 8000);
}
//Apply formatting like background and border
sheet ["A1:H1"].Style.SetBackgroundColor("#d3d3d3");
sheet ["A1:H1"].Style.TopBorder.SetColor("#000000");
sheet ["A1:H1"].Style.BottomBorder.SetColor("#000000");
sheet ["H2:H11"].Style.RightBorder.SetColor("#000000");
sheet ["H2:H11"].Style.RightBorder.Type = IronXL.Styles.BorderType.Medium;
sheet ["A11:H11"].Style.BottomBorder.SetColor("#000000");
sheet ["A11:H11"].Style.BottomBorder.Type = IronXL.Styles.BorderType.Medium;
//Apply Formulas
decimal sum = sheet ["A2:A11"].Sum();
decimal avg = sheet ["B2:B11"].Avg();
decimal max = sheet ["C2:C11"].Max();
decimal min = sheet ["D2:D11"].Min();
sheet ["A12"].Value = "Sum";
sheet ["B12"].Value = sum;
sheet ["C12"].Value = "Avg";
sheet ["D12"].Value = avg;
sheet ["E12"].Value = "Max";
sheet ["F12"].Value = max;
sheet ["G12"].Value = "Min";
sheet ["H12"].Value = min;
//Save and Open Excel File
SaveService saveService = new SaveService();
saveService.SaveAndView("Budget.xlsx", "application/octet-stream", workbook.ToStream());
}
private void CreateExcel(object sender, EventArgs e)
{
//Create Workbook
WorkBook workbook = WorkBook.Create(ExcelFileFormat.XLSX);
//Create Worksheet
var sheet = workbook.CreateWorkSheet("2022 Budget");
//Set Cell values
sheet ["A1"].Value = "January";
sheet ["B1"].Value = "February";
sheet ["C1"].Value = "March";
sheet ["D1"].Value = "April";
sheet ["E1"].Value = "May";
sheet ["F1"].Value = "June";
sheet ["G1"].Value = "July";
sheet ["H1"].Value = "August";
//Set Cell input Dynamically
Random r = new Random();
for (int i = 2; i <= 11; i++)
{
sheet ["A" + i].Value = r.Next(1, 1000);
sheet ["B" + i].Value = r.Next(1000, 2000);
sheet ["C" + i].Value = r.Next(2000, 3000);
sheet ["D" + i].Value = r.Next(3000, 4000);
sheet ["E" + i].Value = r.Next(4000, 5000);
sheet ["F" + i].Value = r.Next(5000, 6000);
sheet ["G" + i].Value = r.Next(6000, 7000);
sheet ["H" + i].Value = r.Next(7000, 8000);
}
//Apply formatting like background and border
sheet ["A1:H1"].Style.SetBackgroundColor("#d3d3d3");
sheet ["A1:H1"].Style.TopBorder.SetColor("#000000");
sheet ["A1:H1"].Style.BottomBorder.SetColor("#000000");
sheet ["H2:H11"].Style.RightBorder.SetColor("#000000");
sheet ["H2:H11"].Style.RightBorder.Type = IronXL.Styles.BorderType.Medium;
sheet ["A11:H11"].Style.BottomBorder.SetColor("#000000");
sheet ["A11:H11"].Style.BottomBorder.Type = IronXL.Styles.BorderType.Medium;
//Apply Formulas
decimal sum = sheet ["A2:A11"].Sum();
decimal avg = sheet ["B2:B11"].Avg();
decimal max = sheet ["C2:C11"].Max();
decimal min = sheet ["D2:D11"].Min();
sheet ["A12"].Value = "Sum";
sheet ["B12"].Value = sum;
sheet ["C12"].Value = "Avg";
sheet ["D12"].Value = avg;
sheet ["E12"].Value = "Max";
sheet ["F12"].Value = max;
sheet ["G12"].Value = "Min";
sheet ["H12"].Value = min;
//Save and Open Excel File
SaveService saveService = new SaveService();
saveService.SaveAndView("Budget.xlsx", "application/octet-stream", workbook.ToStream());
}
Private Sub CreateExcel(ByVal sender As Object, ByVal e As EventArgs)
'Create Workbook
Dim workbook As WorkBook = WorkBook.Create(ExcelFileFormat.XLSX)
'Create Worksheet
Dim sheet = workbook.CreateWorkSheet("2022 Budget")
'Set Cell values
sheet ("A1").Value = "January"
sheet ("B1").Value = "February"
sheet ("C1").Value = "March"
sheet ("D1").Value = "April"
sheet ("E1").Value = "May"
sheet ("F1").Value = "June"
sheet ("G1").Value = "July"
sheet ("H1").Value = "August"
'Set Cell input Dynamically
Dim r As New Random()
For i As Integer = 2 To 11
sheet ("A" & i).Value = r.Next(1, 1000)
sheet ("B" & i).Value = r.Next(1000, 2000)
sheet ("C" & i).Value = r.Next(2000, 3000)
sheet ("D" & i).Value = r.Next(3000, 4000)
sheet ("E" & i).Value = r.Next(4000, 5000)
sheet ("F" & i).Value = r.Next(5000, 6000)
sheet ("G" & i).Value = r.Next(6000, 7000)
sheet ("H" & i).Value = r.Next(7000, 8000)
Next i
'Apply formatting like background and border
sheet ("A1:H1").Style.SetBackgroundColor("#d3d3d3")
sheet ("A1:H1").Style.TopBorder.SetColor("#000000")
sheet ("A1:H1").Style.BottomBorder.SetColor("#000000")
sheet ("H2:H11").Style.RightBorder.SetColor("#000000")
sheet ("H2:H11").Style.RightBorder.Type = IronXL.Styles.BorderType.Medium
sheet ("A11:H11").Style.BottomBorder.SetColor("#000000")
sheet ("A11:H11").Style.BottomBorder.Type = IronXL.Styles.BorderType.Medium
'Apply Formulas
Dim sum As Decimal = sheet ("A2:A11").Sum()
Dim avg As Decimal = sheet ("B2:B11").Avg()
Dim max As Decimal = sheet ("C2:C11").Max()
Dim min As Decimal = sheet ("D2:D11").Min()
sheet ("A12").Value = "Sum"
sheet ("B12").Value = sum
sheet ("C12").Value = "Avg"
sheet ("D12").Value = avg
sheet ("E12").Value = "Max"
sheet ("F12").Value = max
sheet ("G12").Value = "Min"
sheet ("H12").Value = min
'Save and Open Excel File
Dim saveService As New SaveService()
saveService.SaveAndView("Budget.xlsx", "application/octet-stream", workbook.ToStream())
End Sub
源代碼使用IronXL創建了一個包含一個工作表的工作簿,然後使用每個單元格的“Value”屬性設置單元格值。
style 屬性允許我們為單元格添加樣式和邊框。 如上所示,我們可以對單個儲存格或一系列儲存格應用樣式。
IronXL 也支持 Excel 公式。 自定義的Excel公式可以針對一個或多個單元格設計。 此外,任何 Excel 公式產生的值都可以存儲在變量中,以供日後使用。
SaveService
類將用於保存和查看生成的 Excel 文件。 此類別在上述代碼中已聲明,並將在後續部分正式定義。
在瀏覽器中查看 Excel 文件
打開 MainPage.xaml.cs
文件並寫下以下代碼。
private void ReadExcel(object sender, EventArgs e)
{
//store the path of a file
string filepath="C:\Files\Customer Data.xlsx";
WorkBook workbook = WorkBook.Load(filepath);
WorkSheet sheet = workbook.WorkSheets.First();
decimal sum = sheet ["B2:B10"].Sum();
sheet ["B11"].Value = sum;
sheet ["B11"].Style.SetBackgroundColor("#808080");
sheet ["B11"].Style.Font.SetColor("#ffffff");
//Save and Open Excel File
SaveService saveService = new SaveService();
saveService.SaveAndView("Modified Data.xlsx", "application/octet-stream", workbook.ToStream());
DisplayAlert("Notification", "Excel file has been modified!", "OK");
}
private void ReadExcel(object sender, EventArgs e)
{
//store the path of a file
string filepath="C:\Files\Customer Data.xlsx";
WorkBook workbook = WorkBook.Load(filepath);
WorkSheet sheet = workbook.WorkSheets.First();
decimal sum = sheet ["B2:B10"].Sum();
sheet ["B11"].Value = sum;
sheet ["B11"].Style.SetBackgroundColor("#808080");
sheet ["B11"].Style.Font.SetColor("#ffffff");
//Save and Open Excel File
SaveService saveService = new SaveService();
saveService.SaveAndView("Modified Data.xlsx", "application/octet-stream", workbook.ToStream());
DisplayAlert("Notification", "Excel file has been modified!", "OK");
}
Private Sub ReadExcel(ByVal sender As Object, ByVal e As EventArgs)
'store the path of a file
Dim filepath As String="C:\Files\Customer Data.xlsx"
Dim workbook As WorkBook = WorkBook.Load(filepath)
Dim sheet As WorkSheet = workbook.WorkSheets.First()
Dim sum As Decimal = sheet ("B2:B10").Sum()
sheet ("B11").Value = sum
sheet ("B11").Style.SetBackgroundColor("#808080")
sheet ("B11").Style.Font.SetColor("#ffffff")
'Save and Open Excel File
Dim saveService As New SaveService()
saveService.SaveAndView("Modified Data.xlsx", "application/octet-stream", workbook.ToStream())
DisplayAlert("Notification", "Excel file has been modified!", "OK")
End Sub
源代碼加載Excel文件,在一系列單元格上應用公式,並用自定義背景和文字顏色進行格式設定。 然後,Excel 文件將作為字節流傳輸到用戶的瀏覽器。 此外,DisplayAlert
方法顯示一則訊息,指出檔案已被開啟並修改。
保存 Excel 文件
在本節中,我們將定義前兩節中提到的 SaveService
類,該類將把我們的 Excel 文件保存在本地存儲中。
創建一個名為 "SaveService.cs" 的類別,並編寫以下代碼:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace MAUI_IronXL
{
public partial class SaveService
{
public partial void SaveAndView(string fileName, string contentType, MemoryStream stream);
}
}
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace MAUI_IronXL
{
public partial class SaveService
{
public partial void SaveAndView(string fileName, string contentType, MemoryStream stream);
}
}
Imports System
Imports System.Collections.Generic
Imports System.Linq
Imports System.Text
Imports System.Threading.Tasks
Namespace MAUI_IronXL
Partial Public Class SaveService
Public Partial Private Sub SaveAndView(ByVal fileName As String, ByVal contentType As String, ByVal stream As MemoryStream)
End Sub
End Class
End Namespace
接下來,在 Platforms > Windows 資料夾內建立一個名為 "SaveWindows.cs" 的類別,並將以下程式碼加入其中。
using Windows.Storage;
using Windows.Storage.Pickers;
using Windows.Storage.Streams;
using Windows.UI.Popups;
namespace MAUI_IronXL
{
public partial class SaveService
{
public async partial void SaveAndView(string fileName, string contentType, MemoryStream stream)
{
StorageFile stFile;
string extension = Path.GetExtension(fileName);
//Gets process windows handle to open the dialog in application process.
IntPtr windowHandle = System.Diagnostics.Process.GetCurrentProcess().MainWindowHandle;
if (!Windows.Foundation.Metadata.ApiInformation.IsTypePresent("Windows.Phone.UI.Input.HardwareButtons"))
{
//Creates file save picker to save a file.
FileSavePicker savePicker = new FileSavePicker();
savePicker.DefaultFileExtension = ".xlsx";
savePicker.SuggestedFileName = fileName;
//Saves the file as Excel file.
savePicker.FileTypeChoices.Add("XLSX", new List<string>() { ".xlsx" });
WinRT.Interop.InitializeWithWindow.Initialize(savePicker, windowHandle);
stFile = await savePicker.PickSaveFileAsync();
}
else
{
StorageFolder local = ApplicationData.Current.LocalFolder;
stFile = await local.CreateFileAsync(fileName, CreationCollisionOption.ReplaceExisting);
}
if (stFile != null)
{
using (IRandomAccessStream zipStream = await stFile.OpenAsync(FileAccessMode.ReadWrite))
{
//Writes compressed data from memory to file.
using(Stream outstream = zipStream.AsStreamForWrite())
{
outstream.SetLength(0);
//Saves the stream as file.
byte [] buffer = outstream.ToArray();
outstream.Write(buffer, 0, buffer.Length);
outstream.Flush();
}
}
//Create message dialog box.
MessageDialog msgDialog = new("Do you want to view the document?", "File has been created successfully");
UICommand yesCmd = new("Yes");
msgDialog.Commands.Add(yesCmd);
UICommand noCmd = new("No");
msgDialog.Commands.Add(noCmd);
WinRT.Interop.InitializeWithWindow.Initialize(msgDialog, windowHandle);
//Showing a dialog box.
IUICommand cmd = await msgDialog.ShowAsync();
if (cmd.Label == yesCmd.Label)
{
//Launch the saved file.
await Windows.System.Launcher.LaunchFileAsync(stFile);
}
}
}
}
}
using Windows.Storage;
using Windows.Storage.Pickers;
using Windows.Storage.Streams;
using Windows.UI.Popups;
namespace MAUI_IronXL
{
public partial class SaveService
{
public async partial void SaveAndView(string fileName, string contentType, MemoryStream stream)
{
StorageFile stFile;
string extension = Path.GetExtension(fileName);
//Gets process windows handle to open the dialog in application process.
IntPtr windowHandle = System.Diagnostics.Process.GetCurrentProcess().MainWindowHandle;
if (!Windows.Foundation.Metadata.ApiInformation.IsTypePresent("Windows.Phone.UI.Input.HardwareButtons"))
{
//Creates file save picker to save a file.
FileSavePicker savePicker = new FileSavePicker();
savePicker.DefaultFileExtension = ".xlsx";
savePicker.SuggestedFileName = fileName;
//Saves the file as Excel file.
savePicker.FileTypeChoices.Add("XLSX", new List<string>() { ".xlsx" });
WinRT.Interop.InitializeWithWindow.Initialize(savePicker, windowHandle);
stFile = await savePicker.PickSaveFileAsync();
}
else
{
StorageFolder local = ApplicationData.Current.LocalFolder;
stFile = await local.CreateFileAsync(fileName, CreationCollisionOption.ReplaceExisting);
}
if (stFile != null)
{
using (IRandomAccessStream zipStream = await stFile.OpenAsync(FileAccessMode.ReadWrite))
{
//Writes compressed data from memory to file.
using(Stream outstream = zipStream.AsStreamForWrite())
{
outstream.SetLength(0);
//Saves the stream as file.
byte [] buffer = outstream.ToArray();
outstream.Write(buffer, 0, buffer.Length);
outstream.Flush();
}
}
//Create message dialog box.
MessageDialog msgDialog = new("Do you want to view the document?", "File has been created successfully");
UICommand yesCmd = new("Yes");
msgDialog.Commands.Add(yesCmd);
UICommand noCmd = new("No");
msgDialog.Commands.Add(noCmd);
WinRT.Interop.InitializeWithWindow.Initialize(msgDialog, windowHandle);
//Showing a dialog box.
IUICommand cmd = await msgDialog.ShowAsync();
if (cmd.Label == yesCmd.Label)
{
//Launch the saved file.
await Windows.System.Launcher.LaunchFileAsync(stFile);
}
}
}
}
}
Imports Windows.Storage
Imports Windows.Storage.Pickers
Imports Windows.Storage.Streams
Imports Windows.UI.Popups
Namespace MAUI_IronXL
Partial Public Class SaveService
Public Async Sub SaveAndView(ByVal fileName As String, ByVal contentType As String, ByVal stream As MemoryStream)
Dim stFile As StorageFile
Dim extension As String = Path.GetExtension(fileName)
'Gets process windows handle to open the dialog in application process.
Dim windowHandle As IntPtr = System.Diagnostics.Process.GetCurrentProcess().MainWindowHandle
If Not Windows.Foundation.Metadata.ApiInformation.IsTypePresent("Windows.Phone.UI.Input.HardwareButtons") Then
'Creates file save picker to save a file.
Dim savePicker As New FileSavePicker()
savePicker.DefaultFileExtension = ".xlsx"
savePicker.SuggestedFileName = fileName
'Saves the file as Excel file.
savePicker.FileTypeChoices.Add("XLSX", New List(Of String)() From {".xlsx"})
WinRT.Interop.InitializeWithWindow.Initialize(savePicker, windowHandle)
stFile = Await savePicker.PickSaveFileAsync()
Else
Dim local As StorageFolder = ApplicationData.Current.LocalFolder
stFile = Await local.CreateFileAsync(fileName, CreationCollisionOption.ReplaceExisting)
End If
If stFile IsNot Nothing Then
Using zipStream As IRandomAccessStream = Await stFile.OpenAsync(FileAccessMode.ReadWrite)
'Writes compressed data from memory to file.
Using outstream As Stream = zipStream.AsStreamForWrite()
outstream.SetLength(0)
'Saves the stream as file.
Dim buffer() As Byte = outstream.ToArray()
outstream.Write(buffer, 0, buffer.Length)
outstream.Flush()
End Using
End Using
'Create message dialog box.
Dim msgDialog As New MessageDialog("Do you want to view the document?", "File has been created successfully")
Dim yesCmd As New UICommand("Yes")
msgDialog.Commands.Add(yesCmd)
Dim noCmd As New UICommand("No")
msgDialog.Commands.Add(noCmd)
WinRT.Interop.InitializeWithWindow.Initialize(msgDialog, windowHandle)
'Showing a dialog box.
Dim cmd As IUICommand = Await msgDialog.ShowAsync()
If cmd.Label = yesCmd.Label Then
'Launch the saved file.
Await Windows.System.Launcher.LaunchFileAsync(stFile)
End If
End If
End Sub
End Class
End Namespace
輸出
建立並運行 MAUI 專案。 成功執行後,將打開一個窗口顯示下圖中的內容。
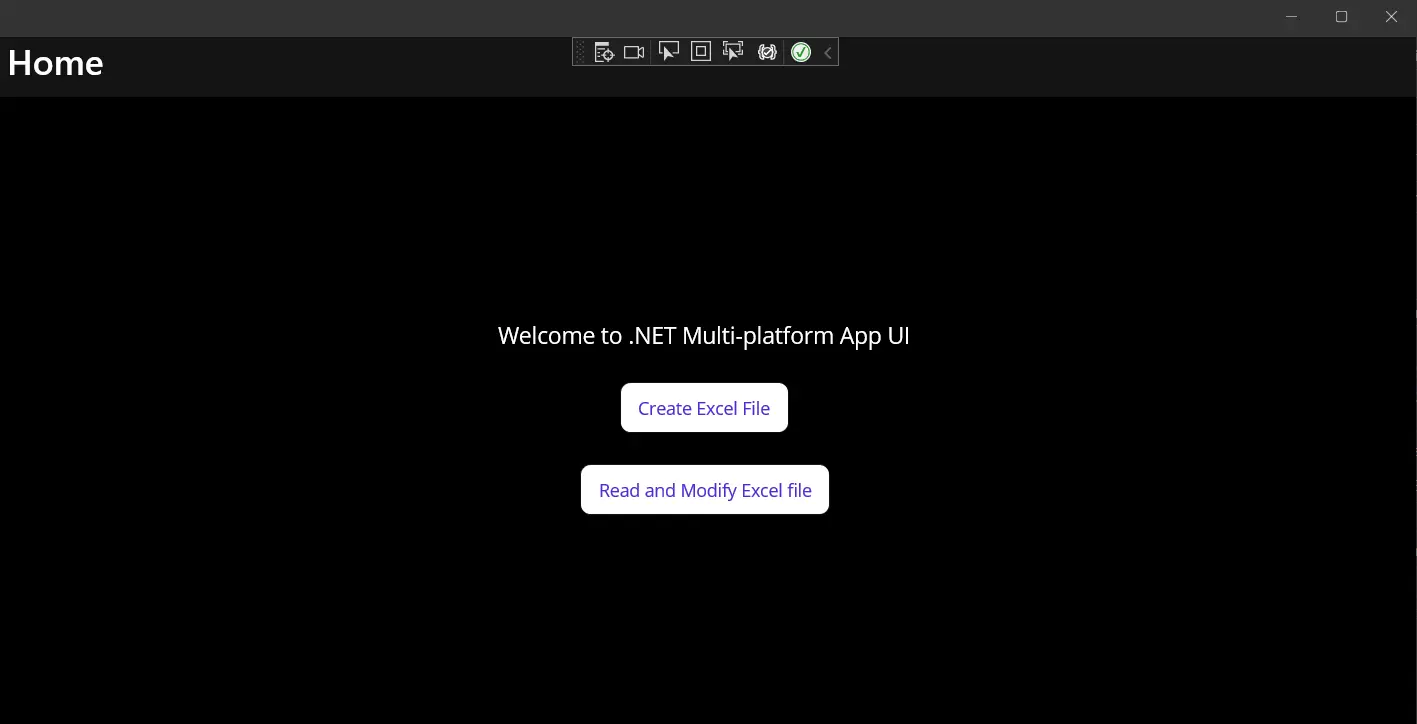
圖 1 - 輸出
按下“建立 Excel 檔案”按鈕將會開啟一個獨立的對話視窗。 此視窗提示使用者選擇一個位置和檔案名稱以保存新的(生成)指定位置和文件名,然後點擊確定。 之後,另一個對話窗口將會出現。
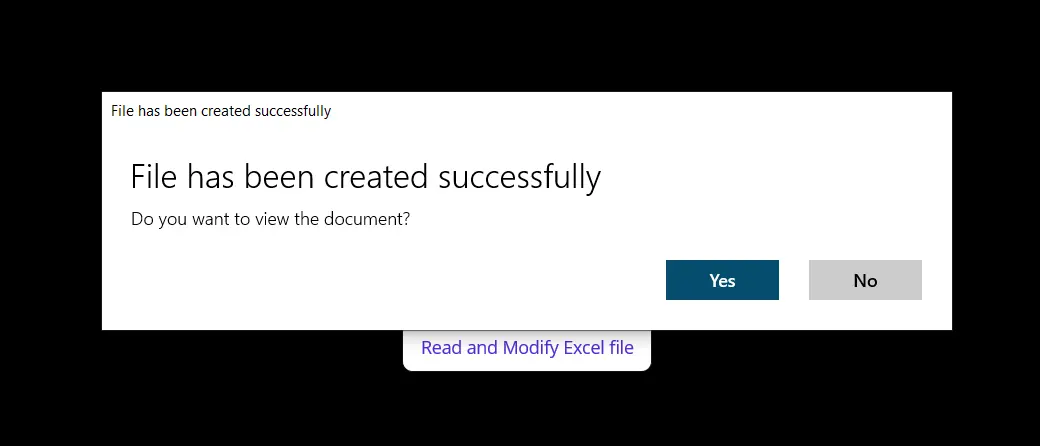
圖2 - 建立 Excel 彈出視窗
按照彈出視窗中的指示打開 Excel 檔案,會顯示如下圖所示的文件。
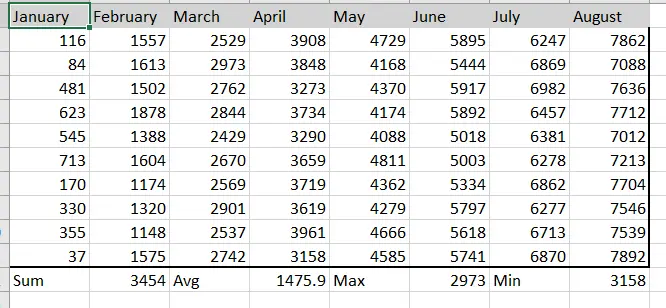
圖3 - 讀取和修改 Excel 彈出視窗
點擊「讀取和修改 Excel 文件」按鈕會加載先前生成的 Excel 文件,並使用我們在前面部分定義的自訂背景和文字顏色進行修改。
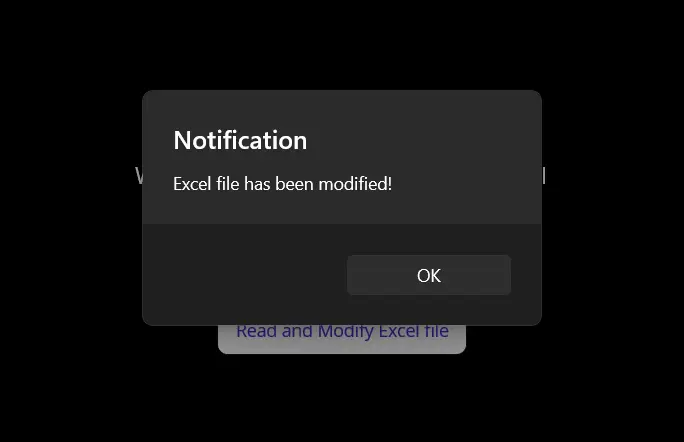
圖4 - Excel 輸出
當您打開修改後的檔案時,您將看到具有目錄的以下輸出。
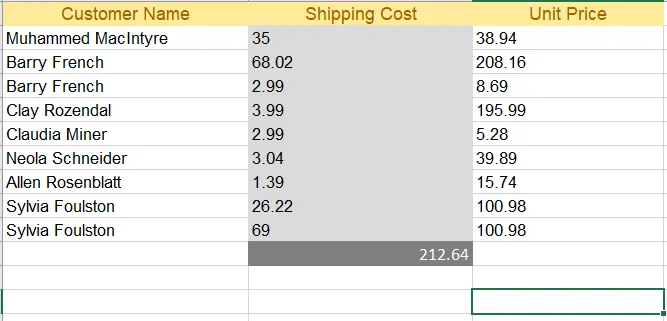
圖 5 - 修改後的 Excel 輸出
結論
這解釋了我們如何使用IronXL庫在.NET MAUI應用程序中創建、讀取和修改Excel文件。 IronXL執行非常出色,並且所有操作都迅速且精確。 IronXL 是一個出色的 Excel 操作庫。 它比Microsoft Interop好得多,因為它不需要在機器上安裝Microsoft Office套件。此外,IronXL支持多種操作,如創建工作簿和工作表、處理單元格範圍、格式化以及導出到多種文件類型,如CSV、TSV等等。
IronXL 支持所有項目模板,如 Windows Form、WPF、ASP.NET Core 等等。 請參考我們的教學課程,建立Excel文件和讀取 Excel 文件欲了解如何使用IronXL的更多資訊。
快速存取連結
在 GitHub 上查看這份操作指南
此項目的原始碼可在 GitHub 上獲得。
使用以下代碼可以輕鬆在幾分鐘內上手。本專案以 Microsoft Visual Studio 2022 專案形式保存,但也兼容任何 .NET IDE。
如何在 .NET MAUI 應用程式中讀取、建立和編輯 Excel 文件