Créer, lire et modifier des fichiers Excel dans .NET MAUI
Introduction
Ce guide pratique explique comment créer et lire des fichiers Excel dans les applications .NET MAUI pour Windows à l'aide d'IronXL. Commençons.
IronXL : C# ; Bibliothèque Excel
IronXL est une bibliothèque C# .NET pour la lecture, l'écriture et la manipulation de fichiers Excel. Il permet aux utilisateurs de créer des documents Excel à partir de zéro, y compris le contenu et l'apparence d'Excel, ainsi que des métadonnées telles que le titre et l'auteur. La bibliothèque prend également en charge les fonctions de personnalisation de l'interface utilisateur, telles que la définition des marges, de l'orientation, de la taille des pages, des images, etc. Il ne nécessite aucun cadre externe, aucune intégration de plate-forme ni aucune autre bibliothèque tierce pour générer des fichiers Excel. Il est autonome et indépendant.
Comment lire des fichiers Excel dans .NET Maui
- Installer la bibliothèque C# pour lire les fichiers Excel
- S'assurer que tous les paquets nécessaires à l'exécution des applications MAUI sont installés
- Créez des fichiers Excel avec des API intuitives dans Maui
- Charger et afficher des fichiers Excel dans le navigateur
- Enregistrer et exporter des fichiers Excel
Installer IronXL
Commencez à utiliser IronXL dans votre projet dès aujourd'hui avec un essai gratuit.
Vous pouvez installer IronXL en utilisant la console NuGet Package Manager dans Visual Studio. Ouvrez la console et entrez la commande suivante pour installer la bibliothèque IronXL.
Install-Package IronXL.Excel
Guide pratique
Création de fichiers Excel en C# à l'aide de IronXL
Conception de l'application frontale
Ouvrez la page XAML nommée **MainPage.xaml**
et remplacez le code par le fragment de code suivant.
<?xml version="1.0" encoding="utf-8" ?>
<ContentPage xmlns="http://schemas.microsoft.com/dotnet/2021/maui"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
x:Class="MAUI_IronXL.MainPage">
<ScrollView>
<VerticalStackLayout
Spacing="25"
Padding="30,0"
VerticalOptions="Center">
<Label
Text="Welcome to .NET Multi-platform App UI"
SemanticProperties.HeadingLevel="Level2"
SemanticProperties.Description="Welcome Multi-platform App UI"
FontSize="18"
HorizontalOptions="Center" />
<Button
x:Name="createBtn"
Text="Create Excel File"
SemanticProperties.Hint="Click on the button to create Excel file"
Clicked="CreateExcel"
HorizontalOptions="Center" />
<Button
x:Name="readExcel"
Text="Read and Modify Excel file"
SemanticProperties.Hint="Click on the button to read Excel file"
Clicked="ReadExcel"
HorizontalOptions="Center" />
</VerticalStackLayout>
</ScrollView>
</ContentPage>
<?xml version="1.0" encoding="utf-8" ?>
<ContentPage xmlns="http://schemas.microsoft.com/dotnet/2021/maui"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
x:Class="MAUI_IronXL.MainPage">
<ScrollView>
<VerticalStackLayout
Spacing="25"
Padding="30,0"
VerticalOptions="Center">
<Label
Text="Welcome to .NET Multi-platform App UI"
SemanticProperties.HeadingLevel="Level2"
SemanticProperties.Description="Welcome Multi-platform App UI"
FontSize="18"
HorizontalOptions="Center" />
<Button
x:Name="createBtn"
Text="Create Excel File"
SemanticProperties.Hint="Click on the button to create Excel file"
Clicked="CreateExcel"
HorizontalOptions="Center" />
<Button
x:Name="readExcel"
Text="Read and Modify Excel file"
SemanticProperties.Hint="Click on the button to read Excel file"
Clicked="ReadExcel"
HorizontalOptions="Center" />
</VerticalStackLayout>
</ScrollView>
</ContentPage>
Le code ci-dessus crée la présentation de notre application MAUI .NET de base. Il crée une étiquette et deux boutons. Un bouton permet de créer un fichier Excel, et le second bouton permet de lire et de modifier le fichier Excel. Les deux éléments sont imbriqués dans un élément parent VerticalStackLayout de sorte qu'ils apparaissent alignés verticalement sur tous les appareils compatibles.
Créer des fichiers Excel
Il est temps de créer le fichier Excel à l'aide d'IronXL. Ouvrez le fichier MainPage.xaml.cs
et écrivez la méthode suivante dans le fichier.
private void CreateExcel(object sender, EventArgs e)
{
//Create Workbook
WorkBook workbook = WorkBook.Create(ExcelFileFormat.XLSX);
//Create Worksheet
var sheet = workbook.CreateWorkSheet("2022 Budget");
//Set Cell values
sheet ["A1"].Value = "January";
sheet ["B1"].Value = "February";
sheet ["C1"].Value = "March";
sheet ["D1"].Value = "April";
sheet ["E1"].Value = "May";
sheet ["F1"].Value = "June";
sheet ["G1"].Value = "July";
sheet ["H1"].Value = "August";
//Set Cell input Dynamically
Random r = new Random();
for (int i = 2; i <= 11; i++)
{
sheet ["A" + i].Value = r.Next(1, 1000);
sheet ["B" + i].Value = r.Next(1000, 2000);
sheet ["C" + i].Value = r.Next(2000, 3000);
sheet ["D" + i].Value = r.Next(3000, 4000);
sheet ["E" + i].Value = r.Next(4000, 5000);
sheet ["F" + i].Value = r.Next(5000, 6000);
sheet ["G" + i].Value = r.Next(6000, 7000);
sheet ["H" + i].Value = r.Next(7000, 8000);
}
//Apply formatting like background and border
sheet ["A1:H1"].Style.SetBackgroundColor("#d3d3d3");
sheet ["A1:H1"].Style.TopBorder.SetColor("#000000");
sheet ["A1:H1"].Style.BottomBorder.SetColor("#000000");
sheet ["H2:H11"].Style.RightBorder.SetColor("#000000");
sheet ["H2:H11"].Style.RightBorder.Type = IronXL.Styles.BorderType.Medium;
sheet ["A11:H11"].Style.BottomBorder.SetColor("#000000");
sheet ["A11:H11"].Style.BottomBorder.Type = IronXL.Styles.BorderType.Medium;
//Apply Formulas
decimal sum = sheet ["A2:A11"].Sum();
decimal avg = sheet ["B2:B11"].Avg();
decimal max = sheet ["C2:C11"].Max();
decimal min = sheet ["D2:D11"].Min();
sheet ["A12"].Value = "Sum";
sheet ["B12"].Value = sum;
sheet ["C12"].Value = "Avg";
sheet ["D12"].Value = avg;
sheet ["E12"].Value = "Max";
sheet ["F12"].Value = max;
sheet ["G12"].Value = "Min";
sheet ["H12"].Value = min;
//Save and Open Excel File
SaveService saveService = new SaveService();
saveService.SaveAndView("Budget.xlsx", "application/octet-stream", workbook.ToStream());
}
private void CreateExcel(object sender, EventArgs e)
{
//Create Workbook
WorkBook workbook = WorkBook.Create(ExcelFileFormat.XLSX);
//Create Worksheet
var sheet = workbook.CreateWorkSheet("2022 Budget");
//Set Cell values
sheet ["A1"].Value = "January";
sheet ["B1"].Value = "February";
sheet ["C1"].Value = "March";
sheet ["D1"].Value = "April";
sheet ["E1"].Value = "May";
sheet ["F1"].Value = "June";
sheet ["G1"].Value = "July";
sheet ["H1"].Value = "August";
//Set Cell input Dynamically
Random r = new Random();
for (int i = 2; i <= 11; i++)
{
sheet ["A" + i].Value = r.Next(1, 1000);
sheet ["B" + i].Value = r.Next(1000, 2000);
sheet ["C" + i].Value = r.Next(2000, 3000);
sheet ["D" + i].Value = r.Next(3000, 4000);
sheet ["E" + i].Value = r.Next(4000, 5000);
sheet ["F" + i].Value = r.Next(5000, 6000);
sheet ["G" + i].Value = r.Next(6000, 7000);
sheet ["H" + i].Value = r.Next(7000, 8000);
}
//Apply formatting like background and border
sheet ["A1:H1"].Style.SetBackgroundColor("#d3d3d3");
sheet ["A1:H1"].Style.TopBorder.SetColor("#000000");
sheet ["A1:H1"].Style.BottomBorder.SetColor("#000000");
sheet ["H2:H11"].Style.RightBorder.SetColor("#000000");
sheet ["H2:H11"].Style.RightBorder.Type = IronXL.Styles.BorderType.Medium;
sheet ["A11:H11"].Style.BottomBorder.SetColor("#000000");
sheet ["A11:H11"].Style.BottomBorder.Type = IronXL.Styles.BorderType.Medium;
//Apply Formulas
decimal sum = sheet ["A2:A11"].Sum();
decimal avg = sheet ["B2:B11"].Avg();
decimal max = sheet ["C2:C11"].Max();
decimal min = sheet ["D2:D11"].Min();
sheet ["A12"].Value = "Sum";
sheet ["B12"].Value = sum;
sheet ["C12"].Value = "Avg";
sheet ["D12"].Value = avg;
sheet ["E12"].Value = "Max";
sheet ["F12"].Value = max;
sheet ["G12"].Value = "Min";
sheet ["H12"].Value = min;
//Save and Open Excel File
SaveService saveService = new SaveService();
saveService.SaveAndView("Budget.xlsx", "application/octet-stream", workbook.ToStream());
}
Private Sub CreateExcel(ByVal sender As Object, ByVal e As EventArgs)
'Create Workbook
Dim workbook As WorkBook = WorkBook.Create(ExcelFileFormat.XLSX)
'Create Worksheet
Dim sheet = workbook.CreateWorkSheet("2022 Budget")
'Set Cell values
sheet ("A1").Value = "January"
sheet ("B1").Value = "February"
sheet ("C1").Value = "March"
sheet ("D1").Value = "April"
sheet ("E1").Value = "May"
sheet ("F1").Value = "June"
sheet ("G1").Value = "July"
sheet ("H1").Value = "August"
'Set Cell input Dynamically
Dim r As New Random()
For i As Integer = 2 To 11
sheet ("A" & i).Value = r.Next(1, 1000)
sheet ("B" & i).Value = r.Next(1000, 2000)
sheet ("C" & i).Value = r.Next(2000, 3000)
sheet ("D" & i).Value = r.Next(3000, 4000)
sheet ("E" & i).Value = r.Next(4000, 5000)
sheet ("F" & i).Value = r.Next(5000, 6000)
sheet ("G" & i).Value = r.Next(6000, 7000)
sheet ("H" & i).Value = r.Next(7000, 8000)
Next i
'Apply formatting like background and border
sheet ("A1:H1").Style.SetBackgroundColor("#d3d3d3")
sheet ("A1:H1").Style.TopBorder.SetColor("#000000")
sheet ("A1:H1").Style.BottomBorder.SetColor("#000000")
sheet ("H2:H11").Style.RightBorder.SetColor("#000000")
sheet ("H2:H11").Style.RightBorder.Type = IronXL.Styles.BorderType.Medium
sheet ("A11:H11").Style.BottomBorder.SetColor("#000000")
sheet ("A11:H11").Style.BottomBorder.Type = IronXL.Styles.BorderType.Medium
'Apply Formulas
Dim sum As Decimal = sheet ("A2:A11").Sum()
Dim avg As Decimal = sheet ("B2:B11").Avg()
Dim max As Decimal = sheet ("C2:C11").Max()
Dim min As Decimal = sheet ("D2:D11").Min()
sheet ("A12").Value = "Sum"
sheet ("B12").Value = sum
sheet ("C12").Value = "Avg"
sheet ("D12").Value = avg
sheet ("E12").Value = "Max"
sheet ("F12").Value = max
sheet ("G12").Value = "Min"
sheet ("H12").Value = min
'Save and Open Excel File
Dim saveService As New SaveService()
saveService.SaveAndView("Budget.xlsx", "application/octet-stream", workbook.ToStream())
End Sub
Le code source crée un classeur contenant une feuille de calcul à l'aide d'IronXL, puis définit les valeurs des cellules à l'aide de la propriété "Value" pour chaque cellule.
La propriété style nous permet d'ajouter un style et des bordures aux cellules. Comme indiqué ci-dessus, nous pouvons appliquer des styles à une seule cellule ou à une série de cellules.
IronXL prend également en charge les formules Excel. Les formules Excel personnalisées peuvent être créées dans une ou plusieurs cellules. En outre, les valeurs résultant d'une formule Excel peuvent être stockées dans une variable en vue d'une utilisation ultérieure.
La classe SaveService
sera utilisée pour sauvegarder et visualiser les fichiers Excel générés. Cette classe est déclarée dans le code ci-dessus et sera définie formellement dans une section ultérieure.
Visualiser les fichiers Excel dans le navigateur
Ouvrez le fichier MainPage.xaml.cs
et écrivez le code suivant.
private void ReadExcel(object sender, EventArgs e)
{
//store the path of a file
string filepath="C:\Files\Customer Data.xlsx";
WorkBook workbook = WorkBook.Load(filepath);
WorkSheet sheet = workbook.WorkSheets.First();
decimal sum = sheet ["B2:B10"].Sum();
sheet ["B11"].Value = sum;
sheet ["B11"].Style.SetBackgroundColor("#808080");
sheet ["B11"].Style.Font.SetColor("#ffffff");
//Save and Open Excel File
SaveService saveService = new SaveService();
saveService.SaveAndView("Modified Data.xlsx", "application/octet-stream", workbook.ToStream());
DisplayAlert("Notification", "Excel file has been modified!", "OK");
}
private void ReadExcel(object sender, EventArgs e)
{
//store the path of a file
string filepath="C:\Files\Customer Data.xlsx";
WorkBook workbook = WorkBook.Load(filepath);
WorkSheet sheet = workbook.WorkSheets.First();
decimal sum = sheet ["B2:B10"].Sum();
sheet ["B11"].Value = sum;
sheet ["B11"].Style.SetBackgroundColor("#808080");
sheet ["B11"].Style.Font.SetColor("#ffffff");
//Save and Open Excel File
SaveService saveService = new SaveService();
saveService.SaveAndView("Modified Data.xlsx", "application/octet-stream", workbook.ToStream());
DisplayAlert("Notification", "Excel file has been modified!", "OK");
}
Private Sub ReadExcel(ByVal sender As Object, ByVal e As EventArgs)
'store the path of a file
Dim filepath As String="C:\Files\Customer Data.xlsx"
Dim workbook As WorkBook = WorkBook.Load(filepath)
Dim sheet As WorkSheet = workbook.WorkSheets.First()
Dim sum As Decimal = sheet ("B2:B10").Sum()
sheet ("B11").Value = sum
sheet ("B11").Style.SetBackgroundColor("#808080")
sheet ("B11").Style.Font.SetColor("#ffffff")
'Save and Open Excel File
Dim saveService As New SaveService()
saveService.SaveAndView("Modified Data.xlsx", "application/octet-stream", workbook.ToStream())
DisplayAlert("Notification", "Excel file has been modified!", "OK")
End Sub
Le code source charge le fichier Excel, applique la formule à une plage de cellules et la met en forme avec des couleurs d'arrière-plan et de texte personnalisées. Ensuite, le fichier Excel est transféré au navigateur de l'utilisateur sous la forme d'un flux d'octets. De plus, la méthode DisplayAlert
présente un message indiquant que le fichier a été ouvert et modifié.
Enregistrer les fichiers Excel
Dans cette section, nous allons maintenant définir la classe SaveService
que nous avons référencée dans les deux sections précédentes et qui enregistrera nos fichiers Excel dans le stockage local.
Créez une classe "SaveService.cs" et écrivez le code suivant :
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace MAUI_IronXL
{
public partial class SaveService
{
public partial void SaveAndView(string fileName, string contentType, MemoryStream stream);
}
}
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace MAUI_IronXL
{
public partial class SaveService
{
public partial void SaveAndView(string fileName, string contentType, MemoryStream stream);
}
}
Imports System
Imports System.Collections.Generic
Imports System.Linq
Imports System.Text
Imports System.Threading.Tasks
Namespace MAUI_IronXL
Partial Public Class SaveService
Public Partial Private Sub SaveAndView(ByVal fileName As String, ByVal contentType As String, ByVal stream As MemoryStream)
End Sub
End Class
End Namespace
Ensuite, créez une classe nommée "SaveWindows.cs" dans le dossier Platforms > Windows, et ajoutez-y le code ci-dessous.
using Windows.Storage;
using Windows.Storage.Pickers;
using Windows.Storage.Streams;
using Windows.UI.Popups;
namespace MAUI_IronXL
{
public partial class SaveService
{
public async partial void SaveAndView(string fileName, string contentType, MemoryStream stream)
{
StorageFile stFile;
string extension = Path.GetExtension(fileName);
//Gets process windows handle to open the dialog in application process.
IntPtr windowHandle = System.Diagnostics.Process.GetCurrentProcess().MainWindowHandle;
if (!Windows.Foundation.Metadata.ApiInformation.IsTypePresent("Windows.Phone.UI.Input.HardwareButtons"))
{
//Creates file save picker to save a file.
FileSavePicker savePicker = new FileSavePicker();
savePicker.DefaultFileExtension = ".xlsx";
savePicker.SuggestedFileName = fileName;
//Saves the file as Excel file.
savePicker.FileTypeChoices.Add("XLSX", new List<string>() { ".xlsx" });
WinRT.Interop.InitializeWithWindow.Initialize(savePicker, windowHandle);
stFile = await savePicker.PickSaveFileAsync();
}
else
{
StorageFolder local = ApplicationData.Current.LocalFolder;
stFile = await local.CreateFileAsync(fileName, CreationCollisionOption.ReplaceExisting);
}
if (stFile != null)
{
using (IRandomAccessStream zipStream = await stFile.OpenAsync(FileAccessMode.ReadWrite))
{
//Writes compressed data from memory to file.
using(Stream outstream = zipStream.AsStreamForWrite())
{
outstream.SetLength(0);
//Saves the stream as file.
byte [] buffer = outstream.ToArray();
outstream.Write(buffer, 0, buffer.Length);
outstream.Flush();
}
}
//Create message dialog box.
MessageDialog msgDialog = new("Do you want to view the document?", "File has been created successfully");
UICommand yesCmd = new("Yes");
msgDialog.Commands.Add(yesCmd);
UICommand noCmd = new("No");
msgDialog.Commands.Add(noCmd);
WinRT.Interop.InitializeWithWindow.Initialize(msgDialog, windowHandle);
//Showing a dialog box.
IUICommand cmd = await msgDialog.ShowAsync();
if (cmd.Label == yesCmd.Label)
{
//Launch the saved file.
await Windows.System.Launcher.LaunchFileAsync(stFile);
}
}
}
}
}
using Windows.Storage;
using Windows.Storage.Pickers;
using Windows.Storage.Streams;
using Windows.UI.Popups;
namespace MAUI_IronXL
{
public partial class SaveService
{
public async partial void SaveAndView(string fileName, string contentType, MemoryStream stream)
{
StorageFile stFile;
string extension = Path.GetExtension(fileName);
//Gets process windows handle to open the dialog in application process.
IntPtr windowHandle = System.Diagnostics.Process.GetCurrentProcess().MainWindowHandle;
if (!Windows.Foundation.Metadata.ApiInformation.IsTypePresent("Windows.Phone.UI.Input.HardwareButtons"))
{
//Creates file save picker to save a file.
FileSavePicker savePicker = new FileSavePicker();
savePicker.DefaultFileExtension = ".xlsx";
savePicker.SuggestedFileName = fileName;
//Saves the file as Excel file.
savePicker.FileTypeChoices.Add("XLSX", new List<string>() { ".xlsx" });
WinRT.Interop.InitializeWithWindow.Initialize(savePicker, windowHandle);
stFile = await savePicker.PickSaveFileAsync();
}
else
{
StorageFolder local = ApplicationData.Current.LocalFolder;
stFile = await local.CreateFileAsync(fileName, CreationCollisionOption.ReplaceExisting);
}
if (stFile != null)
{
using (IRandomAccessStream zipStream = await stFile.OpenAsync(FileAccessMode.ReadWrite))
{
//Writes compressed data from memory to file.
using(Stream outstream = zipStream.AsStreamForWrite())
{
outstream.SetLength(0);
//Saves the stream as file.
byte [] buffer = outstream.ToArray();
outstream.Write(buffer, 0, buffer.Length);
outstream.Flush();
}
}
//Create message dialog box.
MessageDialog msgDialog = new("Do you want to view the document?", "File has been created successfully");
UICommand yesCmd = new("Yes");
msgDialog.Commands.Add(yesCmd);
UICommand noCmd = new("No");
msgDialog.Commands.Add(noCmd);
WinRT.Interop.InitializeWithWindow.Initialize(msgDialog, windowHandle);
//Showing a dialog box.
IUICommand cmd = await msgDialog.ShowAsync();
if (cmd.Label == yesCmd.Label)
{
//Launch the saved file.
await Windows.System.Launcher.LaunchFileAsync(stFile);
}
}
}
}
}
Imports Windows.Storage
Imports Windows.Storage.Pickers
Imports Windows.Storage.Streams
Imports Windows.UI.Popups
Namespace MAUI_IronXL
Partial Public Class SaveService
Public Async Sub SaveAndView(ByVal fileName As String, ByVal contentType As String, ByVal stream As MemoryStream)
Dim stFile As StorageFile
Dim extension As String = Path.GetExtension(fileName)
'Gets process windows handle to open the dialog in application process.
Dim windowHandle As IntPtr = System.Diagnostics.Process.GetCurrentProcess().MainWindowHandle
If Not Windows.Foundation.Metadata.ApiInformation.IsTypePresent("Windows.Phone.UI.Input.HardwareButtons") Then
'Creates file save picker to save a file.
Dim savePicker As New FileSavePicker()
savePicker.DefaultFileExtension = ".xlsx"
savePicker.SuggestedFileName = fileName
'Saves the file as Excel file.
savePicker.FileTypeChoices.Add("XLSX", New List(Of String)() From {".xlsx"})
WinRT.Interop.InitializeWithWindow.Initialize(savePicker, windowHandle)
stFile = Await savePicker.PickSaveFileAsync()
Else
Dim local As StorageFolder = ApplicationData.Current.LocalFolder
stFile = Await local.CreateFileAsync(fileName, CreationCollisionOption.ReplaceExisting)
End If
If stFile IsNot Nothing Then
Using zipStream As IRandomAccessStream = Await stFile.OpenAsync(FileAccessMode.ReadWrite)
'Writes compressed data from memory to file.
Using outstream As Stream = zipStream.AsStreamForWrite()
outstream.SetLength(0)
'Saves the stream as file.
Dim buffer() As Byte = outstream.ToArray()
outstream.Write(buffer, 0, buffer.Length)
outstream.Flush()
End Using
End Using
'Create message dialog box.
Dim msgDialog As New MessageDialog("Do you want to view the document?", "File has been created successfully")
Dim yesCmd As New UICommand("Yes")
msgDialog.Commands.Add(yesCmd)
Dim noCmd As New UICommand("No")
msgDialog.Commands.Add(noCmd)
WinRT.Interop.InitializeWithWindow.Initialize(msgDialog, windowHandle)
'Showing a dialog box.
Dim cmd As IUICommand = Await msgDialog.ShowAsync()
If cmd.Label = yesCmd.Label Then
'Launch the saved file.
Await Windows.System.Launcher.LaunchFileAsync(stFile)
End If
End If
End Sub
End Class
End Namespace
Sortie
Construire et exécuter le projet MAUI. Si l'opération est réussie, une fenêtre s'ouvrira, affichant le contenu décrit dans l'image ci-dessous.
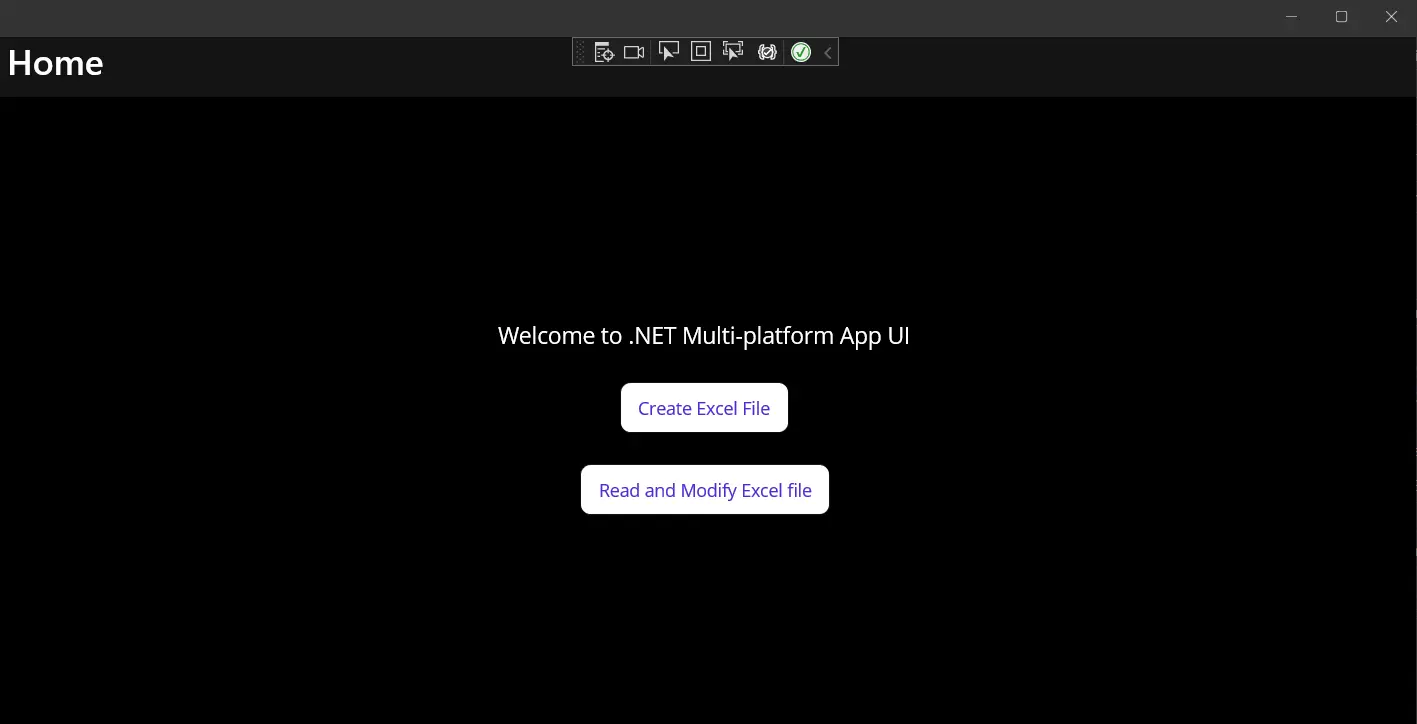
Figure 1 - Sortie
En cliquant sur le bouton "Créer un fichier Excel", une autre fenêtre de dialogue s'ouvre. Cette fenêtre invite les utilisateurs à choisir un emplacement et un nom de fichier pour enregistrer un nouveau fichier Excel généré. Spécifiez l'emplacement et le nom de fichier comme indiqué, puis cliquez sur OK. Une autre fenêtre de dialogue apparaît ensuite.
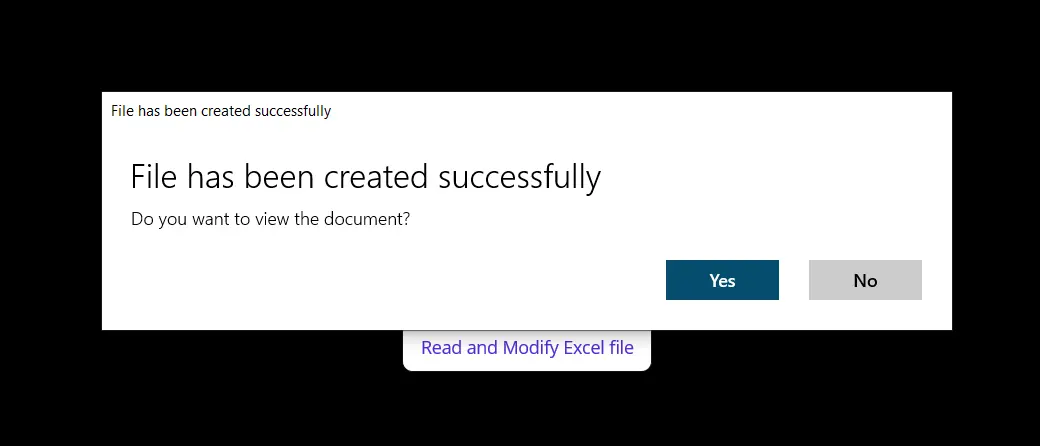
Figure 2 - Créer une fenêtre contextuelle Excel
En ouvrant le fichier Excel comme indiqué dans la fenêtre contextuelle, vous obtiendrez un document comme indiqué dans la capture d'écran ci-dessous.
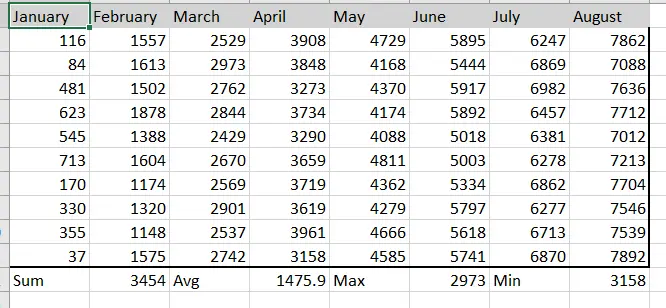
Figure 3 - Lire et Modifier la Fenêtre Contextuelle Excel
En cliquant sur le bouton "Lire et modifier le fichier Excel", le fichier Excel généré précédemment sera chargé et modifié avec les couleurs d'arrière-plan et de texte personnalisées que nous avons définies dans une section précédente.
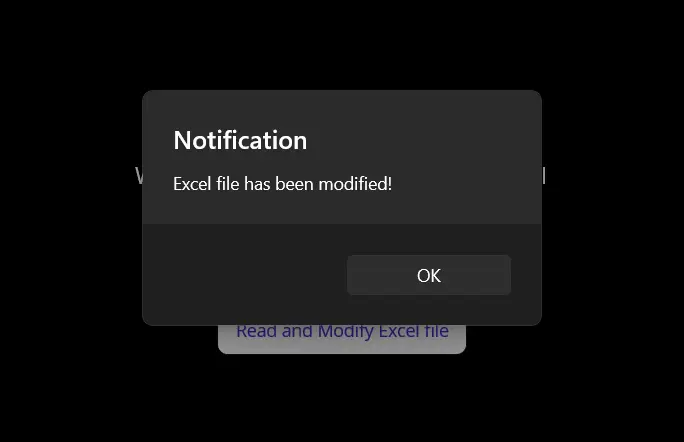
Figure 4 - Sortie Excel
Lorsque vous ouvrez le fichier modifié, vous obtenez le résultat suivant avec la table des matières.
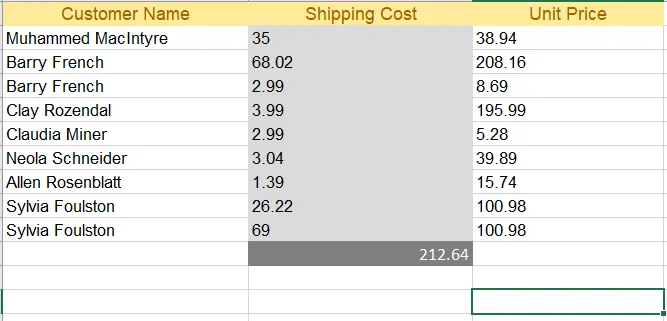
Figure 5 - Sortie Excel Modifiée
Conclusion
Nous avons expliqué comment créer, lire et modifier les fichiers Excel dans l'application .NET MAUI à l'aide de la bibliothèque IronXL. IronXL est très performant et effectue toutes les opérations avec rapidité et précision. IronXL est une excellente bibliothèque pour les opérations sur Excel. Il est bien meilleur que Microsoft Interop car il ne nécessite aucune installation de la suite Microsoft Office sur la machine. En outre, IronXL prend en charge de nombreuses opérations telles que la création de classeurs et de feuilles de calcul, le travail avec des plages de cellules, le formatage et l'exportation vers différents types de documents tels que CSV, TSV, et bien d'autres encore.
IronXL prend en charge tous les modèles de projet tels que Windows Form, WPF, ASP.NET Core et bien d'autres. Consultez nos didacticiels pour lire des fichiers Excel pour des informations supplémentaires sur l'utilisation de IronXL.
Liens d'accès rapide
Explorer ce guide pratique sur GitHub
Le code source de ce projet est disponible sur GitHub.
Utilisez ce code comme un moyen facile d'être opérationnel en quelques minutes. Le projet est enregistré en tant que projet Microsoft Visual Studio 2022, mais il est compatible avec n'importe quel IDE .NET.
Comment lire, créer et modifier des fichiers Excel dans des applications .NET MAUIVoir la référence de l'API
Explorez la référence API d'IronXL, qui décrit en détail toutes les fonctionnalités, les espaces de noms, les classes, les méthodes, les champs et les énums d'IronXL.
Voir la référence de l'API