How to Scrape a Blog in C#
Let’s use Iron WebScraper to extract Blog content using C# or VB.NET.
This tutorial shows how a WordPress blog (or similar) may be scraped back into content using .NET
// Define a class that extends WebScraper from IronWebScraper
public class BlogScraper : WebScraper
{
/// <summary>
/// Override this method to initialize your web-scraper.
/// Set at least one start URL and configure domain or URL patterns.
/// </summary>
public override void Init()
{
// Set your license key for IronWebScraper
License.LicenseKey = "YourLicenseKey";
// Enable logging for all actions
this.LoggingLevel = WebScraper.LogLevel.All;
// Set a directory to store output and cache files
this.WorkingDirectory = AppSetting.GetAppRoot() + @"\BlogSample\Output\";
// Enable caching with a specific duration
EnableWebCache(new TimeSpan(1, 30, 30));
// Request the start URL and specify the response handler
this.Request("http://blogSite.com/", Parse);
}
}
// Define a class that extends WebScraper from IronWebScraper
public class BlogScraper : WebScraper
{
/// <summary>
/// Override this method to initialize your web-scraper.
/// Set at least one start URL and configure domain or URL patterns.
/// </summary>
public override void Init()
{
// Set your license key for IronWebScraper
License.LicenseKey = "YourLicenseKey";
// Enable logging for all actions
this.LoggingLevel = WebScraper.LogLevel.All;
// Set a directory to store output and cache files
this.WorkingDirectory = AppSetting.GetAppRoot() + @"\BlogSample\Output\";
// Enable caching with a specific duration
EnableWebCache(new TimeSpan(1, 30, 30));
// Request the start URL and specify the response handler
this.Request("http://blogSite.com/", Parse);
}
}
' Define a class that extends WebScraper from IronWebScraper
Public Class BlogScraper
Inherits WebScraper
''' <summary>
''' Override this method to initialize your web-scraper.
''' Set at least one start URL and configure domain or URL patterns.
''' </summary>
Public Overrides Sub Init()
' Set your license key for IronWebScraper
License.LicenseKey = "YourLicenseKey"
' Enable logging for all actions
Me.LoggingLevel = WebScraper.LogLevel.All
' Set a directory to store output and cache files
Me.WorkingDirectory = AppSetting.GetAppRoot() & "\BlogSample\Output\"
' Enable caching with a specific duration
EnableWebCache(New TimeSpan(1, 30, 30))
' Request the start URL and specify the response handler
Me.Request("http://blogSite.com/", Parse)
End Sub
End Class
As usual, we create a Scraper and inherit from the WebScraper class. In this case, it is "BlogScraper".
We set a working directory to “\BlogSample\Output\” where all of our output and cache files can go.
Then we enable the web cache to save requested pages inside the cache folder “WebCache.”
Now let’s write a parse function:
/// <summary>
/// Override this method to handle the Http Response for your web scraper.
/// Add additional methods if you handle multiple page types.
/// </summary>
/// <param name="response">The HTTP Response object to parse.</param>
public override void Parse(Response response)
{
// Iterate over each link found in the section navigation
foreach (var link in response.Css("div.section-nav > ul > li > a"))
{
switch(link.TextContentClean)
{
case "Reviews":
{
// Handle reviews case
}
break;
case "Science":
{
// Handle science case
}
break;
default:
{
// Save the link title to a file
Scrape(new ScrapedData() { { "Title", link.TextContentClean } }, "BlogScraper.Jsonl");
}
break;
}
}
}
/// <summary>
/// Override this method to handle the Http Response for your web scraper.
/// Add additional methods if you handle multiple page types.
/// </summary>
/// <param name="response">The HTTP Response object to parse.</param>
public override void Parse(Response response)
{
// Iterate over each link found in the section navigation
foreach (var link in response.Css("div.section-nav > ul > li > a"))
{
switch(link.TextContentClean)
{
case "Reviews":
{
// Handle reviews case
}
break;
case "Science":
{
// Handle science case
}
break;
default:
{
// Save the link title to a file
Scrape(new ScrapedData() { { "Title", link.TextContentClean } }, "BlogScraper.Jsonl");
}
break;
}
}
}
''' <summary>
''' Override this method to handle the Http Response for your web scraper.
''' Add additional methods if you handle multiple page types.
''' </summary>
''' <param name="response">The HTTP Response object to parse.</param>
Public Overrides Sub Parse(ByVal response As Response)
' Iterate over each link found in the section navigation
For Each link In response.Css("div.section-nav > ul > li > a")
Select Case link.TextContentClean
Case "Reviews"
' Handle reviews case
Case "Science"
' Handle science case
Case Else
' Save the link title to a file
Scrape(New ScrapedData() From {
{ "Title", link.TextContentClean }
},
"BlogScraper.Jsonl")
End Select
Next link
End Sub
Inside the Parse method, we get all the links to category pages (Movies, Science, Reviews, etc.) from the top menu.
We then switch to a suitable parse method based on the link category.
Let's prepare our object model for the Science Page:
/// <summary>
/// Represents a model for Science Page
/// </summary>
public class ScienceModel
{
/// <summary>
/// Gets or sets the title.
/// </summary>
public string Title { get; set; }
/// <summary>
/// Gets or sets the author.
/// </summary>
public string Author { get; set; }
/// <summary>
/// Gets or sets the date.
/// </summary>
public string Date { get; set; }
/// <summary>
/// Gets or sets the image.
/// </summary>
public string Image { get; set; }
/// <summary>
/// Gets or sets the text.
/// </summary>
public string Text { get; set; }
}
/// <summary>
/// Represents a model for Science Page
/// </summary>
public class ScienceModel
{
/// <summary>
/// Gets or sets the title.
/// </summary>
public string Title { get; set; }
/// <summary>
/// Gets or sets the author.
/// </summary>
public string Author { get; set; }
/// <summary>
/// Gets or sets the date.
/// </summary>
public string Date { get; set; }
/// <summary>
/// Gets or sets the image.
/// </summary>
public string Image { get; set; }
/// <summary>
/// Gets or sets the text.
/// </summary>
public string Text { get; set; }
}
''' <summary>
''' Represents a model for Science Page
''' </summary>
Public Class ScienceModel
''' <summary>
''' Gets or sets the title.
''' </summary>
Public Property Title() As String
''' <summary>
''' Gets or sets the author.
''' </summary>
Public Property Author() As String
''' <summary>
''' Gets or sets the date.
''' </summary>
Public Property [Date]() As String
''' <summary>
''' Gets or sets the image.
''' </summary>
Public Property Image() As String
''' <summary>
''' Gets or sets the text.
''' </summary>
Public Property Text() As String
End Class
Now let’s implement a single page scrape:
/// <summary>
/// Parses the reviews from the response.
/// </summary>
/// <param name="response">The HTTP Response object.</param>
public void ParseReviews(Response response)
{
// A list to hold Science models
var scienceList = new List<ScienceModel>();
foreach (var postBox in response.Css("section.main > div > div.post-list"))
{
var item = new ScienceModel
{
Title = postBox.Css("h1.headline > a")[0].TextContentClean,
Author = postBox.Css("div.author > a")[0].TextContentClean,
Date = postBox.Css("div.time > a")[0].TextContentClean,
Image = postBox.Css("div.image-wrapper.default-state > img")[0].Attributes["src"],
Text = postBox.Css("div.summary > p")[0].TextContentClean
};
scienceList.Add(item);
}
// Save the science list to a JSONL file
Scrape(scienceList, "BlogScience.Jsonl");
}
/// <summary>
/// Parses the reviews from the response.
/// </summary>
/// <param name="response">The HTTP Response object.</param>
public void ParseReviews(Response response)
{
// A list to hold Science models
var scienceList = new List<ScienceModel>();
foreach (var postBox in response.Css("section.main > div > div.post-list"))
{
var item = new ScienceModel
{
Title = postBox.Css("h1.headline > a")[0].TextContentClean,
Author = postBox.Css("div.author > a")[0].TextContentClean,
Date = postBox.Css("div.time > a")[0].TextContentClean,
Image = postBox.Css("div.image-wrapper.default-state > img")[0].Attributes["src"],
Text = postBox.Css("div.summary > p")[0].TextContentClean
};
scienceList.Add(item);
}
// Save the science list to a JSONL file
Scrape(scienceList, "BlogScience.Jsonl");
}
''' <summary>
''' Parses the reviews from the response.
''' </summary>
''' <param name="response">The HTTP Response object.</param>
Public Sub ParseReviews(ByVal response As Response)
' A list to hold Science models
Dim scienceList = New List(Of ScienceModel)()
For Each postBox In response.Css("section.main > div > div.post-list")
Dim item = New ScienceModel With {
.Title = postBox.Css("h1.headline > a")(0).TextContentClean,
.Author = postBox.Css("div.author > a")(0).TextContentClean,
.Date = postBox.Css("div.time > a")(0).TextContentClean,
.Image = postBox.Css("div.image-wrapper.default-state > img")(0).Attributes("src"),
.Text = postBox.Css("div.summary > p")(0).TextContentClean
}
scienceList.Add(item)
Next postBox
' Save the science list to a JSONL file
Scrape(scienceList, "BlogScience.Jsonl")
End Sub
After we have created our model, we can parse the response object to drill down into its main elements (title, author, date, image, text).
Then, we save our results in a separate file using Scrape(object, fileName)
.
Click here for the full tutorial on the use of IronWebscraper
Get started with IronWebscraper
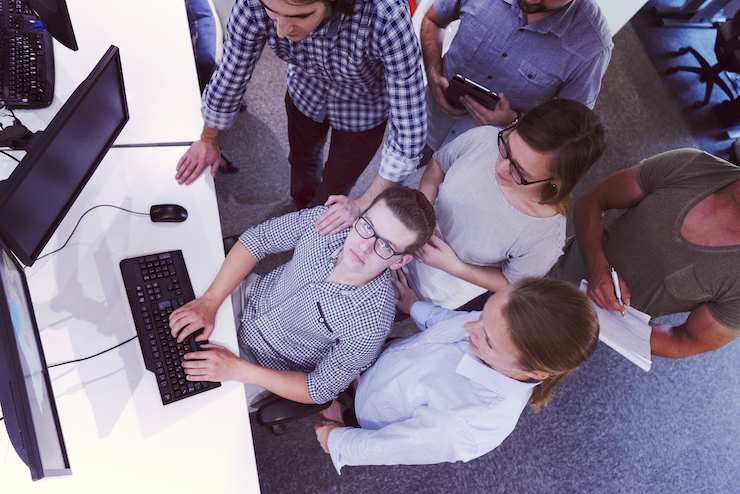
Frequently Asked Questions
What is this web scraping framework?
IronWebScraper is a web scraping framework designed to extract and process data from web pages using C# or VB.NET.
How do I start a blog web scraper?
To start a blog web scraper using IronWebScraper, create a class that extends the WebScraper class, set your start URL, configure domain or URL patterns, and define how the HTTP responses should be parsed.
What is the purpose of setting a working directory?
Setting a working directory in IronWebScraper allows you to specify where output and cache files should be stored, facilitating organized data management.
How can I handle multiple page types?
You can handle multiple page types in IronWebScraper by overriding the Parse method to check for different link categories and switch to suitable parsing methods accordingly.
What is the use of the web cache?
The web cache in IronWebScraper is used to save requested pages, which can improve performance by reducing the need to re-fetch the same pages multiple times.
How do you save scraped data?
Scraped data in IronWebScraper can be saved by using the Scrape method, which allows you to specify the data object and the file name for saving.
What is a ScienceModel in this context?
A ScienceModel is a class that represents the data structure for a science page, including properties such as Title, Author, Date, Image, and Text.
How does this framework handle HTTP responses?
IronWebScraper handles HTTP responses by overriding the Parse method, which processes the response object to extract and save desired data.
Can I use this framework with VB.NET?
Yes, IronWebScraper can be used with both C# and VB.NET, making it versatile for developers familiar with either language.
Where can I find the full tutorial on using this framework?
The full tutorial on using IronWebScraper can be found on the IronSoftware website, under the web scraping tutorials section.