在 .NET MAUI 中创建、读取和编辑 Excel 文件
介绍
本操作指南解释了如何使用IronXL在Windows的.NET MAUI应用程序中创建和读取Excel文件。让我们开始吧。
IronXL:C# Excel 库
IronXL 是一个用于读写和操作 Excel 文件的 C# .NET 库。它允许用户从头开始创建 Excel 文档,包括 Excel 的内容和外观,以及标题和作者等元数据。该库还支持用户界面定制功能,如设置页边距、方向、页面大小、图像等。生成 Excel 文件不需要任何外部框架、平台集成或其他第三方库。它自成一体,可独立运行。
如何在 .NET Maui 中读取 Excel 文件
- 安装 C# 库读取 Excel 文件
- 确保已安装运行 MAUI 应用程序所需的所有软件包
- 在 Maui 中使用直观的 API 创建 Excel 文件
- 在浏览器中加载和查看 Excel 文件
- 保存和导出 Excel 文件
安装 IronXL
开始在您的项目中使用IronPDF,并立即获取免费试用。
查看 IronXL 上 Nuget 用于快速安装和部署。它有超过800万次下载,正在使用C#改变Excel。
考虑安装 IronXL DLL 直接。下载并手动安装到您的项目或GAC表单中: IronXL.zip
您可以使用Visual Studio中的NuGet包管理控制台安装IronXL。打开控制台并输入以下命令以安装IronXL库。
Install-Package IronXL.Excel
使用指南
使用 IronXL 在 C# 中创建 Excel 文件
设计应用程序前端
打开名为 **MainPage.xaml**
的 XAML 页面,用以下代码段替换其中的代码。
<?xml version="1.0" encoding="utf-8" ?>
<ContentPage xmlns="http://schemas.microsoft.com/dotnet/2021/maui"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
x:Class="MAUI_IronXL.MainPage">
<ScrollView>
<VerticalStackLayout
Spacing="25"
Padding="30,0"
VerticalOptions="Center">
<Label
Text="Welcome to .NET Multi-platform App UI"
SemanticProperties.HeadingLevel="Level2"
SemanticProperties.Description="Welcome Multi-platform App UI"
FontSize="18"
HorizontalOptions="Center" />
<Button
x:Name="createBtn"
Text="Create Excel File"
SemanticProperties.Hint="Click on the button to create Excel file"
Clicked="CreateExcel"
HorizontalOptions="Center" />
<Button
x:Name="readExcel"
Text="Read and Modify Excel file"
SemanticProperties.Hint="Click on the button to read Excel file"
Clicked="ReadExcel"
HorizontalOptions="Center" />
</VerticalStackLayout>
</ScrollView>
</ContentPage>
上面的代码为我们的基本 .NET MAUI 应用程序创建了布局。它创建了一个标签和两个按钮。一个按钮用于创建 Excel 文件,第二个按钮支持读取和修改 Excel 文件。这两个元素都嵌套在一个 VerticalStackLayout 父元素中,因此它们在所有支持的设备上都是垂直对齐的。
创建 Excel 文件
是时候使用 IronXL 创建 Excel 文件了。打开 MainPage.xaml.cs
文件并在文件中编写以下方法。
private void CreateExcel(object sender, EventArgs e)
{
//Create Workbook
WorkBook workbook = WorkBook.Create(ExcelFileFormat.XLSX);
//Create Worksheet
var sheet = workbook.CreateWorkSheet("2022 Budget");
//Set Cell values
sheet ["A1"].Value = "January";
sheet ["B1"].Value = "February";
sheet ["C1"].Value = "March";
sheet ["D1"].Value = "April";
sheet ["E1"].Value = "May";
sheet ["F1"].Value = "June";
sheet ["G1"].Value = "July";
sheet ["H1"].Value = "August";
//Set Cell input Dynamically
Random r = new Random();
for (int i = 2; i <= 11; i++)
{
sheet ["A" + i].Value = r.Next(1, 1000);
sheet ["B" + i].Value = r.Next(1000, 2000);
sheet ["C" + i].Value = r.Next(2000, 3000);
sheet ["D" + i].Value = r.Next(3000, 4000);
sheet ["E" + i].Value = r.Next(4000, 5000);
sheet ["F" + i].Value = r.Next(5000, 6000);
sheet ["G" + i].Value = r.Next(6000, 7000);
sheet ["H" + i].Value = r.Next(7000, 8000);
}
//Apply formatting like background and border
sheet ["A1:H1"].Style.SetBackgroundColor("#d3d3d3");
sheet ["A1:H1"].Style.TopBorder.SetColor("#000000");
sheet ["A1:H1"].Style.BottomBorder.SetColor("#000000");
sheet ["H2:H11"].Style.RightBorder.SetColor("#000000");
sheet ["H2:H11"].Style.RightBorder.Type = IronXL.Styles.BorderType.Medium;
sheet ["A11:H11"].Style.BottomBorder.SetColor("#000000");
sheet ["A11:H11"].Style.BottomBorder.Type = IronXL.Styles.BorderType.Medium;
//Apply Formulas
decimal sum = sheet ["A2:A11"].Sum();
decimal avg = sheet ["B2:B11"].Avg();
decimal max = sheet ["C2:C11"].Max();
decimal min = sheet ["D2:D11"].Min();
sheet ["A12"].Value = "Sum";
sheet ["B12"].Value = sum;
sheet ["C12"].Value = "Avg";
sheet ["D12"].Value = avg;
sheet ["E12"].Value = "Max";
sheet ["F12"].Value = max;
sheet ["G12"].Value = "Min";
sheet ["H12"].Value = min;
//Save and Open Excel File
SaveService saveService = new SaveService();
saveService.SaveAndView("Budget.xlsx", "application/octet-stream", workbook.ToStream());
}
private void CreateExcel(object sender, EventArgs e)
{
//Create Workbook
WorkBook workbook = WorkBook.Create(ExcelFileFormat.XLSX);
//Create Worksheet
var sheet = workbook.CreateWorkSheet("2022 Budget");
//Set Cell values
sheet ["A1"].Value = "January";
sheet ["B1"].Value = "February";
sheet ["C1"].Value = "March";
sheet ["D1"].Value = "April";
sheet ["E1"].Value = "May";
sheet ["F1"].Value = "June";
sheet ["G1"].Value = "July";
sheet ["H1"].Value = "August";
//Set Cell input Dynamically
Random r = new Random();
for (int i = 2; i <= 11; i++)
{
sheet ["A" + i].Value = r.Next(1, 1000);
sheet ["B" + i].Value = r.Next(1000, 2000);
sheet ["C" + i].Value = r.Next(2000, 3000);
sheet ["D" + i].Value = r.Next(3000, 4000);
sheet ["E" + i].Value = r.Next(4000, 5000);
sheet ["F" + i].Value = r.Next(5000, 6000);
sheet ["G" + i].Value = r.Next(6000, 7000);
sheet ["H" + i].Value = r.Next(7000, 8000);
}
//Apply formatting like background and border
sheet ["A1:H1"].Style.SetBackgroundColor("#d3d3d3");
sheet ["A1:H1"].Style.TopBorder.SetColor("#000000");
sheet ["A1:H1"].Style.BottomBorder.SetColor("#000000");
sheet ["H2:H11"].Style.RightBorder.SetColor("#000000");
sheet ["H2:H11"].Style.RightBorder.Type = IronXL.Styles.BorderType.Medium;
sheet ["A11:H11"].Style.BottomBorder.SetColor("#000000");
sheet ["A11:H11"].Style.BottomBorder.Type = IronXL.Styles.BorderType.Medium;
//Apply Formulas
decimal sum = sheet ["A2:A11"].Sum();
decimal avg = sheet ["B2:B11"].Avg();
decimal max = sheet ["C2:C11"].Max();
decimal min = sheet ["D2:D11"].Min();
sheet ["A12"].Value = "Sum";
sheet ["B12"].Value = sum;
sheet ["C12"].Value = "Avg";
sheet ["D12"].Value = avg;
sheet ["E12"].Value = "Max";
sheet ["F12"].Value = max;
sheet ["G12"].Value = "Min";
sheet ["H12"].Value = min;
//Save and Open Excel File
SaveService saveService = new SaveService();
saveService.SaveAndView("Budget.xlsx", "application/octet-stream", workbook.ToStream());
}
Private Sub CreateExcel(ByVal sender As Object, ByVal e As EventArgs)
'Create Workbook
Dim workbook As WorkBook = WorkBook.Create(ExcelFileFormat.XLSX)
'Create Worksheet
Dim sheet = workbook.CreateWorkSheet("2022 Budget")
'Set Cell values
sheet ("A1").Value = "January"
sheet ("B1").Value = "February"
sheet ("C1").Value = "March"
sheet ("D1").Value = "April"
sheet ("E1").Value = "May"
sheet ("F1").Value = "June"
sheet ("G1").Value = "July"
sheet ("H1").Value = "August"
'Set Cell input Dynamically
Dim r As New Random()
For i As Integer = 2 To 11
sheet ("A" & i).Value = r.Next(1, 1000)
sheet ("B" & i).Value = r.Next(1000, 2000)
sheet ("C" & i).Value = r.Next(2000, 3000)
sheet ("D" & i).Value = r.Next(3000, 4000)
sheet ("E" & i).Value = r.Next(4000, 5000)
sheet ("F" & i).Value = r.Next(5000, 6000)
sheet ("G" & i).Value = r.Next(6000, 7000)
sheet ("H" & i).Value = r.Next(7000, 8000)
Next i
'Apply formatting like background and border
sheet ("A1:H1").Style.SetBackgroundColor("#d3d3d3")
sheet ("A1:H1").Style.TopBorder.SetColor("#000000")
sheet ("A1:H1").Style.BottomBorder.SetColor("#000000")
sheet ("H2:H11").Style.RightBorder.SetColor("#000000")
sheet ("H2:H11").Style.RightBorder.Type = IronXL.Styles.BorderType.Medium
sheet ("A11:H11").Style.BottomBorder.SetColor("#000000")
sheet ("A11:H11").Style.BottomBorder.Type = IronXL.Styles.BorderType.Medium
'Apply Formulas
Dim sum As Decimal = sheet ("A2:A11").Sum()
Dim avg As Decimal = sheet ("B2:B11").Avg()
Dim max As Decimal = sheet ("C2:C11").Max()
Dim min As Decimal = sheet ("D2:D11").Min()
sheet ("A12").Value = "Sum"
sheet ("B12").Value = sum
sheet ("C12").Value = "Avg"
sheet ("D12").Value = avg
sheet ("E12").Value = "Max"
sheet ("F12").Value = max
sheet ("G12").Value = "Min"
sheet ("H12").Value = min
'Save and Open Excel File
Dim saveService As New SaveService()
saveService.SaveAndView("Budget.xlsx", "application/octet-stream", workbook.ToStream())
End Sub
源代码使用 IronXL 创建了一个包含一个工作表的工作簿,然后使用 "Value "属性为每个单元格设置单元格值。
通过样式属性,我们可以为单元格添加样式和边框。如上图所示,我们可以为单个单元格或单元格区域应用样式。
IronXL 还支持 Excel 公式。自定义 Excel 公式可以精心设计一个或多个单元格。此外,任何 Excel 公式产生的值都可以存储在变量中,以便以后使用。
保存服务 "类将用于保存和查看生成的 Excel 文件。该类已在上面的代码中声明,并将在后面的章节中正式定义。
在浏览器中查看 Excel 文件
打开 MainPage.xaml.cs
文件并编写以下代码。
private void ReadExcel(object sender, EventArgs e)
{
//store the path of a file
string filepath="C:\Files\Customer Data.xlsx";
WorkBook workbook = WorkBook.Load(filepath);
WorkSheet sheet = workbook.WorkSheets.First();
decimal sum = sheet ["B2:B10"].Sum();
sheet ["B11"].Value = sum;
sheet ["B11"].Style.SetBackgroundColor("#808080");
sheet ["B11"].Style.Font.SetColor("#ffffff");
//Save and Open Excel File
SaveService saveService = new SaveService();
saveService.SaveAndView("Modified Data.xlsx", "application/octet-stream", workbook.ToStream());
DisplayAlert("Notification", "Excel file has been modified!", "OK");
}
private void ReadExcel(object sender, EventArgs e)
{
//store the path of a file
string filepath="C:\Files\Customer Data.xlsx";
WorkBook workbook = WorkBook.Load(filepath);
WorkSheet sheet = workbook.WorkSheets.First();
decimal sum = sheet ["B2:B10"].Sum();
sheet ["B11"].Value = sum;
sheet ["B11"].Style.SetBackgroundColor("#808080");
sheet ["B11"].Style.Font.SetColor("#ffffff");
//Save and Open Excel File
SaveService saveService = new SaveService();
saveService.SaveAndView("Modified Data.xlsx", "application/octet-stream", workbook.ToStream());
DisplayAlert("Notification", "Excel file has been modified!", "OK");
}
Private Sub ReadExcel(ByVal sender As Object, ByVal e As EventArgs)
'store the path of a file
Dim filepath As String="C:\Files\Customer Data.xlsx"
Dim workbook As WorkBook = WorkBook.Load(filepath)
Dim sheet As WorkSheet = workbook.WorkSheets.First()
Dim sum As Decimal = sheet ("B2:B10").Sum()
sheet ("B11").Value = sum
sheet ("B11").Style.SetBackgroundColor("#808080")
sheet ("B11").Style.Font.SetColor("#ffffff")
'Save and Open Excel File
Dim saveService As New SaveService()
saveService.SaveAndView("Modified Data.xlsx", "application/octet-stream", workbook.ToStream())
DisplayAlert("Notification", "Excel file has been modified!", "OK")
End Sub
源代码加载 Excel 文件,在单元格范围内应用公式,并使用自定义背景和文本颜色进行格式化。随后,Excel 文件将以字节流的形式传输到用户的浏览器。此外,"DisplayAlert "方法会显示一条信息,说明文件已被打开和修改。
保存 Excel 文件
在本节中,我们将定义在前两节中引用的 SaveService
类,该类将把 Excel 文件保存到本地存储器中。
创建一个 "SaveService.cs "类并编写以下代码:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace MAUI_IronXL
{
public partial class SaveService
{
public partial void SaveAndView(string fileName, string contentType, MemoryStream stream);
}
}
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace MAUI_IronXL
{
public partial class SaveService
{
public partial void SaveAndView(string fileName, string contentType, MemoryStream stream);
}
}
Imports System
Imports System.Collections.Generic
Imports System.Linq
Imports System.Text
Imports System.Threading.Tasks
Namespace MAUI_IronXL
Partial Public Class SaveService
Public Partial Private Sub SaveAndView(ByVal fileName As String, ByVal contentType As String, ByVal stream As MemoryStream)
End Sub
End Class
End Namespace
接下来,在 Platforms > Windows 文件夹中创建一个名为 "SaveWindows.cs "的类,并添加以下代码。
using Windows.Storage;
using Windows.Storage.Pickers;
using Windows.Storage.Streams;
using Windows.UI.Popups;
namespace MAUI_IronXL
{
public partial class SaveService
{
public async partial void SaveAndView(string fileName, string contentType, MemoryStream stream)
{
StorageFile stFile;
string extension = Path.GetExtension(fileName);
//Gets process windows handle to open the dialog in application process.
IntPtr windowHandle = System.Diagnostics.Process.GetCurrentProcess().MainWindowHandle;
if (!Windows.Foundation.Metadata.ApiInformation.IsTypePresent("Windows.Phone.UI.Input.HardwareButtons"))
{
//Creates file save picker to save a file.
FileSavePicker savePicker = new FileSavePicker();
savePicker.DefaultFileExtension = ".xlsx";
savePicker.SuggestedFileName = fileName;
//Saves the file as Excel file.
savePicker.FileTypeChoices.Add("XLSX", new List<string>() { ".xlsx" });
WinRT.Interop.InitializeWithWindow.Initialize(savePicker, windowHandle);
stFile = await savePicker.PickSaveFileAsync();
}
else
{
StorageFolder local = ApplicationData.Current.LocalFolder;
stFile = await local.CreateFileAsync(fileName, CreationCollisionOption.ReplaceExisting);
}
if (stFile != null)
{
using (IRandomAccessStream zipStream = await stFile.OpenAsync(FileAccessMode.ReadWrite))
{
//Writes compressed data from memory to file.
using(Stream outstream = zipStream.AsStreamForWrite())
{
outstream.SetLength(0);
//Saves the stream as file.
byte [] buffer = outstream.ToArray();
outstream.Write(buffer, 0, buffer.Length);
outstream.Flush();
}
}
//Create message dialog box.
MessageDialog msgDialog = new("Do you want to view the document?", "File has been created successfully");
UICommand yesCmd = new("Yes");
msgDialog.Commands.Add(yesCmd);
UICommand noCmd = new("No");
msgDialog.Commands.Add(noCmd);
WinRT.Interop.InitializeWithWindow.Initialize(msgDialog, windowHandle);
//Showing a dialog box.
IUICommand cmd = await msgDialog.ShowAsync();
if (cmd.Label == yesCmd.Label)
{
//Launch the saved file.
await Windows.System.Launcher.LaunchFileAsync(stFile);
}
}
}
}
}
using Windows.Storage;
using Windows.Storage.Pickers;
using Windows.Storage.Streams;
using Windows.UI.Popups;
namespace MAUI_IronXL
{
public partial class SaveService
{
public async partial void SaveAndView(string fileName, string contentType, MemoryStream stream)
{
StorageFile stFile;
string extension = Path.GetExtension(fileName);
//Gets process windows handle to open the dialog in application process.
IntPtr windowHandle = System.Diagnostics.Process.GetCurrentProcess().MainWindowHandle;
if (!Windows.Foundation.Metadata.ApiInformation.IsTypePresent("Windows.Phone.UI.Input.HardwareButtons"))
{
//Creates file save picker to save a file.
FileSavePicker savePicker = new FileSavePicker();
savePicker.DefaultFileExtension = ".xlsx";
savePicker.SuggestedFileName = fileName;
//Saves the file as Excel file.
savePicker.FileTypeChoices.Add("XLSX", new List<string>() { ".xlsx" });
WinRT.Interop.InitializeWithWindow.Initialize(savePicker, windowHandle);
stFile = await savePicker.PickSaveFileAsync();
}
else
{
StorageFolder local = ApplicationData.Current.LocalFolder;
stFile = await local.CreateFileAsync(fileName, CreationCollisionOption.ReplaceExisting);
}
if (stFile != null)
{
using (IRandomAccessStream zipStream = await stFile.OpenAsync(FileAccessMode.ReadWrite))
{
//Writes compressed data from memory to file.
using(Stream outstream = zipStream.AsStreamForWrite())
{
outstream.SetLength(0);
//Saves the stream as file.
byte [] buffer = outstream.ToArray();
outstream.Write(buffer, 0, buffer.Length);
outstream.Flush();
}
}
//Create message dialog box.
MessageDialog msgDialog = new("Do you want to view the document?", "File has been created successfully");
UICommand yesCmd = new("Yes");
msgDialog.Commands.Add(yesCmd);
UICommand noCmd = new("No");
msgDialog.Commands.Add(noCmd);
WinRT.Interop.InitializeWithWindow.Initialize(msgDialog, windowHandle);
//Showing a dialog box.
IUICommand cmd = await msgDialog.ShowAsync();
if (cmd.Label == yesCmd.Label)
{
//Launch the saved file.
await Windows.System.Launcher.LaunchFileAsync(stFile);
}
}
}
}
}
Imports Windows.Storage
Imports Windows.Storage.Pickers
Imports Windows.Storage.Streams
Imports Windows.UI.Popups
Namespace MAUI_IronXL
Partial Public Class SaveService
Public Async Sub SaveAndView(ByVal fileName As String, ByVal contentType As String, ByVal stream As MemoryStream)
Dim stFile As StorageFile
Dim extension As String = Path.GetExtension(fileName)
'Gets process windows handle to open the dialog in application process.
Dim windowHandle As IntPtr = System.Diagnostics.Process.GetCurrentProcess().MainWindowHandle
If Not Windows.Foundation.Metadata.ApiInformation.IsTypePresent("Windows.Phone.UI.Input.HardwareButtons") Then
'Creates file save picker to save a file.
Dim savePicker As New FileSavePicker()
savePicker.DefaultFileExtension = ".xlsx"
savePicker.SuggestedFileName = fileName
'Saves the file as Excel file.
savePicker.FileTypeChoices.Add("XLSX", New List(Of String)() From {".xlsx"})
WinRT.Interop.InitializeWithWindow.Initialize(savePicker, windowHandle)
stFile = Await savePicker.PickSaveFileAsync()
Else
Dim local As StorageFolder = ApplicationData.Current.LocalFolder
stFile = Await local.CreateFileAsync(fileName, CreationCollisionOption.ReplaceExisting)
End If
If stFile IsNot Nothing Then
Using zipStream As IRandomAccessStream = Await stFile.OpenAsync(FileAccessMode.ReadWrite)
'Writes compressed data from memory to file.
Using outstream As Stream = zipStream.AsStreamForWrite()
outstream.SetLength(0)
'Saves the stream as file.
Dim buffer() As Byte = outstream.ToArray()
outstream.Write(buffer, 0, buffer.Length)
outstream.Flush()
End Using
End Using
'Create message dialog box.
Dim msgDialog As New MessageDialog("Do you want to view the document?", "File has been created successfully")
Dim yesCmd As New UICommand("Yes")
msgDialog.Commands.Add(yesCmd)
Dim noCmd As New UICommand("No")
msgDialog.Commands.Add(noCmd)
WinRT.Interop.InitializeWithWindow.Initialize(msgDialog, windowHandle)
'Showing a dialog box.
Dim cmd As IUICommand = Await msgDialog.ShowAsync()
If cmd.Label = yesCmd.Label Then
'Launch the saved file.
Await Windows.System.Launcher.LaunchFileAsync(stFile)
End If
End If
End Sub
End Class
End Namespace
输出
构建并运行 MAUI 项目。成功执行后,将打开一个窗口,显示下图所示内容。
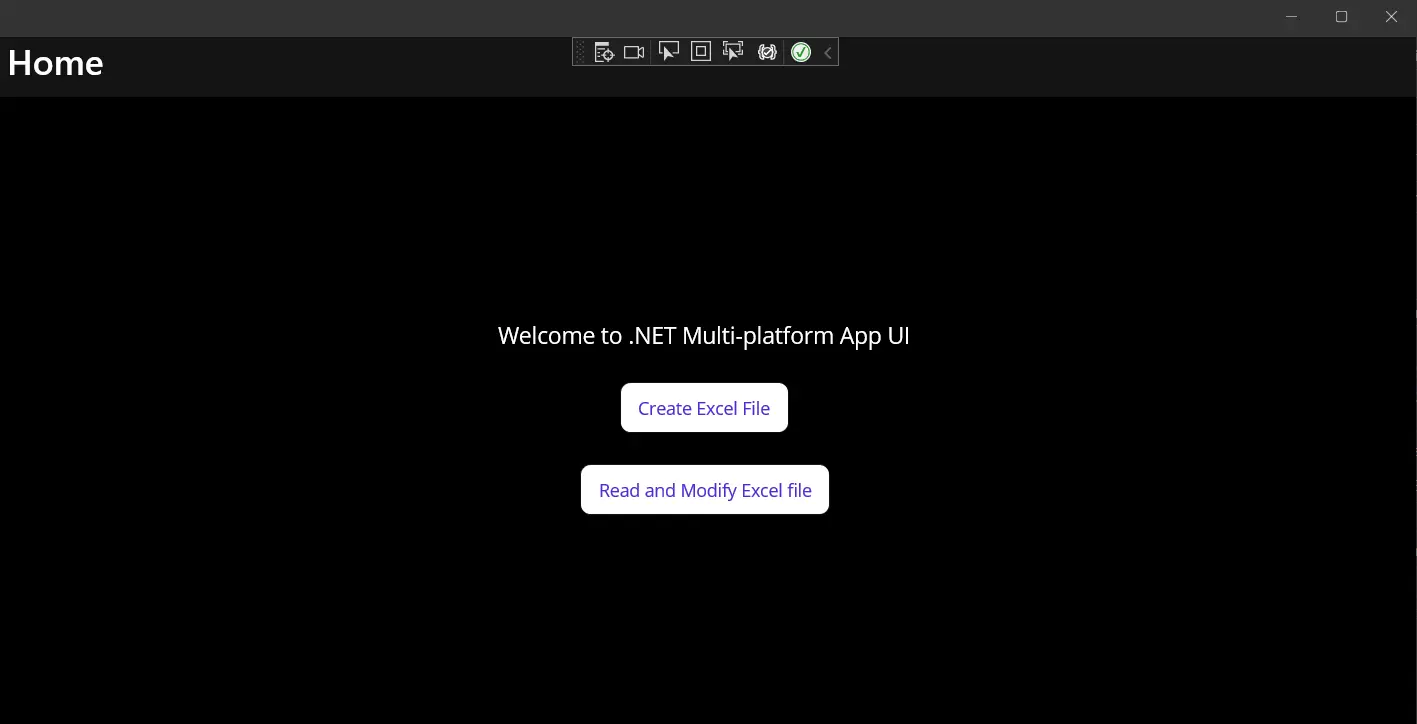
图 1 - 输出
单击 "创建 Excel 文件 "按钮将打开一个单独的对话窗口。该窗口会提示用户选择保存新 Excel 文件的位置和文件名。 (生成的) Excel 文件。按照指示指定位置和文件名,然后单击确定。随后会出现另一个对话窗口。
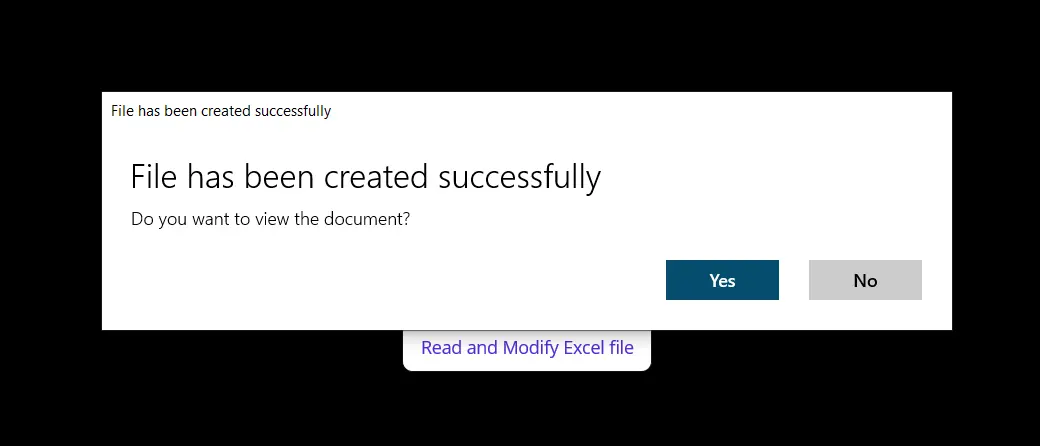
图 2 - 创建 Excel 弹出窗口
按照弹出窗口中的指示打开 Excel 文件,就会出现如下截图所示的文档。
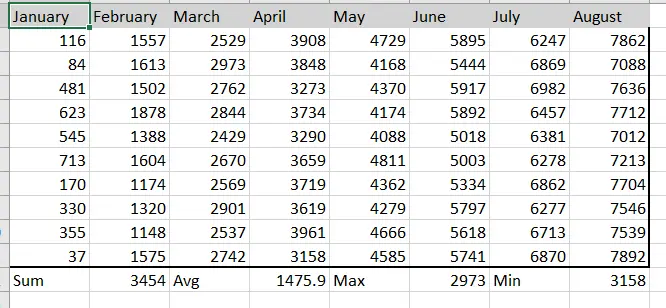
图 3 - 读取和修改 Excel 弹出窗口
单击 "读取并修改 Excel 文件 "按钮将加载先前生成的 Excel 文件,并使用我们在前一节中定义的自定义背景和文本颜色对其进行修改。
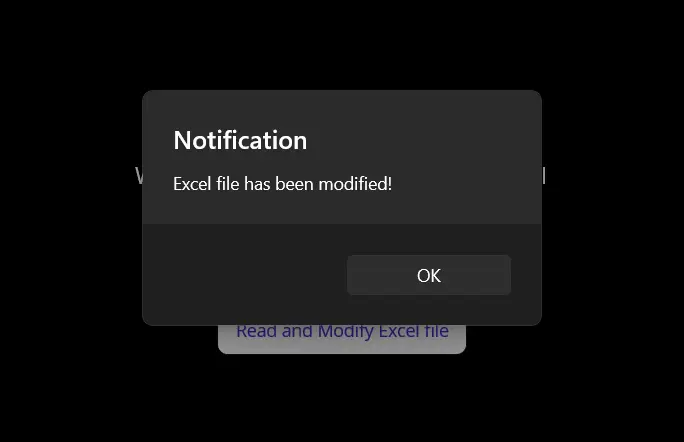
图 4 - Excel 输出
打开修改后的文件,你会看到以下输出结果和目录。
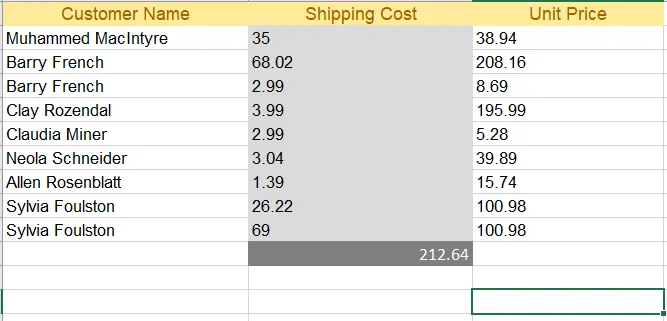
图 5 - 修改后的 Excel 输出
结论
以上介绍了如何使用 IronXL 库在 .NET MAUI 应用程序中创建、读取和修改 Excel 文件。IronXL 性能非常出色,能快速、准确地完成所有操作。IronXL 是 Excel 操作的优秀库。它比 Microsoft Interop 好得多,因为它不需要在机器上安装任何 Microsoft Office 套件。此外,IronXL 还支持多种操作,如创建工作簿和工作表、处理单元格范围、格式化以及导出为 CSV、TSV 等多种文档类型。
IronXL 支持所有项目模板,如 Windows Form、WPF、ASP.NET Core 等。请参考我们的教程 创建 Excel 文件 和 读取 Excel 文件 了解有关如何使用 IronXL 的更多信息。
快速访问链接
在 GitHub 上查看此操作指南
该项目的源代码可在 GitHub 上获取。
使用此代码,只需几分钟就能轻松上手并运行。该项目保存为 Microsoft Visual Studio 2022 项目,但兼容任何 .NET IDE。
如何在 .NET MAUI 应用程序中读取、创建和编辑 Excel 文件