使用 IronXL 在 ASP.NET MVC 中读取 Excel 文件
本教程指导开发者在ASP.NET MVC应用程序中使用IronXL实现Excel文件解析。
如何在 ASP.NET 中读取 Excel 文件
创建 ASP.NET 项目
使用 Visual Studio 2022(或类似产品版本)创建一个新的 ASP.NET 项目。 根据特定项目的需要添加额外的NuGet包和源代码。
安装 IronXL 库
立即在您的项目中开始使用IronXL,并享受免费试用。
在创建新项目后,我们需要安装 IronXL 库。 按照以下步骤安装IronXL库。 打开 NuGet 包管理器控制台,并输入以下命令:
Install-Package IronXL.Excel
读取Excel文件
打开ASP.NET项目中的默认控制器(即HomeController)文件,并将Index
方法替换为以下代码:
public ActionResult Index()
{
WorkBook workbook = WorkBook.Load(@"C:\Files\Customer Data.xlsx");
WorkSheet sheet = workbook.WorkSheets.First();
var dataTable = sheet.ToDataTable();
return View(dataTable);
}
public ActionResult Index()
{
WorkBook workbook = WorkBook.Load(@"C:\Files\Customer Data.xlsx");
WorkSheet sheet = workbook.WorkSheets.First();
var dataTable = sheet.ToDataTable();
return View(dataTable);
}
Public Function Index() As ActionResult
Dim workbook As WorkBook = WorkBook.Load("C:\Files\Customer Data.xlsx")
Dim sheet As WorkSheet = workbook.WorkSheets.First()
Dim dataTable = sheet.ToDataTable()
Return View(dataTable)
End Function
在Index
操作方法中,我们使用IronXL的Load
加载Excel文件。 Excel文件的路径(包括文件名)作为参数传递给方法调用。 接下来,我们选择第一个Excel工作表作为工作表,并将其中包含的数据加载到一个Datatable
对象中。 最后,Datatable
被发送到前端。
在网页上显示Excel数据
下一个示例展示了如何在网络浏览器中显示上一个示例中返回的数据表。
此示例中将使用的工作 Excel 文件如下所示:
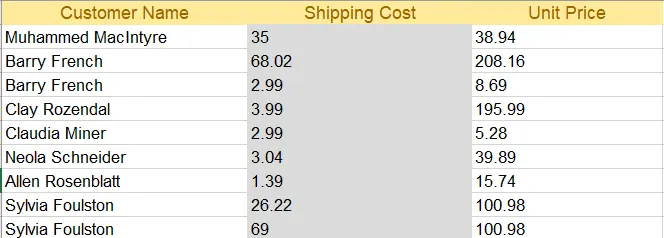
Excel 文件
打开 index.cshtml(索引视图)并将代码替换为以下 HTML 代码。
@{
ViewData ["Title"] = "Home Page";
}
@using System.Data
@model DataTable
<div class="text-center">
<h1 class="display-4">Welcome to IronXL Read Excel MVC</h1>
</div>
<table class="table table-dark">
<tbody>
@foreach (DataRow row in Model.Rows)
{
<tr>
@for (int i = 0; i < Model.Columns.Count; i++)
{
<td>@row [i]</td>
}
</tr>
}
</tbody>
</table>
@{
ViewData ["Title"] = "Home Page";
}
@using System.Data
@model DataTable
<div class="text-center">
<h1 class="display-4">Welcome to IronXL Read Excel MVC</h1>
</div>
<table class="table table-dark">
<tbody>
@foreach (DataRow row in Model.Rows)
{
<tr>
@for (int i = 0; i < Model.Columns.Count; i++)
{
<td>@row [i]</td>
}
</tr>
}
</tbody>
</table>
@
If True Then
ViewData ("Title") = "Home Page"
End If
'INSTANT VB WARNING: An assignment within expression was extracted from the following statement:
'ORIGINAL LINE: using System.Data model DataTable <div class="text-center"> <h1 class="display-4"> Welcome to IronXL Read Excel MVC</h1> </div> <table class="table table-dark"> <tbody> foreach(DataRow row in Model.Rows)
"display-4"> Welcome [to] IronXL Read Excel MVC</h1> </div> <table class="table table-dark"> (Of tbody) foreach(DataRow row in Model.Rows)
If True Then
'INSTANT VB WARNING: An assignment within expression was extracted from the following statement:
'ORIGINAL LINE: using System.Data model DataTable <div class="text-center"> <h1 class="display-4"> Welcome to IronXL Read Excel MVC</h1> </div> <table class
"text-center"> <h1 class="display-4"> Welcome [to] IronXL Read Excel MVC</h1> </div> <table class
[using] System.Data model DataTable <div class="text-center"> <h1 class
'INSTANT VB TODO TASK: Local functions are not converted by Instant VB:
' (Of tr) @for(int i = 0; i < Model.Columns.Count; i++)
' {
' <td> @row [i]</td>
' }
</tr>
End If
'INSTANT VB TODO TASK: The following line uses invalid syntax:
' </tbody> </table>
上述代码使用从Index
方法返回的Datatable
作为模型。 使用@for
循环将表中的每一行打印到网页上,并包括用于装饰的Bootstrap格式。
运行项目将产生以下结果。
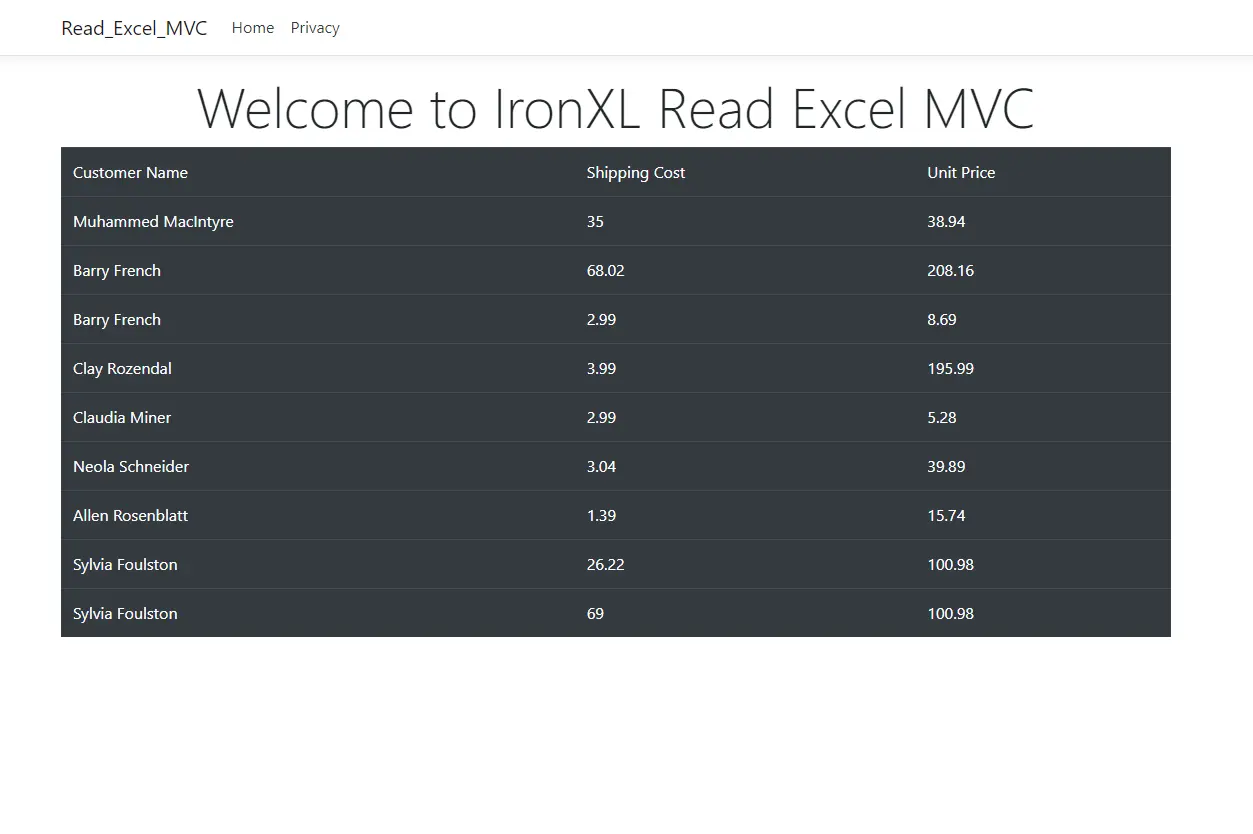
Bootstrap 表格