C# QR Code Generator for .NET
如您可能在「創建條碼」入門教程中讀到的,使用IronBarcode創建、設計並導出條碼為圖像非常簡單,往往可以通過一行代碼完成。
在這個例子中,我們將更深入地研究 QR 碼,它們在工業應用和零售業中的普及性逐漸增加。
如何在C#中讀取和生成QR碼
- Download QR code Generator Library
- 只需添加一行代碼即可生成一個QR碼
- 將您的徽標添加到 QR 碼
- 驗證您的 QR 碼是否可讀取
- 處理二進制數據的 QR 代碼讀寫
在C#中安裝QR Code生成器庫
立即在您的專案中使用IronBarcode,並享受免費試用。
在開始之前,我們需要安裝BarCode NuGet 套件。
Install-Package BarCode
https://www.nuget.org/packages/BarCode/ 作為替代方案,可以下載 IronBarCode.Dll並將其作為參考添加到您的專案中,從 [.NET Barcode DLL] 開始。
匯入名稱空間
在本教程中,我們需要確保我們的類文件引用 IronBarcode 以及一些正常的系統組件。
using IronBarCode;
using System;
using System.Drawing;
using System.Linq;
using IronBarCode;
using System;
using System.Drawing;
using System.Linq;
Imports IronBarCode
Imports System
Imports System.Drawing
Imports System.Linq
使用一行代碼創建 QR 碼
我們的第一個例子展示了如何使用一些簡單的文字創建一個標準化的條碼,直徑為500像素的正方形,並具有中等錯誤校正功能。
:path=/static-assets/barcode/content-code-examples/tutorials/csharp-qr-code-generator-1.cs
using IronBarCode;
// Generate a Simple BarCode image and save as PDF
QRCodeWriter.CreateQrCode("hello world", 500, QRCodeWriter.QrErrorCorrectionLevel.Medium).SaveAsPng("MyQR.png");
Imports IronBarCode
' Generate a Simple BarCode image and save as PDF
QRCodeWriter.CreateQrCode("hello world", 500, QRCodeWriter.QrErrorCorrectionLevel.Medium).SaveAsPng("MyQR.png")
錯誤更正允許我們定義在實際情況中讀取 QR 碼的難易程度。 較高的錯誤校正等級會生成更大的 QR 碼,具有更多像素和更高的複雜性。
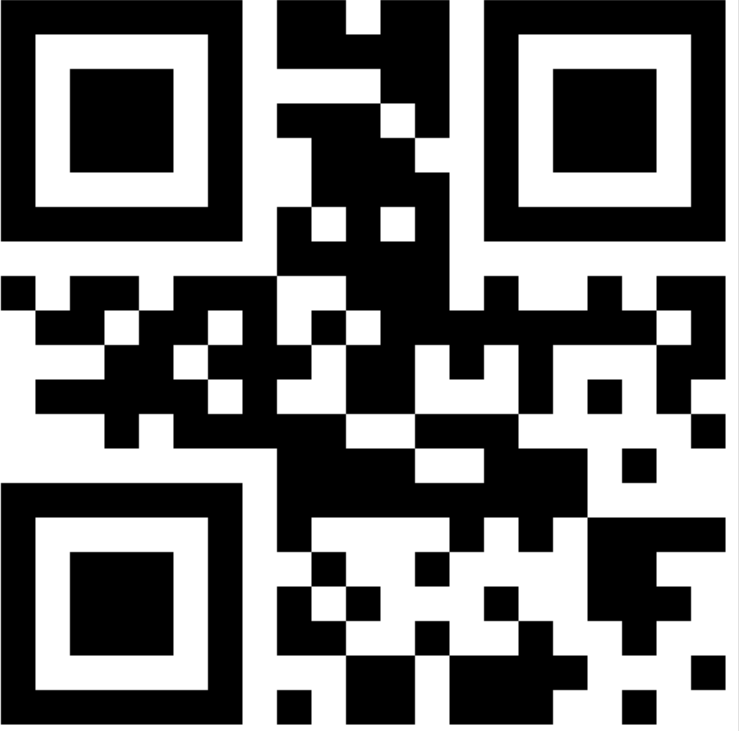
添加標誌
在第二個例子中,我們將研究一個常見的使用案例,那就是一家公司希望在他們的QR碼中添加Logo。 使用 QRCodeWriter.CreateQRCodeWithLogo 方法,在下面的代碼示例中您可以看到這有多簡單。
:path=/static-assets/barcode/content-code-examples/tutorials/csharp-qr-code-generator-2.cs
using IronBarCode;
using IronSoftware.Drawing;
// You may add styling with color, logo images or branding:
QRCodeLogo qrCodeLogo = new QRCodeLogo("visual-studio-logo.png");
GeneratedBarcode myQRCodeWithLogo = QRCodeWriter.CreateQrCodeWithLogo("https://ironsoftware.com/", qrCodeLogo);
myQRCodeWithLogo.ResizeTo(500, 500).SetMargins(10).ChangeBarCodeColor(Color.DarkGreen);
// Logo will automatically be sized appropriately and snapped to the QR grid.
myQRCodeWithLogo.SaveAsPng("myQRWithLogo.png");
Imports IronBarCode
Imports IronSoftware.Drawing
' You may add styling with color, logo images or branding:
Private qrCodeLogo As New QRCodeLogo("visual-studio-logo.png")
Private myQRCodeWithLogo As GeneratedBarcode = QRCodeWriter.CreateQrCodeWithLogo("https://ironsoftware.com/", qrCodeLogo)
myQRCodeWithLogo.ResizeTo(500, 500).SetMargins(10).ChangeBarCodeColor(Color.DarkGreen)
' Logo will automatically be sized appropriately and snapped to the QR grid.
myQRCodeWithLogo.SaveAsPng("myQRWithLogo.png")
此示例將 Visual Studio 標誌添加到條碼中。 它會自動將其調整到適當的大小,以便純代碼仍然可讀,並將該標誌對齊到QR碼方格網格,使其看起來合適。 下一行代碼將條形碼漆成深綠色。 但是,它不會使標誌褪色。
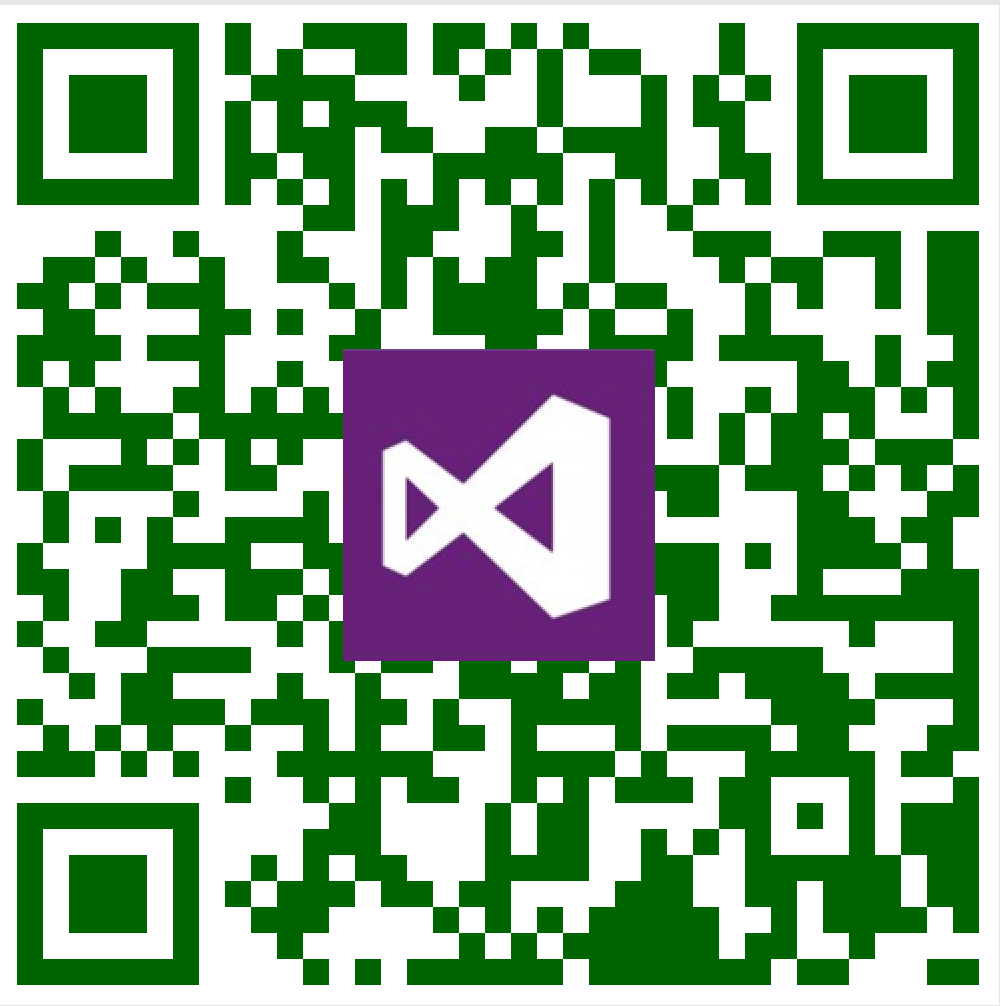
保存為圖像PDF或HTML
最後,我們將該 QR 保存為 PDF。 最後一行代碼將為您的方便在預設的PDF瀏覽器中打開PDF文件。
:path=/static-assets/barcode/content-code-examples/tutorials/csharp-qr-code-generator-3.cs
using IronBarCode;
// You may add styling with color, logo images or branding:
QRCodeLogo qrCodeLogo = new QRCodeLogo("visual-studio-logo.png");
GeneratedBarcode myQRCodeWithLogo = QRCodeWriter.CreateQrCodeWithLogo("https://ironsoftware.com/", qrCodeLogo);
myQRCodeWithLogo.ChangeBarCodeColor(System.Drawing.Color.DarkGreen);
// Save as PDF
myQRCodeWithLogo.SaveAsPdf("MyQRWithLogo.pdf");
// Also Save as HTML
myQRCodeWithLogo.SaveAsHtmlFile("MyQRWithLogo.html");
Imports IronBarCode
' You may add styling with color, logo images or branding:
Private qrCodeLogo As New QRCodeLogo("visual-studio-logo.png")
Private myQRCodeWithLogo As GeneratedBarcode = QRCodeWriter.CreateQrCodeWithLogo("https://ironsoftware.com/", qrCodeLogo)
myQRCodeWithLogo.ChangeBarCodeColor(System.Drawing.Color.DarkGreen)
' Save as PDF
myQRCodeWithLogo.SaveAsPdf("MyQRWithLogo.pdf")
' Also Save as HTML
myQRCodeWithLogo.SaveAsHtmlFile("MyQRWithLogo.html")
驗證 QR 碼
在將標誌添加到 QR 碼並更改顏色時,我們要確保我們的 QR 碼仍然可讀。
通過使用 GeneratedBarcode.Verify()
方法,我們可以測試條碼是否仍然可讀。
在下面的程式碼範例中,我們將看到將 QR 碼改為淺藍色實際上會使其無法讀取。 我們在代碼中檢測到這一點,並且更喜歡深藍色。
:path=/static-assets/barcode/content-code-examples/tutorials/csharp-qr-code-generator-4.cs
using IronBarCode;
using IronSoftware.Drawing;
using System;
// Verifying QR Codes
QRCodeLogo qrCodeLogo = new QRCodeLogo("visual-studio-logo.png");
GeneratedBarcode MyVerifiedQR = QRCodeWriter.CreateQrCodeWithLogo("https://ironsoftware.com/", qrCodeLogo);
MyVerifiedQR.ChangeBarCodeColor(System.Drawing.Color.LightBlue);
if (!MyVerifiedQR.Verify())
{
Console.WriteLine("\t LightBlue is not dark enough to be read accurately. Lets try DarkBlue");
MyVerifiedQR.ChangeBarCodeColor(Color.DarkBlue);
}
MyVerifiedQR.SaveAsHtmlFile("MyVerifiedQR.html");
// open the barcode html file in your default web browser
System.Diagnostics.Process.Start("MyVerifiedQR.html");
Imports Microsoft.VisualBasic
Imports IronBarCode
Imports IronSoftware.Drawing
Imports System
' Verifying QR Codes
Private qrCodeLogo As New QRCodeLogo("visual-studio-logo.png")
Private MyVerifiedQR As GeneratedBarcode = QRCodeWriter.CreateQrCodeWithLogo("https://ironsoftware.com/", qrCodeLogo)
MyVerifiedQR.ChangeBarCodeColor(System.Drawing.Color.LightBlue)
If Not MyVerifiedQR.Verify() Then
Console.WriteLine(vbTab & " LightBlue is not dark enough to be read accurately. Lets try DarkBlue")
MyVerifiedQR.ChangeBarCodeColor(Color.DarkBlue)
End If
MyVerifiedQR.SaveAsHtmlFile("MyVerifiedQR.html")
' open the barcode html file in your default web browser
System.Diagnostics.Process.Start("MyVerifiedQR.html")
最後幾行程式碼將 QR Code 匯出為不含資源的獨立 HTML 檔案,然後在預設瀏覽器中打開該 HTML 檔案。
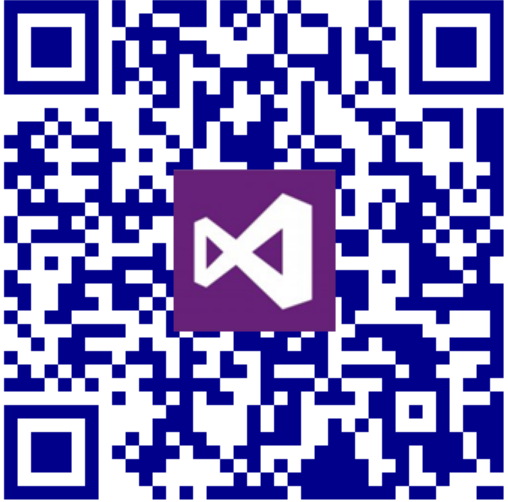
讀取和寫入二進位資料
QR 碼是處理二進位數據的絕佳格式。 有時二進位數據比處理文本更節省空間或更適合。
在此範例中,我們將從字符串編碼一些二進制數據,將其寫入到 QR 格式的條碼中,然後將其作為二進制數據的位元組組讀取回來。 您會注意到這個功能在許多條碼庫中並不常見,使得IronBarcode在此方面具有獨特性。
:path=/static-assets/barcode/content-code-examples/tutorials/csharp-qr-code-generator-5.cs
using IronBarCode;
using System;
using System.Linq;
// Create Some Binary Data - This example equally well for Byte[] and System.IO.Stream
byte[] BinaryData = System.Text.Encoding.UTF8.GetBytes("https://ironsoftware.com/csharp/barcode/");
// WRITE QR with Binary Content
QRCodeWriter.CreateQrCode(BinaryData, 500).SaveAsImage("MyBinaryQR.png");
// READ QR with Binary Content
var MyReturnedData = BarcodeReader.Read("MyBinaryQR.png").First();
if (BinaryData.SequenceEqual(MyReturnedData.BinaryValue))
{
Console.WriteLine("\t Binary Data Read and Written Perfectly");
}
else
{
throw new Exception("Corrupted Data");
}
Imports Microsoft.VisualBasic
Imports IronBarCode
Imports System
Imports System.Linq
' Create Some Binary Data - This example equally well for Byte[] and System.IO.Stream
Private BinaryData() As Byte = System.Text.Encoding.UTF8.GetBytes("https://ironsoftware.com/csharp/barcode/")
' WRITE QR with Binary Content
QRCodeWriter.CreateQrCode(BinaryData, 500).SaveAsImage("MyBinaryQR.png")
' READ QR with Binary Content
Dim MyReturnedData = BarcodeReader.Read("MyBinaryQR.png").First()
If BinaryData.SequenceEqual(MyReturnedData.BinaryValue) Then
Console.WriteLine(vbTab & " Binary Data Read and Written Perfectly")
Else
Throw New Exception("Corrupted Data")
End If
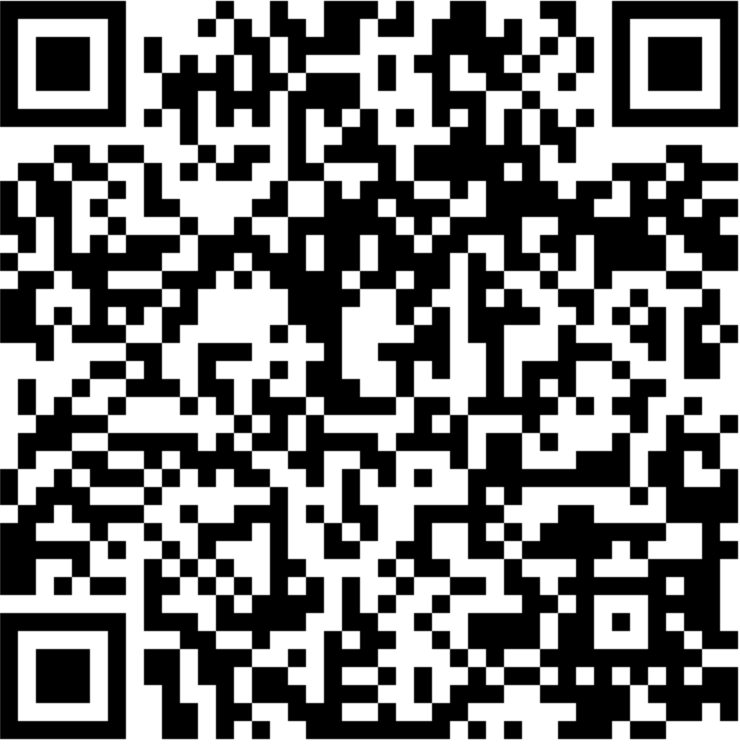
總而言之,Barcode C# Library 是專門為真實世界中的 QR 代碼使用而設計的。 不僅能快速且精確地讀取QR碼,還可以對它們進行樣式設計,添加標誌,驗證條碼,以及用二進制數據進行編碼。
讀取QR碼
為了避免您在教程之間來回跳轉,我將添加一個使用IronBarcode讀取QR碼的我最喜歡的方式的代碼示例。
:path=/static-assets/barcode/content-code-examples/tutorials/csharp-qr-code-generator-6.cs
using IronBarCode;
using System;
using System.Linq;
BarcodeResults result = BarcodeReader.Read("QR.png", new BarcodeReaderOptions() { ExpectBarcodeTypes = BarcodeEncoding.QRCode });
if (result != null)
{
Console.WriteLine(result.First().Value);
}
Imports IronBarCode
Imports System
Imports System.Linq
Private result As BarcodeResults = BarcodeReader.Read("QR.png", New BarcodeReaderOptions() With {.ExpectBarcodeTypes = BarcodeEncoding.QRCode})
If result IsNot Nothing Then
Console.WriteLine(result.First().Value)
End If
還有一種更高級的形式,讓開發人員有更多的控制:
:path=/static-assets/barcode/content-code-examples/tutorials/csharp-qr-code-generator-7.cs
using IronBarCode;
using System;
using System.Linq;
BarcodeReaderOptions options = new BarcodeReaderOptions
{
Speed = ReadingSpeed.Faster,
ExpectMultipleBarcodes = false,
ExpectBarcodeTypes = BarcodeEncoding.All,
Multithreaded = false,
MaxParallelThreads = 0,
CropArea = null,
UseCode39ExtendedMode = false,
RemoveFalsePositive = false,
ImageFilters = null
};
BarcodeResults result = BarcodeReader.Read("QR.png", options);
if (result != null)
{
Console.WriteLine(result.First().Value);
}
Imports IronBarCode
Imports System
Imports System.Linq
Private options As New BarcodeReaderOptions With {
.Speed = ReadingSpeed.Faster,
.ExpectMultipleBarcodes = False,
.ExpectBarcodeTypes = BarcodeEncoding.All,
.Multithreaded = False,
.MaxParallelThreads = 0,
.CropArea = Nothing,
.UseCode39ExtendedMode = False,
.RemoveFalsePositive = False,
.ImageFilters = Nothing
}
Private result As BarcodeResults = BarcodeReader.Read("QR.png", options)
If result IsNot Nothing Then
Console.WriteLine(result.First().Value)
End If
向前邁進
要了解有關此示例和在C# 中使用 QR 代碼的更多資訊,我們鼓勵您在我們的 GitHub 頁面上 fork 它或從我們的網站下載源代碼。
原始碼下載
下載這個C# QR碼生成器教程和DLL。
進一步文件說明
您也可能對 API 參考資料中的QRCodeWriter和BarcodeReader類別感興趣。
他們完整記錄了 IronBarcode 的全部功能集,一個 VB.NET 條碼生成器,不可能全部包含在單個教程中。