在C#中读取条码
IronBarcode提供了一個多功能、先進且高效的庫,用於在.NET中讀取條碼。
如何在C#和VB.NET中讀取條碼
- 從NuGet或者通過DLL下載安裝IronBarcode
- 使用
BarcodeReader.Read
讀取任何條碼或 QR 的方法 - 在單次掃描中、PDF 或多幀 Tiff 文件中讀取多個條碼或 QR 碼
- 允許 IronBarcode 從不完美的掃描和照片中讀取
- 下載教程專案,立即開始掃描
安裝
立即在您的專案中使用IronBarcode,並享受免費試用。
IronBarcode提供了一個多功能、先進且高效的庫,用於在.NET中讀取條碼。
第一步是安裝 IronBarcode,這可以通過我們的 NuGet 套件最輕鬆地完成,儘管您也可以選擇手動安裝該 DLL 到您的項目或全域程序集快取。 IronBarcode 很適合用來製作 C# 條碼掃描器應用程式。
Install-Package BarCode
讀取您的第一個條碼
在 .NET 中使用 IronBarcode 類庫和 .NET Barcode Reader 來讀取條碼或 QR Code 非常簡單。 在我們的第一個例子中,我們可以看到如何用一行程式碼讀取這個條碼。
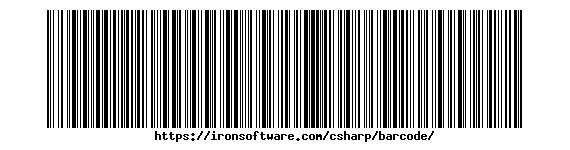
我們可以提取其值、其影像、其編碼類型、其二進位數據(如果有),然後我們可以將其輸出到控制台。
:path=/static-assets/barcode/content-code-examples/tutorials/reading-barcodes-1.cs
using IronBarCode;
using System;
// Read barcode
BarcodeResults results = BarcodeReader.Read("GetStarted.png");
// Log the result to Console Window
foreach (BarcodeResult result in results)
{
if (result != null)
{
Console.WriteLine("GetStarted was a success. Read Value: " + result.Text);
}
}
Imports IronBarCode
Imports System
' Read barcode
Private results As BarcodeResults = BarcodeReader.Read("GetStarted.png")
' Log the result to Console Window
For Each result As BarcodeResult In results
If result IsNot Nothing Then
Console.WriteLine("GetStarted was a success. Read Value: " & result.Text)
End If
Next result
努力更專注和具體化
在下一個範例中,我們將添加條碼掃描選項來讀取一個具挑戰性的圖像。 Speed 枚舉的 ExtremeDetail 值允許對可能被遮擋、損壞或成斜角的條碼進行更深入的掃描。
:path=/static-assets/barcode/content-code-examples/tutorials/reading-barcodes-2.cs
using IronBarCode;
BarcodeReaderOptions options = new BarcodeReaderOptions()
{
// Choose a speed from: Faster, Balanced, Detailed, ExtremeDetail
// There is a tradeoff in performance as more Detail is set
Speed = ReadingSpeed.ExtremeDetail,
// By default, all barcode formats are scanned for.
// Specifying one or more, performance will increase.
ExpectBarcodeTypes = BarcodeEncoding.QRCode | BarcodeEncoding.Code128,
};
// Read barcode
BarcodeResults results = BarcodeReader.Read("TryHarderQR.png", options);
Imports IronBarCode
Private options As New BarcodeReaderOptions() With {
.Speed = ReadingSpeed.ExtremeDetail,
.ExpectBarcodeTypes = BarcodeEncoding.QRCode Or BarcodeEncoding.Code128
}
' Read barcode
Private results As BarcodeResults = BarcodeReader.Read("TryHarderQR.png", options)
這將讀取這個傾斜的 QR 碼:
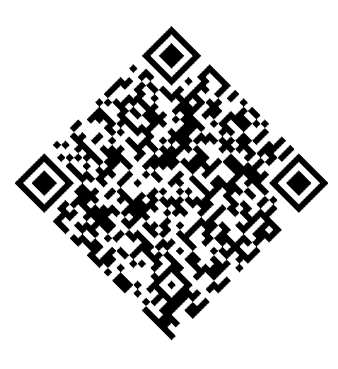
在我們的示例中,您可以看到我們可以指定條碼編碼(s)我們正在尋找甚至多種格式。 這樣做可以大幅提高條碼讀取的性能和準確性。 這 pipe character, or 'Bitwise OR,' is used to specify multiple formats simultaneously.
如果我們前進並使用 ImageFilters 和 AutoRotate 屬性,同樣的效果可以達到,但具有更高的特異性。
:path=/static-assets/barcode/content-code-examples/tutorials/reading-barcodes-3.cs
using IronBarCode;
BarcodeReaderOptions options = new BarcodeReaderOptions()
{
// Choose which filters are to be applied (in order)
ImageFilters = new ImageFilterCollection() {
new AdaptiveThresholdFilter(),
},
// Uses machine learning to auto rotate the barcode
AutoRotate = true,
};
// Read barcode
BarcodeResults results = BarcodeReader.Read("TryHarderQR.png", options);
Imports IronBarCode
Private options As New BarcodeReaderOptions() With {
.ImageFilters = New ImageFilterCollection() From {New AdaptiveThresholdFilter()},
.AutoRotate = True
}
' Read barcode
Private results As BarcodeResults = BarcodeReader.Read("TryHarderQR.png", options)
讀取多個條碼
PDF 文件
在下一個範例中,我們將探討如何讀取一個掃描的PDF文件並以極少的代碼行數找到所有一維格式的條碼。
如您所見,這與從單個文件中讀取單個條碼非常相似,只是我們現在有了關於找到條碼所在頁碼的新信息。
:path=/static-assets/barcode/content-code-examples/tutorials/reading-barcodes-4.cs
using IronBarCode;
using System;
// Multiple barcodes may be scanned up from a single document or image. A PDF document may also used as the input image
BarcodeResults results = BarcodeReader.ReadPdf("MultipleBarcodes.pdf");
// Work with the results
foreach (var pageResult in results)
{
string Value = pageResult.Value;
int PageNum = pageResult.PageNumber;
System.Drawing.Bitmap Img = pageResult.BarcodeImage;
BarcodeEncoding BarcodeType = pageResult.BarcodeType;
byte[] Binary = pageResult.BinaryValue;
Console.WriteLine(pageResult.Value + " on page " + PageNum);
}
Imports IronBarCode
Imports System
' Multiple barcodes may be scanned up from a single document or image. A PDF document may also used as the input image
Private results As BarcodeResults = BarcodeReader.ReadPdf("MultipleBarcodes.pdf")
' Work with the results
For Each pageResult In results
Dim Value As String = pageResult.Value
Dim PageNum As Integer = pageResult.PageNumber
Dim Img As System.Drawing.Bitmap = pageResult.BarcodeImage
Dim BarcodeType As BarcodeEncoding = pageResult.BarcodeType
Dim Binary() As Byte = pageResult.BinaryValue
Console.WriteLine(pageResult.Value & " on page " & PageNum)
Next pageResult
我們在不同的頁面上發現以下條碼。
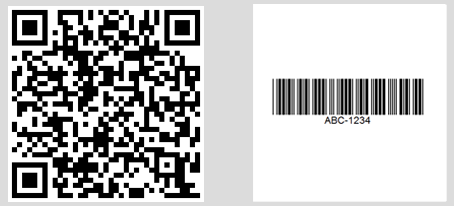
掃描 TIFFs
在我們的下一個例子中,我們可以看出,可以從多幀TIFF中獲得相同的結果,在此情境下這將類似於PDF處理。
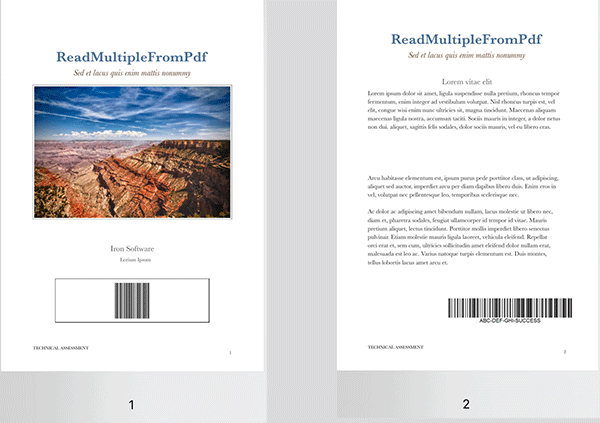
:path=/static-assets/barcode/content-code-examples/tutorials/reading-barcodes-5.cs
using IronBarCode;
// Multi frame TIFF and GIF images can also be scanned
BarcodeResults multiFrameResults = BarcodeReader.Read("Multiframe.tiff");
foreach (var pageResult in multiFrameResults)
{
//...
}
Imports IronBarCode
' Multi frame TIFF and GIF images can also be scanned
Private multiFrameResults As BarcodeResults = BarcodeReader.Read("Multiframe.tiff")
For Each pageResult In multiFrameResults
'...
Next pageResult
多執行緒
要讀取多個文件,我們可以通過創建文件列表並使用 IronBarcode 來獲得更好的結果。 BarcodeReader.Read
方法。 這利用多個執行緒並潛在地使用您 CPU 的所有核心進行條碼掃描過程,並且可能比一次讀取一個條碼快得多。
:path=/static-assets/barcode/content-code-examples/tutorials/reading-barcodes-6.cs
using IronBarCode;
// The Multithreaded property allows for faster barcode scanning across multiple images or PDFs. All threads are automatically managed by IronBarCode.
var ListOfDocuments = new[] { "image1.png", "image2.JPG", "image3.pdf" };
BarcodeReaderOptions options = new BarcodeReaderOptions()
{
// Enable multithreading
Multithreaded = true,
};
BarcodeResults batchResults = BarcodeReader.Read(ListOfDocuments, options);
Imports IronBarCode
' The Multithreaded property allows for faster barcode scanning across multiple images or PDFs. All threads are automatically managed by IronBarCode.
Private ListOfDocuments = { "image1.png", "image2.JPG", "image3.pdf" }
Private options As New BarcodeReaderOptions() With {.Multithreaded = True}
Private batchResults As BarcodeResults = BarcodeReader.Read(ListOfDocuments, options)
摘要
總而言之,IronBarcode 是一個多功能的 .NET 軟體庫和 C# 二維碼生成器,能夠讀取多種條碼格式。 無論條碼是完美的螢幕截圖,還是不完美的現實世界圖像,例如照片或掃描,它都可以做到。
進一步閱讀
若想了解更多有關使用 IronBarcode 的資訊,您可以探索本部分中的其他教程,以及我們首頁上的示例,大多數開發人員會發現這些內容足以讓他們開始使用。
我们的API 參考文獻,特別是 BarcodeReader 類和 BarcodeEncoding 枚舉,將提供有關您可以使用此 C# 條碼庫 達成的詳細資訊。
源代碼下載
我們也強烈建議您下載此教程並自行運行。 您可以透過下載源代碼或在 GitHub 上來分支我們來實現這一點。 此 .NET 條碼閱讀器教學的原始碼可作為使用 C# 編寫的 Visual Studio 2017 控制台應用程式專案使用。