使用C# OCR从图像中读取文本
在本教程中,我们将学习如何在C#和其他.NET语言中将图像转换为文本。
如何用 C# 将图像转换为文本
- 下载 OCR 图像到文本 IronOCR 库
- 调整裁剪区域以读取图像的部分内容
- 通过语言包使用多达 125 种国际语言
- 将 OCR 扫描结果导出为文本或可搜索 PDF 文件
在 .NET 应用程序中从图像读取文本
我们将使用IronOcr.IronTesseract
类来识别图像中的文本,并探讨如何使用_Iron Tesseract OCR_以获得在.NET中从图像读取文本时的最高性能,无论是在准确性还是速度方面。
为了实现“图像转文本”,我们将在Visual Studio项目中安装IronOCR库。
为此,我们下载 IronOcr DLL 或使用 NuGet .
Install-Package IronOcr
为什么选择IronOCR?
我们使用IronOCR进行Tesseract管理,因为它的独特之处在于:
- 直接在纯.NET环境中即可使用
- 不需要在您的机器上安装Tesseract。
- 运行最新引擎:Tesseract 5(以及魔方 4 & 3)
- 适用于任何 .NET 项目:.NET Framework 4.5 +、.NET Standard 2 + 和 .NET Core 2、3 和 5!
- 具有比传统的Tesseract更高的准确性和速度。
- 支持 Xamarin、Mono、Azure 和 Docker
- 使用 NuGet 包管理复杂的 Tesseract 字典系统。
- 支持PDFS、多帧Tiff和所有主要图像格式,无需配置。
可以纠正低质量和倾斜的扫描,以从tesseract获得最佳结果。
立即在您的项目中开始使用IronOCR,并享受免费试用。
使用 C# 中的 Tesseract
在这个简单的示例中,您可以看到我们使用了 IronOcr.IronTesseract
类从图像中读取文本,并自动将其值返回为字符串。
:path=/static-assets/ocr/content-code-examples/tutorials/how-to-read-text-from-an-image-in-csharp-net-1.cs
// PM> Install-Package IronOcr
using IronOcr;
OcrResult result = new IronTesseract().Read(@"img\Screenshot.png");
Console.WriteLine(result.Text);
' PM> Install-Package IronOcr
Imports IronOcr
Private result As OcrResult = (New IronTesseract()).Read("img\Screenshot.png")
Console.WriteLine(result.Text)
这使得以下文字的准确率达到 100%:
IronOCR Simple Example
In this simple example we will test the accuracy of our C# OCR library to read text from a PNG
Image. This is a very basic test, but things will get more complicated as the tutorial continues.
The quick brown fox jumps over the lazy dog
虽然这看起来可能很简单,但是在“表面之下”其实进行着复杂的操作:扫描图像以确定对齐、质量和分辨率,查看其属性,优化OCR引擎,并使用训练有素的人工智能网络以人类的方式识别文本。
OCR对于计算机来说不是一个简单的过程,其阅读速度可能与人类相似。 换句话说,OCR 不是一个瞬间完成的过程。 在这种情况下,它是百分之百准确的。
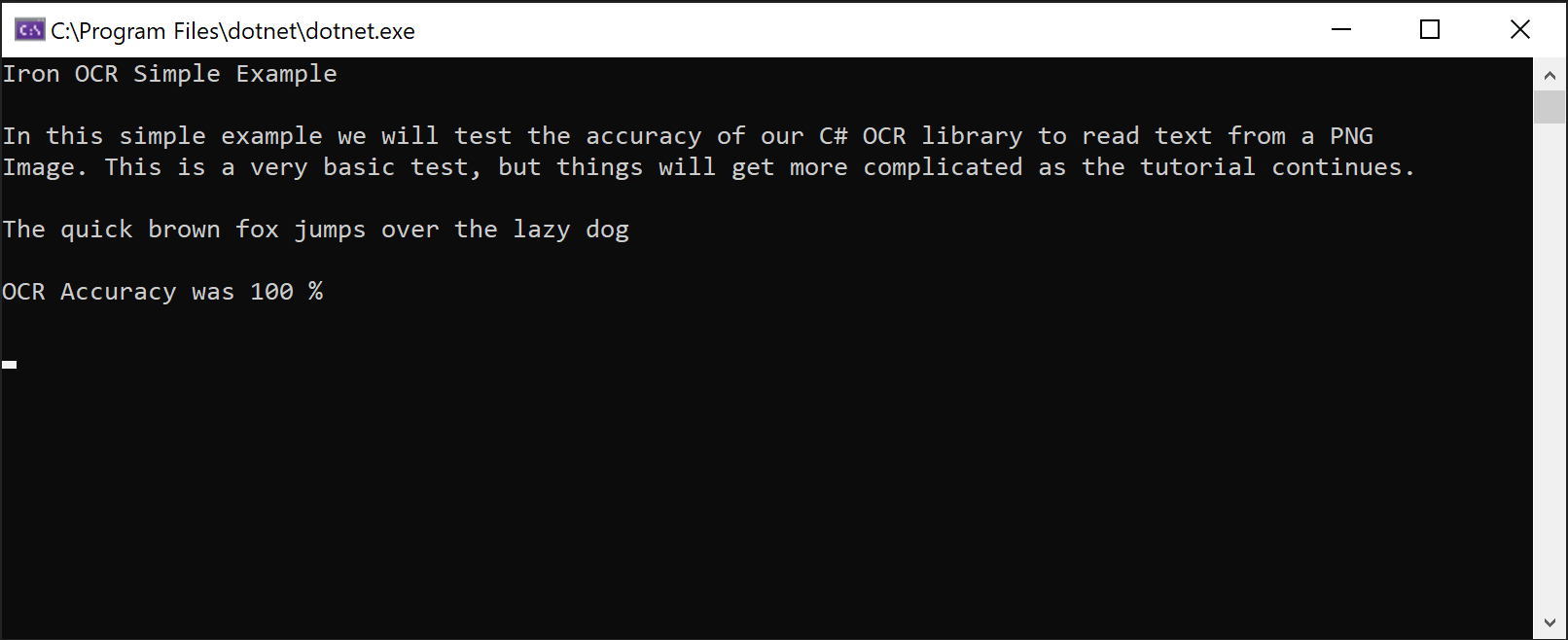
IronOCR Tesseract 的 C# 高级用法
在大多数真实世界的使用情况中,开发者们通常希望为他们的项目获得最佳性能。 在这种情况下,我们建议您继续使用 OcrInput
和 钢铁魔方
IronOcr 命名空间内的类。
OcrInput 允许您设置 OCR 作业的特定特性,例如:
- 处理几乎所有类型的图像,包括JPEG、TIFF、GIF、BMP和PNG
- 导入整个或部分PDF文档
- 增强对比度、分辨率和大小。
纠正旋转、扫描噪声、数字噪声、倾斜和负像
IronTesseract
- 从数百种预包装的语言和语言变体中选择
- 使用 Tesseract 5、4 或 3 OCR 引擎“开箱即用”
- 指定文档类型,无论我们是在查看屏幕截图、代码片段还是整个文档。
- 读取条形码
- 输出结果至:可搜索的PDF、Hocr HTML、DOM和字符串
示例:开始使用 OcrInput + IronTesseract
这一切可能看起来令人生畏,但在下面的示例中,您将看到我们建议您开始使用的默认设置,这些设置几乎适用于您输入到IronOCR的任何图像。
:path=/static-assets/ocr/content-code-examples/tutorials/how-to-read-text-from-an-image-in-csharp-net-2.cs
using IronOcr;
IronTesseract ocr = new IronTesseract();
using OcrInput input = new OcrInput();
var pageindices = new int[] { 1, 2 };
input.LoadImageFrames(@"img\Potter.tiff", pageindices);
OcrResult result = ocr.Read(input);
Console.WriteLine(result.Text);
Imports IronOcr
Private ocr As New IronTesseract()
Private OcrInput As using
Private pageindices = New Integer() { 1, 2 }
input.LoadImageFrames("img\Potter.tiff", pageindices)
Dim result As OcrResult = ocr.Read(input)
Console.WriteLine(result.Text)
即使是中等质量的扫描,我们也可以使用它,准确率达到 100%。
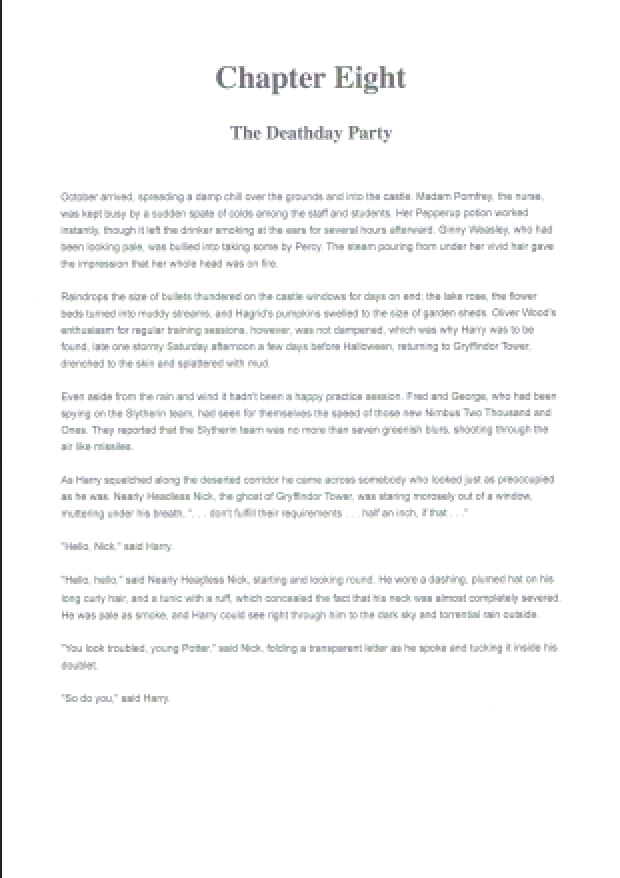
正如您所看到的,阅读文本(并可选择条形码)从扫描图像(例如 TIFF)转换相对简单。
这个OCR任务的准确率达到了100%。
OCR在处理现实世界的文件时并不是一门完美的科学,但IronTesseract已经是目前最好的之一。
您还将注意到,IronOCR可以自动读取多页文档,例如TIFFs甚至从 PDF 文档中提取文本自动。
示例:低质量扫描
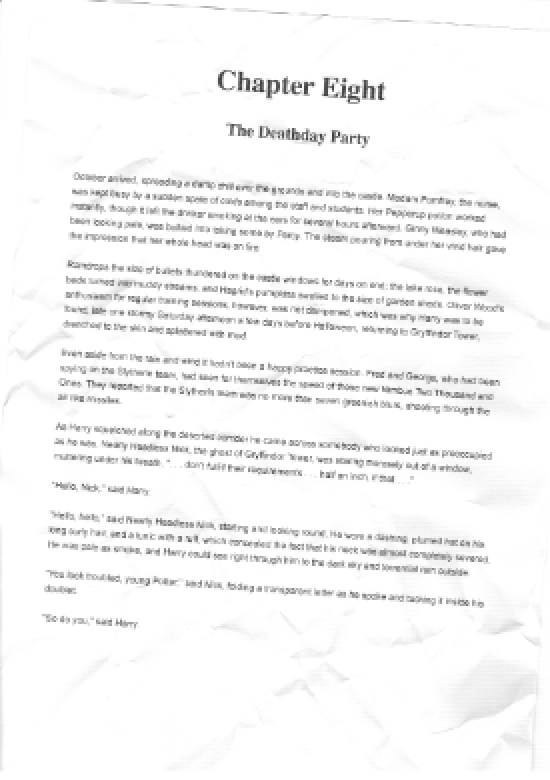
现在我们将尝试对同一页面进行更低质量的扫描,采用低DPI,页面有很多失真、数字噪声和原纸损坏。
这是IronOCR真正胜过其他OCR库(例如Tesseract)的地方,我们会发现其他的OCR项目避免讨论这一点。 在现实世界扫描的图像上进行OCR,而不是在数字化制作的、不切实际的“完美”测试样例上进行,以获得100%的OCR准确率。
:path=/static-assets/ocr/content-code-examples/tutorials/how-to-read-text-from-an-image-in-csharp-net-3.cs
using IronOcr;
IronTesseract ocr = new IronTesseract();
using OcrInput input = new OcrInput();
var pageindices = new int[] { 1, 2 };
input.LoadImageFrames(@"img\Potter.LowQuality.tiff", pageindices);
input.Deskew(); // removes rotation and perspective
OcrResult result = ocr.Read(input);
Console.WriteLine(result.Text);
Imports IronOcr
Private ocr As New IronTesseract()
Private OcrInput As using
Private pageindices = New Integer() { 1, 2 }
input.LoadImageFrames("img\Potter.LowQuality.tiff", pageindices)
input.Deskew() ' removes rotation and perspective
Dim result As OcrResult = ocr.Read(input)
Console.WriteLine(result.Text)
不添加 `Input.Deskew()要矫正图像我们得到了52.5%的准确率。 不够好。
添加 Input.Deskew
()准确率达到99.8%,即 险些 与高质量扫描的OCR一样准确。
图像滤镜可能需要一点时间来运行 - 但也减少了OCR处理时间。这对开发者来说是一个需要很好把握的平衡,以了解他们的输入文件。
如果您不确定:
Input.Deskew()
是一种安全且非常有效的过滤器。- 其次尝试
Input.DeNoise
()修复大量数字噪音。
性能调优
OCR任务的速度最重要的因素实际上是输入图像的质量。 背景噪音越少,分辨率越高,最理想的目标分辨率约为200 dpi,将产生最快速和最准确的OCR结果。
然而,这并非必要,因为IronOCR擅长纠正不完美的文件。(虽然这很耗时,而且会导致 OCR 作业占用更多的 CPU 周期).
如果可能的话,选择数字噪声较少的输入图像格式,如TIFF或PNG,也可以比 有损 图像格式,如 JPEG。
图像滤镜
以下图像过滤器可以显著提高性能:
- OcrInput.Rotate(双学位)** - 向顺时针方向旋转图像若干度。要逆时针旋转,请使用负数。
- OcrInput.Binarize()** - 这个图像滤镜将每个像素变成黑色或白色,没有中间色。 可能提高OCR性能,用于文本与背景对比度非常低的情况。
- OcrInput.ToGrayScale()** - 此图像滤镜将每个像素转换为灰度阴影。 不太可能提高OCR精度,但可以提高速度。
- OcrInput.对比度()** - 自动增加对比度。 此过滤器通常能提高低对比度扫描中的OCR速度和准确性。
- OcrInput.DeNoise()** - 去除数字噪声。此滤镜仅应在预期有噪声的情况下使用。
- OcrInput.Invert()** - 反转每个颜色。 例如,白变黑:黑变白。
- OcrInput.膨胀()** - 高级形态学。 Dilation 在图像中的对象边界处添加像素。 侵蚀的反义词
- OcrInput.Erode()** - 高级形态学。 侵蚀 移除物体边界上的像素,与扩张相反
- OcrInput.Deskew()** - 旋转图像,使其正面朝上并保持正交。 这对OCR非常有用,因为Tesseract对倾斜扫描的容忍度可以低至5度。
- OcrInput.DeepCleanBackgroundNoise()** - 强大的背景噪音消除。 仅在已知文档背景噪声极端的情况下使用此过滤器,因为此过滤器也可能降低清晰文档的OCR精确度,并且非常耗费CPU。
- OcrInput.EnhanceResolution - 增强低质量图像的分辨率。 此过滤器通常不需要,因为_OcrInput.MinimumDPI_和_OcrInput.TargetDPI_将自动捕捉并解决低分辨率输入。
性能调优以提速
使用 Iron Tesseract,我们可能希望加快对高质量扫描的 OCR 速度。
如果要优化速度,我们可能会从这个位置开始,然后逐步打开功能,直到找到完美的平衡点。
:path=/static-assets/ocr/content-code-examples/tutorials/how-to-read-text-from-an-image-in-csharp-net-4.cs
using IronOcr;
IronTesseract ocr = new IronTesseract();
// Configure for speed
ocr.Configuration.BlackListCharacters = "~`$#^*_}{][|\\";
ocr.Configuration.PageSegmentationMode = TesseractPageSegmentationMode.Auto;
ocr.Language = OcrLanguage.EnglishFast;
using OcrInput input = new OcrInput();
var pageindices = new int[] { 1, 2 };
input.LoadImageFrames(@"img\Potter.tiff", pageindices);
OcrResult result = ocr.Read(input);
Console.WriteLine(result.Text);
Imports IronOcr
Private ocr As New IronTesseract()
' Configure for speed
ocr.Configuration.BlackListCharacters = "~`$#^*_}{][|\"
ocr.Configuration.PageSegmentationMode = TesseractPageSegmentationMode.Auto
ocr.Language = OcrLanguage.EnglishFast
Using input As New OcrInput()
Dim pageindices = New Integer() { 1, 2 }
input.LoadImageFrames("img\Potter.tiff", pageindices)
Dim result As OcrResult = ocr.Read(input)
Console.WriteLine(result.Text)
End Using
这一结果的准确率为99.8%,而基准准确率为100%,但速度快了 35%。
读取图像的裁剪区域
如您所见,以下代码示例显示,Iron的Tesseract OCR分支擅长读取图像的特定区域。
我们可能会使用 System.Drawing.Rectangle
以像素为单位指定要读取的图像的确切区域。
这在我们处理填写了标准化表格的情况下非常有用,其中只有某些区域的文本会根据不同情况发生变化。
示例:扫描页面的一个区域
我们可以使用 System.Drawing.Rectangle
指定一个区域来读取文档。 度量单位始终是像素。
我们将看到这样做可以提高速度,同时避免读取不必要的文本。 在这个例子中,我们将从标准文件的中央区域读取学生的姓名。
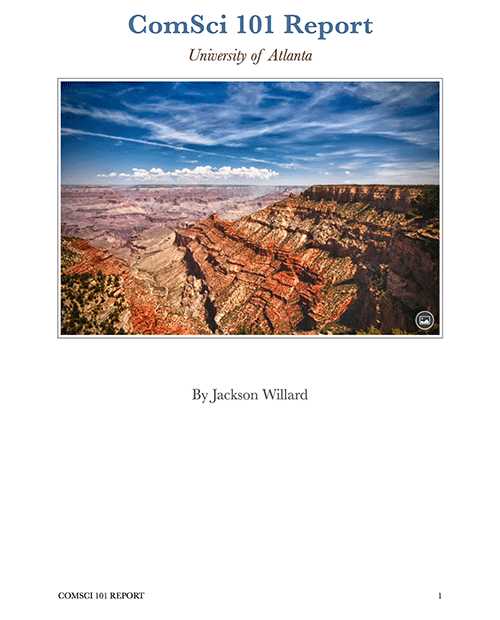
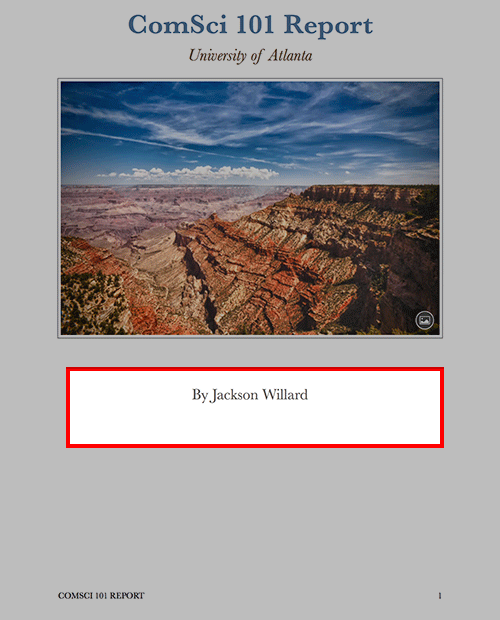
:path=/static-assets/ocr/content-code-examples/tutorials/how-to-read-text-from-an-image-in-csharp-net-5.cs
using IronOcr;
using IronSoftware.Drawing;
IronTesseract ocr = new IronTesseract();
using OcrInput input = new OcrInput();
// a 41% improvement on speed
Rectangle contentArea = new Rectangle(x: 215, y: 1250, height: 280, width: 1335);
input.LoadImage("img/ComSci.png", contentArea);
OcrResult result = ocr.Read(input);
Console.WriteLine(result.Text);
Imports IronOcr
Imports IronSoftware.Drawing
Private ocr As New IronTesseract()
Private OcrInput As using
' a 41% improvement on speed
Private contentArea As New Rectangle(x:= 215, y:= 1250, height:= 280, width:= 1335)
input.LoadImage("img/ComSci.png", contentArea)
Dim result As OcrResult = ocr.Read(input)
Console.WriteLine(result.Text)
这带来了41%的速度提升 - 并且使我们能够具体化。 这对于_.NET OCR_场景非常有用,适用于文件相似且一致的情况,如发票、收据、支票、表格、费用报销等。
ContentAreas(OCR 裁剪)读取 PDF 文件时也支持
国际语言
IronOCR支持通过作为DLL分发的语言包支持125种国际语言,这些语言包可以从本网站下载,也可以从Visual Studio的NuGet包管理器下载。
我们可以通过浏览 NuGet 来安装它们。(搜索 "IronOcr.Languages")或从OCR 语言包页面.
支持的语言包括:
- 南非荷兰语
- 阿姆哈拉语 亦称 አማርኛ
- 阿拉伯语 也被称为 العربية
- 阿拉伯字母 Also known as العربية
- 亚美尼亚字母 也称为 Հայերեն
- Assamese 也被称为 অসমীয়া
阿塞拜疆语,又称为azərbaycan dili。
阿塞拜疆塞尔维克语 也被称为 azərbaycan dili
- 白俄罗斯语 亦称为беларуская мова
- 孟加拉语(也称为Bangla,বাংলা)
- 孟加拉字母 也被称为孟加拉语,বাংলা
- 西藏标准语,也称为藏语,中央藏语 ཡིག་
- Bosnian 又被称为bosanski jezik
- 布列塔尼语 亦称为brezhoneg
- Bulgarian 也被称为 български език
- 加拿大原住民字母 也称为加拿大第一民族、加拿大土著人、加拿大原住民、因纽特人
- 加泰罗尼亚语 Also known as català, valencià
- 宿霧語(亦稱為比沙雅語、宾利沙雅语)
- Czech 亦称为捷克语、čeština、český jazyk
- CherokeeAlphabet,也被称为ᏣᎳᎩ ᎦᏬᏂᎯᏍᏗ,Tsalagi Gawonihisdi
Chinese Simplified 也称为中文(Zhōngwén), 汉语, 漢語_
ChineseSimplifiedVertical Also known as 中文(Zhōngwén), 汉语, 漢語
ChineseTraditional 也被称为中文(Zhōngwén), 汉语, 漢語
ChineseTraditionalVertical 也被称为 中文(Zhōngwén), 汉语, 漢語
- Cherokee 也被称为 ᏣᎳᎩ ᎦᏬᏂᎯᏍᏗ, Tsalagi Gawonihisdi
- 科西嘉语 又称为科西嘉语,科西嘉岛语
- 威尔士语(又称为Cymraeg)
- 西里尔字母 又称为西里尔文字
- 丹麦语也称为丹斯克
- DanishFraktur 也称为 dansk
- 德语(又称为Deutsch)
GermanFraktur 也被称为 Deutsch
DevanagariAlphabet Also known as Nagair,देवनागरी
- Divehi 也被称为 ދިވެހި
- Dzongkha 也被称为 རྫོང་ཁ
- 希腊语 也称为 ελληνικά
- 英语
- MiddleEnglish 也被称为 English(公元 1100-1500 年)_
- 世界语
- 爱沙尼亚语 也被称为 eesti,eesti keel
- 埃塞俄比亚字母 也被称为格尔兹,ግዕዝ,Gəʿəz
- 巴斯克语 也称为euskara,euskera
- 法罗语(又名føroyskt)
- 波斯语(亦称为فارسی)
- 菲律宾语(又称为菲律宾的国家语言,标准化的他加禄语)
- 芬兰语,也被称为苏俄米语,苏俄门基里语。
- 金融 也称为财务、数值和技术文件
- 法语(也称为français,langue française)
- FrakturAlphabet 也称为通用Fraktur,拉丁字母的书法风格
- Frankish 也称为 Frenkisk,古弗兰克语
- 中法语 也被称为 Moyen Français,中世纪法语(ca. 1400-1600 AD)
- WesternFrisian(也被称为Frysk)
- GeorgianAlphabet 也称为 ქართული
- 苏格兰盖尔语,也被称为盖尔语。
- 爱尔兰语(也称为Gaeilge)
- 加利西亚语(又称为galego)
- 古希腊语 也被称为 Ἑλληνική
- 希腊字母 也被称为 ελληνικά
- Gujarati 也称为 ગુજરાતી
- GujaratiAlphabet 也被称为 ગુજરાતી
- GurmukhiAlphabet 也被称为Gurmukhī, ਗੁਰਮੁਖੀ, Shahmukhi, گُرمُکھی, Sihk Script
- 한글,也被称为朝鲜文字、韩文、朝鲜文。
- HangulVerticalAlphabet也被称为韩国字母,한글,Hangeul,朝鲜字,hosŏn'gŭl
- 汉简化字体也称为三韩,韩语
- 汉简化垂直字母,也被称为Samhan,한어,韩语。
- 韓語,又称三韩。
- 漢傳統豎排字母表 又稱為三韓,한어,韓語
- 海地克里奥尔语
- 希伯来语 亦称为עברית
- HebrewAlphabet Also known as 希伯来文
- Hindi 也被称为 हिन्दी, हिंदी
- 克罗地亚语(又称为 hrvatski jezik)
- 匈牙利语(也称为马扎尔语)
- 亚美尼亚语 也称为Հայերեն
- 因纽特语 也称为ᐃᓄᒃᑎᑐᑦ
- 印度尼西亚语(又称为印尼语)
- 冰岛语 也称为Íslenska
- 意大利语 又称为意大利语
- ItalianOld也被称为italiano
- 日本字母 也被称为 日本語(にほんご)
- 日本垂直字母 也被称为日本语(にほんご)
- 爪哇语 也被称为basa Jawa
- 日本文,又称为日本語(にほんご)_
- 日本垂直,也称为日本语(にほんご)_
- 卡纳达语 也被称为 ಕನ್ನಡ
- KannadaAlphabet 也被称为 ಕನ್ನಡ
- 格鲁吉亚语 也称为 ქართული
- 格鲁吉亚老字体(也称为ქართული)
哈萨克语(又称қазақ тілі)
Khmer 也被称为ខ្មែរ、ខេមរភាសា、ភាសាខ្មែរ
KhmerAlphabet 也称为 ខ្មែរ, ខេមរភាសា, ភាសាខ្មែរ
- 吉尔吉斯语 也被称为Кыргызча、Кыргыз тили
- 北库尔德语 又称库尔曼吉语,کورمانجی,Kurmancî
- 한국어(韓國語), 조선어(朝鮮語)_
KoreanVertical Also known as 韩国语(韓國語), 조선어(朝鮮語)
Lao Also known as ພາສາລາວ
- LaoAlphabet 也被称为 ພາສາລາວ
- 拉丁语 也被称为 latine, lingua latina
- 拉丁字母 也被称为拉丁文,拉丁语
- Latvian(也被称为latviešu valoda)
- 立陶宛语(又称为lietuvių kalba)
- 卢森堡语(又称为Lëtzebuergesch)
- Malayalam 又称为 മലയാളം
- MalayalamAlphabet 也被称为 മലയാളം
- Marathi 也称为मराठी
- MICR(又称磁性墨水字符识别,MICR支票编码)
- 马其顿语(又称为македонски јазик)
- 马耳他语 亦称为 Malti
- 蒙古语 又称为 монгол
- 毛利语(也称为te reo Māori)
- 马来语,也被称为巴哈萨马来语,بهاس ملايو
- Myanmar 也称为缅甸语,ဗမာစာ
- MyanmarAlphabet 也称为 Burmese,ဗမာစာ
- 尼泊尔语(又称नेपाली)
- 荷兰语(又称尼德兰语、佛兰芒语)
- 挪威语(又称为Norsk)
- 奥克语 又称为奥克语,lenga d'òc
奥里亚语 也被称为 ଓଡ଼ିଆ
OriyaAlphabet 也被称为 ଓଡ଼ିଆ
- Panjabi 也被称为 ਪੰਜਾਬੀ, پنجابی
- 波兰语(也被称为język polski,polszczyzna)
- 葡萄牙语 也被称为 português
- Pashto 也被称为 پښتو
- Quechua 也称为Runa Simi,Kichwa
- 罗马尼亚语又称为limba română
- Russian 也被称为 русский язык
- Sanskrit 也被称为 संस्कृतम्
- Sinhala 也被称为 සිංහල
- SinhalaAlphabet 也被称为 සිංහල
斯洛伐克语(又称为slovenčina, slovenský jazyk)
SlovakFraktur 也被称为slovenčina,slovenský jazyk
- Slovene 也被称为斯洛文尼亚语,斯洛文尼亚斯奇纳
- Sindhi 也被称为 सिन्धी, سنڌي، سندھی
- 西班牙语 也被称为 español,castellano
- 西班牙语又称为español,castellano。
阿尔巴尼亚语,又称为gjuha shqipe。
Also known as српски језик
SerbianLatin Also known as српски језик
- 巽他语 又称为巴萨巽他
- 斯瓦希里语(又称基斯瓦希里语)
- 瑞典语(又称为Svenska)
- Syriac,也被称为Syrian、Syriac Aramaic、ܠܫܢܐ ܣܘܪܝܝܐ、Leššānā Suryāyā
Also known as Syrian, Syriac Aramaic, ܠܫܢܐ ܣܘܨܝܝܐ, Leššānā Suryāyā
泰米尔语 也称为தமிழ்
- TamilAlphabet 也被称为 தமிழ்
- 也被称为татар теле,tatar tele
- Telugu 也被称为 తెలుగు
- TeluguAlphabet 也称为 తెలుగు
- Tajik 也被称为 тоҷикӣ, toğikī, تاجیکی
- Tagalog 也被称为Wikang Tagalog, ᜏᜒᜃᜅ᜔ ᜆᜄᜎᜓᜄ᜔
- 泰语 Also known as ไทย
- ThaanaAlphabet 也被称为 Taana,Tāna,ތާނަ
ThaiAlphabet Also known as ไทย
TibetanAlphabet Also known as Tibetan Standard, Tibetan, Central ཡིག་
- 提格利尼亚语 也被称为 ትግርኛ
- 汤加(又名 faka Tonga)
土耳其语 也被称为 Türkçe
Uyghur 也称为Uyƣurqə, ئۇيغۇرچە
- 乌克兰语 又称为українська мова
- Urdu 也被称为 اردو
- 乌兹别克 Also known as 乌兹别克, Ўзбек, أۇزبېك
- UzbekCyrillic 亦称为 O‘zbek, Ўзбек, أۇزبېك
- 越南语 也称为 Tiếng Việt
- VietnameseAlphabet 也称为 Tiếng Việt
- Also known as ייִדיש
- Yoruba 也被称为约鲁巴语
示例:阿拉伯语的OCR(以及更多)
在以下示例中,我们将展示如何扫描阿拉伯文文件。
PM> Install-Package IronOcr.Languages.Arabic
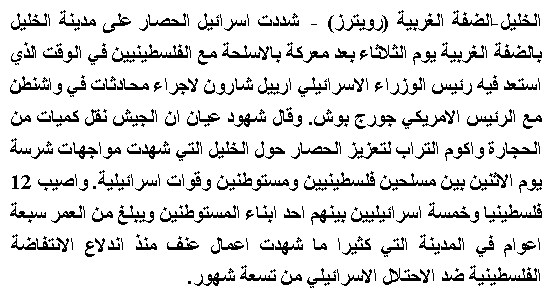
:path=/static-assets/ocr/content-code-examples/tutorials/how-to-read-text-from-an-image-in-csharp-net-6.cs
// PM> Install IronOcr.Languages.Arabic
using IronOcr;
IronTesseract ocr = new IronTesseract();
ocr.Language = OcrLanguage.Arabic;
using OcrInput input = new OcrInput();
input.LoadImageFrame("img/arabic.gif", 1);
// add image filters if needed
// In this case, even thought input is very low quality
// IronTesseract can read what conventional Tesseract cannot.
OcrResult result = ocr.Read(input);
// Console can't print Arabic on Windows easily.
// Let's save to disk instead.
result.SaveAsTextFile("arabic.txt");
' PM> Install IronOcr.Languages.Arabic
Imports IronOcr
Private ocr As New IronTesseract()
ocr.Language = OcrLanguage.Arabic
Using input As New OcrInput()
input.LoadImageFrame("img/arabic.gif", 1)
' add image filters if needed
' In this case, even thought input is very low quality
' IronTesseract can read what conventional Tesseract cannot.
Dim result As OcrResult = ocr.Read(input)
' Console can't print Arabic on Windows easily.
' Let's save to disk instead.
result.SaveAsTextFile("arabic.txt")
End Using
示例:在同一文档中使用多种语言进行光学字符识别。
在下面的示例中,我们将展示如何将多种语言的OCR扫描到同一个文档中。
这其实很常见,例如一个中文文件可能包含英文单词和网址。
PM> Install-Package IronOcr.Languages.ChineseSimplified
:path=/static-assets/ocr/content-code-examples/tutorials/how-to-read-text-from-an-image-in-csharp-net-7.cs
using IronOcr;
IronTesseract ocr = new IronTesseract();
ocr.Language = OcrLanguage.ChineseSimplified;
// We can add any number of languages.
ocr.AddSecondaryLanguage(OcrLanguage.English);
// Optionally add custom tesseract .traineddata files by specifying a file path
using OcrInput input = new OcrInput();
input.LoadImage("img/MultiLanguage.jpeg");
OcrResult result = ocr.Read(input);
result.SaveAsTextFile("MultiLanguage.txt");
Imports IronOcr
Private ocr As New IronTesseract()
ocr.Language = OcrLanguage.ChineseSimplified
' We can add any number of languages.
ocr.AddSecondaryLanguage(OcrLanguage.English)
' Optionally add custom tesseract .traineddata files by specifying a file path
Using input As New OcrInput()
input.LoadImage("img/MultiLanguage.jpeg")
Dim result As OcrResult = ocr.Read(input)
result.SaveAsTextFile("MultiLanguage.txt")
End Using
多页文档
IronOcr 可以将多个页面/图像合并为单个 OcrResult
。 这在文档是由多个图像制成的情况下极其有用。 我们稍后将看到,IronTesseract 的这一特殊功能在将 OCR 输入转换为可搜索的 PDF 和 HTML 文件时极其有用。
IronOCR使得可以将图像、TIFF帧和PDF页面“混合匹配”成一个OCR输入。
:path=/static-assets/ocr/content-code-examples/tutorials/how-to-read-text-from-an-image-in-csharp-net-8.cs
using IronOcr;
IronTesseract ocr = new IronTesseract();
using OcrInput input = new OcrInput();
input.LoadImage("image1.jpeg");
input.LoadImage("image2.png");
var pageindices = new int[] { 1, 2 };
input.LoadImageFrames("image3.gif", pageindices);
OcrResult result = ocr.Read(input);
Console.WriteLine($"{result.Pages.Length} Pages"); // 3 Pages
Imports IronOcr
Private ocr As New IronTesseract()
Private OcrInput As using
input.LoadImage("image1.jpeg")
input.LoadImage("image2.png")
Dim pageindices = New Integer() { 1, 2 }
input.LoadImageFrames("image3.gif", pageindices)
Dim result As OcrResult = ocr.Read(input)
Console.WriteLine($"{result.Pages.Length} Pages") ' 3 Pages
我们还可以轻松地对 TIFF 文件的每一页进行 OCR 识别。
:path=/static-assets/ocr/content-code-examples/tutorials/how-to-read-text-from-an-image-in-csharp-net-9.cs
using IronOcr;
IronTesseract ocr = new IronTesseract();
using OcrInput input = new OcrInput();
var pageindices = new int[] { 1, 2 };
input.LoadImageFrames("MultiFrame.Tiff", pageindices);
OcrResult result = ocr.Read(input);
Console.WriteLine(result.Text);
Console.WriteLine($"{result.Pages.Length} Pages");
// 1 page for every frame (page) in the TIFF
Imports IronOcr
Private ocr As New IronTesseract()
Private OcrInput As using
Private pageindices = New Integer() { 1, 2 }
input.LoadImageFrames("MultiFrame.Tiff", pageindices)
Dim result As OcrResult = ocr.Read(input)
Console.WriteLine(result.Text)
Console.WriteLine($"{result.Pages.Length} Pages")
' 1 page for every frame (page) in the TIFF
:path=/static-assets/ocr/content-code-examples/tutorials/how-to-read-text-from-an-image-in-csharp-net-10.cs
using IronOcr;
IronTesseract ocr = new IronTesseract();
using OcrInput input = new OcrInput();
input.LoadPdf("example.pdf", Password: "password");
// We can also select specific PDF page numbers to OCR
OcrResult result = ocr.Read(input);
Console.WriteLine(result.Text);
Console.WriteLine($"{result.Pages.Length} Pages");
// 1 page for every page of the PDF
Imports IronOcr
Private ocr As New IronTesseract()
Private OcrInput As using
input.LoadPdf("example.pdf", Password:= "password")
' We can also select specific PDF page numbers to OCR
Dim result As OcrResult = ocr.Read(input)
Console.WriteLine(result.Text)
Console.WriteLine($"{result.Pages.Length} Pages")
' 1 page for every page of the PDF
可搜索的PDF
在C#和VB.NET中将OCR结果导出为可搜索的PDF是IronOCR的一个热门功能。 这可以真正帮助数据库填充、搜索引擎优化和PDF可用性的企业和政府。
:path=/static-assets/ocr/content-code-examples/tutorials/how-to-read-text-from-an-image-in-csharp-net-11.cs
using IronOcr;
IronTesseract ocr = new IronTesseract();
using OcrInput input = new OcrInput();
input.Title = "Quarterly Report";
input.LoadImage("image1.jpeg");
input.LoadImage("image2.png");
var pageindices = new int[] { 1, 2 };
input.LoadImageFrames("image3.gif", pageindices);
OcrResult result = ocr.Read(input);
result.SaveAsSearchablePdf("searchable.pdf");
Imports IronOcr
Private ocr As New IronTesseract()
Private OcrInput As using
input.Title = "Quarterly Report"
input.LoadImage("image1.jpeg")
input.LoadImage("image2.png")
Dim pageindices = New Integer() { 1, 2 }
input.LoadImageFrames("image3.gif", pageindices)
Dim result As OcrResult = ocr.Read(input)
result.SaveAsSearchablePdf("searchable.pdf")
另一个 OCR 技巧是将现有 PDF 文档转换为可搜索文档。
:path=/static-assets/ocr/content-code-examples/tutorials/how-to-read-text-from-an-image-in-csharp-net-12.cs
using IronOcr;
IronTesseract ocr = new IronTesseract();
using OcrInput input = new OcrInput();
input.Title = "Pdf Metadata Name";
input.LoadPdf("example.pdf", Password: "password");
OcrResult result = ocr.Read(input);
result.SaveAsSearchablePdf("searchable.pdf");
Imports IronOcr
Private ocr As New IronTesseract()
Private OcrInput As using
input.Title = "Pdf Metadata Name"
input.LoadPdf("example.pdf", Password:= "password")
Dim result As OcrResult = ocr.Read(input)
result.SaveAsSearchablePdf("searchable.pdf")
使用 IronTesseract 将一页或多页的 TIFF 文件转换为可搜索的 PDF 文件也是如此。
:path=/static-assets/ocr/content-code-examples/tutorials/how-to-read-text-from-an-image-in-csharp-net-13.cs
using IronOcr;
IronTesseract ocr = new IronTesseract();
using OcrInput input = new OcrInput();
input.Title = "Pdf Title";
var pageindices = new int[] { 1, 2 };
input.LoadImageFrames("example.tiff", pageindices);
OcrResult result = ocr.Read(input);
result.SaveAsSearchablePdf("searchable.pdf");
Imports IronOcr
Private ocr As New IronTesseract()
Private OcrInput As using
input.Title = "Pdf Title"
Dim pageindices = New Integer() { 1, 2 }
input.LoadImageFrames("example.tiff", pageindices)
Dim result As OcrResult = ocr.Read(input)
result.SaveAsSearchablePdf("searchable.pdf")
导出 Hocr HTML
我们可以同样将OCR结果文档导出为Hocr HTML。 这是一个XML文档,可以通过XML阅读器进行解析,或标记为视觉上吸引人的HTML。
这允许一定程度上的PDF到HTML和TIFF到HTML转换。
:path=/static-assets/ocr/content-code-examples/tutorials/how-to-read-text-from-an-image-in-csharp-net-14.cs
using IronOcr;
IronTesseract ocr = new IronTesseract();
using OcrInput input = new OcrInput();
input.Title = "Html Title";
// Add more content as required...
input.LoadImage("image2.jpeg");
input.LoadPdf("example.pdf",Password: "password");
var pageindices = new int[] { 1, 2 };
input.LoadImageFrames("example.tiff", pageindices);
OcrResult result = ocr.Read(input);
result.SaveAsHocrFile("hocr.html");
Imports IronOcr
Private ocr As New IronTesseract()
Private OcrInput As using
input.Title = "Html Title"
' Add more content as required...
input.LoadImage("image2.jpeg")
input.LoadPdf("example.pdf",Password:= "password")
Dim pageindices = New Integer() { 1, 2 }
input.LoadImageFrames("example.tiff", pageindices)
Dim result As OcrResult = ocr.Read(input)
result.SaveAsHocrFile("hocr.html")
在OCR文档中读取条形码
IronOCR相比传统的tesseract有一个独特的额外优势,它还能读取条形码和二维码;
:path=/static-assets/ocr/content-code-examples/tutorials/how-to-read-text-from-an-image-in-csharp-net-15.cs
using IronOcr;
IronTesseract ocr = new IronTesseract();
ocr.Configuration.ReadBarCodes = true;
using OcrInput input = new OcrInput();
input.LoadImage("img/Barcode.png");
OcrResult result = ocr.Read(input);
foreach (var barcode in result.Barcodes)
{
Console.WriteLine(barcode.Value);
// type and location properties also exposed
}
Imports IronOcr
Private ocr As New IronTesseract()
ocr.Configuration.ReadBarCodes = True
Using input As New OcrInput()
input.LoadImage("img/Barcode.png")
Dim result As OcrResult = ocr.Read(input)
For Each barcode In result.Barcodes
Console.WriteLine(barcode.Value)
' type and location properties also exposed
Next barcode
End Using
深入探讨图像到文本OCR结果
在本教程中,我们将要查看的最后一项内容是OCR结果对象。 当我们阅读OCR时,通常只想要文本输出,但IronOCR实际上包含了大量对高级开发者可能有用的信息。
在OCR结果对象中,我们有一个可以迭代的页面集合。 在每一页中,我们可能会找到条形码、功率图、文本行、单词和字符。
每个对象实际上包含:一个位置; X坐标; Y坐标; 宽度和高度; 其中包含可以检查的图像; a font name; 字体大小;文本的书写方向; 文本的旋转; 以及IronOCR对那个特定单词、行或段落的统计置信度。
简而言之,这使得开发者可以以他们选择的任何方式创造性地处理和导出 OCR 数据。
我们还可以处理并从.NET OCR结果对象中导出任何元素,例如段落、单词或条形码,作为图像或位图。
:path=/static-assets/ocr/content-code-examples/tutorials/how-to-read-text-from-an-image-in-csharp-net-16.cs
using IronOcr;
using IronSoftware.Drawing;
// We can delve deep into OCR results as an object model of Pages, Barcodes, Paragraphs, Lines, Words and Characters
// This allows us to explore, export and draw OCR content using other APIs
IronTesseract ocr = new IronTesseract();
ocr.Configuration.ReadBarCodes = true;
using OcrInput input = new OcrInput();
var pageindices = new int[] { 1, 2 };
input.LoadImageFrames(@"img\Potter.tiff", pageindices);
OcrResult result = ocr.Read(input);
foreach (var page in result.Pages)
{
// Page object
int pageNumber = page.PageNumber;
string pageText = page.Text;
int pageWordCount = page.WordCount;
// null if we don't set Ocr.Configuration.ReadBarCodes = true;
OcrResult.Barcode[] barcodes = page.Barcodes;
AnyBitmap pageImage = page.ToBitmap(input);
System.Drawing.Bitmap pageImageLegacy = page.ToBitmap(input);
double pageWidth = page.Width;
double pageHeight = page.Height;
foreach (var paragraph in page.Paragraphs)
{
// Pages -> Paragraphs
int paragraphNumber = paragraph.ParagraphNumber;
String paragraphText = paragraph.Text;
System.Drawing.Bitmap paragraphImage = paragraph.ToBitmap(input);
int paragraphXLocation = paragraph.X;
int paragraphYLocation = paragraph.Y;
int paragraphWidth = paragraph.Width;
int paragraphHeight = paragraph.Height;
double paragraphOcrAccuracy = paragraph.Confidence;
var paragraphTextDirection = paragraph.TextDirection;
foreach (var line in paragraph.Lines)
{
// Pages -> Paragraphs -> Lines
int lineNumber = line.LineNumber;
String lineText = line.Text;
AnyBitmap lineImage = line.ToBitmap(input);
System.Drawing.Bitmap lineImageLegacy = line.ToBitmap(input);
int lineXLocation = line.X;
int lineYLocation = line.Y;
int lineWidth = line.Width;
int lineHeight = line.Height;
double lineOcrAccuracy = line.Confidence;
double lineSkew = line.BaselineAngle;
double lineOffset = line.BaselineOffset;
foreach (var word in line.Words)
{
// Pages -> Paragraphs -> Lines -> Words
int wordNumber = word.WordNumber;
String wordText = word.Text;
AnyBitmap wordImage = word.ToBitmap(input);
System.Drawing.Image wordImageLegacy = word.ToBitmap(input);
int wordXLocation = word.X;
int wordYLocation = word.Y;
int wordWidth = word.Width;
int wordHeight = word.Height;
double wordOcrAccuracy = word.Confidence;
if (word.Font != null)
{
// Word.Font is only set when using Tesseract Engine Modes rather than LTSM
String fontName = word.Font.FontName;
double fontSize = word.Font.FontSize;
bool isBold = word.Font.IsBold;
bool isFixedWidth = word.Font.IsFixedWidth;
bool isItalic = word.Font.IsItalic;
bool isSerif = word.Font.IsSerif;
bool isUnderlined = word.Font.IsUnderlined;
bool fontIsCaligraphic = word.Font.IsCaligraphic;
}
foreach (var character in word.Characters)
{
// Pages -> Paragraphs -> Lines -> Words -> Characters
int characterNumber = character.CharacterNumber;
String characterText = character.Text;
AnyBitmap characterImage = character.ToBitmap(input);
System.Drawing.Bitmap characterImageLegacy = character.ToBitmap(input);
int characterXLocation = character.X;
int characterYLocation = character.Y;
int characterWidth = character.Width;
int characterHeight = character.Height;
double characterOcrAccuracy = character.Confidence;
// Output alternative symbols choices and their probability.
// Very useful for spell checking
OcrResult.Choice[] characterChoices = character.Choices;
}
}
}
}
}
Imports IronOcr
Imports IronSoftware.Drawing
' We can delve deep into OCR results as an object model of Pages, Barcodes, Paragraphs, Lines, Words and Characters
' This allows us to explore, export and draw OCR content using other APIs
Private ocr As New IronTesseract()
ocr.Configuration.ReadBarCodes = True
Using input As New OcrInput()
Dim pageindices = New Integer() { 1, 2 }
input.LoadImageFrames("img\Potter.tiff", pageindices)
Dim result As OcrResult = ocr.Read(input)
For Each page In result.Pages
' Page object
Dim pageNumber As Integer = page.PageNumber
Dim pageText As String = page.Text
Dim pageWordCount As Integer = page.WordCount
' null if we don't set Ocr.Configuration.ReadBarCodes = true;
Dim barcodes() As OcrResult.Barcode = page.Barcodes
Dim pageImage As AnyBitmap = page.ToBitmap(input)
Dim pageImageLegacy As System.Drawing.Bitmap = page.ToBitmap(input)
Dim pageWidth As Double = page.Width
Dim pageHeight As Double = page.Height
For Each paragraph In page.Paragraphs
' Pages -> Paragraphs
Dim paragraphNumber As Integer = paragraph.ParagraphNumber
Dim paragraphText As String = paragraph.Text
Dim paragraphImage As System.Drawing.Bitmap = paragraph.ToBitmap(input)
Dim paragraphXLocation As Integer = paragraph.X
Dim paragraphYLocation As Integer = paragraph.Y
Dim paragraphWidth As Integer = paragraph.Width
Dim paragraphHeight As Integer = paragraph.Height
Dim paragraphOcrAccuracy As Double = paragraph.Confidence
Dim paragraphTextDirection = paragraph.TextDirection
For Each line In paragraph.Lines
' Pages -> Paragraphs -> Lines
Dim lineNumber As Integer = line.LineNumber
Dim lineText As String = line.Text
Dim lineImage As AnyBitmap = line.ToBitmap(input)
Dim lineImageLegacy As System.Drawing.Bitmap = line.ToBitmap(input)
Dim lineXLocation As Integer = line.X
Dim lineYLocation As Integer = line.Y
Dim lineWidth As Integer = line.Width
Dim lineHeight As Integer = line.Height
Dim lineOcrAccuracy As Double = line.Confidence
Dim lineSkew As Double = line.BaselineAngle
Dim lineOffset As Double = line.BaselineOffset
For Each word In line.Words
' Pages -> Paragraphs -> Lines -> Words
Dim wordNumber As Integer = word.WordNumber
Dim wordText As String = word.Text
Dim wordImage As AnyBitmap = word.ToBitmap(input)
Dim wordImageLegacy As System.Drawing.Image = word.ToBitmap(input)
Dim wordXLocation As Integer = word.X
Dim wordYLocation As Integer = word.Y
Dim wordWidth As Integer = word.Width
Dim wordHeight As Integer = word.Height
Dim wordOcrAccuracy As Double = word.Confidence
If word.Font IsNot Nothing Then
' Word.Font is only set when using Tesseract Engine Modes rather than LTSM
Dim fontName As String = word.Font.FontName
Dim fontSize As Double = word.Font.FontSize
Dim isBold As Boolean = word.Font.IsBold
Dim isFixedWidth As Boolean = word.Font.IsFixedWidth
Dim isItalic As Boolean = word.Font.IsItalic
Dim isSerif As Boolean = word.Font.IsSerif
Dim isUnderlined As Boolean = word.Font.IsUnderlined
Dim fontIsCaligraphic As Boolean = word.Font.IsCaligraphic
End If
For Each character In word.Characters
' Pages -> Paragraphs -> Lines -> Words -> Characters
Dim characterNumber As Integer = character.CharacterNumber
Dim characterText As String = character.Text
Dim characterImage As AnyBitmap = character.ToBitmap(input)
Dim characterImageLegacy As System.Drawing.Bitmap = character.ToBitmap(input)
Dim characterXLocation As Integer = character.X
Dim characterYLocation As Integer = character.Y
Dim characterWidth As Integer = character.Width
Dim characterHeight As Integer = character.Height
Dim characterOcrAccuracy As Double = character.Confidence
' Output alternative symbols choices and their probability.
' Very useful for spell checking
Dim characterChoices() As OcrResult.Choice = character.Choices
Next character
Next word
Next line
Next paragraph
Next page
End Using
摘要
IronOCR为C#开发者提供最先进的魔方应用程序接口我们知道的在任何平台上。
IronOCR 可以部署在 Windows、Linux、Mac、Azure、AWS、Lambda 上,并支持 .NET Framework、.NET Standard 和 .NET Core 项目。
即使我们向IronOCR输入一个格式不完美、倾斜且有数字噪音的文档,它也能以约99%的统计精度准确读取其内容。
我们还可以在OCR扫描中读取条形码,并且还可以将我们的OCR导出为HTML和可搜索的PDF。
这是IronOCR独有的功能,你在标准OCR库或普通Tesseract中找不到这个功能。
向前发展
要继续了解更多关于IronOCR的信息,我们建议您:
- 开始使用我们的C# Tesseract OCR 快速入门指导。
- 探索C# 和 VB 代码示例
- 阅读深入分析MSDN 风格 API 参考.