C# .NET QR 码生成器
如您可能在“创建条形码”入门教程中阅读到的,使用IronBarcode创建、设计和导出条形码为图像非常简单,通常可通过一行代码完成。
在这个示例中,我们将更深入地研究QR码,它在工业应用和零售领域的普及度越来越高。
如何在 C# 中读取并生成 QR 码
- Download QR code Generator Library
- 添加一行代码即可创建 QR 代码
- 将您的徽标添加到 QR 代码中
- 验证您的 QR 代码是否可读
- 读写二进制数据的 QR
在C#中安装QR码生成库
立即在您的项目中开始使用IronBarcode,并享受免费试用。
在开始之前,我们需要安装BarCode NuGet 包。
Install-Package BarCode
https://www.nuget.org/packages/BarCode/ 作为替代方案,可以下载 IronBarCode.Dll,并将其作为引用添加到您的项目中,从[.NET Barcode DLL]引用。
导入命名空间
对于本教程,我们需要确保我们的类文件引用IronBarcode以及一些常规系统程序集。
using IronBarCode;
using System;
using System.Drawing;
using System.Linq;
using IronBarCode;
using System;
using System.Drawing;
using System.Linq;
Imports IronBarCode
Imports System
Imports System.Drawing
Imports System.Linq
使用一行代码创建一个 QR 码
我们的第一个示例向我们展示了如何创建一个标准化的条形码,其中包含一些简单的文本,500像素的正方形直径,以及中等错误更正。
:path=/static-assets/barcode/content-code-examples/tutorials/csharp-qr-code-generator-1.cs
using IronBarCode;
// Generate a Simple BarCode image and save as PDF
QRCodeWriter.CreateQrCode("hello world", 500, QRCodeWriter.QrErrorCorrectionLevel.Medium).SaveAsPng("MyQR.png");
Imports IronBarCode
' Generate a Simple BarCode image and save as PDF
QRCodeWriter.CreateQrCode("hello world", 500, QRCodeWriter.QrErrorCorrectionLevel.Medium).SaveAsPng("MyQR.png")
纠错功能使我们能够定义在现实世界情况下二维码的可读性有多高。 更高的错误纠正级别会生成具有更多像素和更复杂的较大QR码。
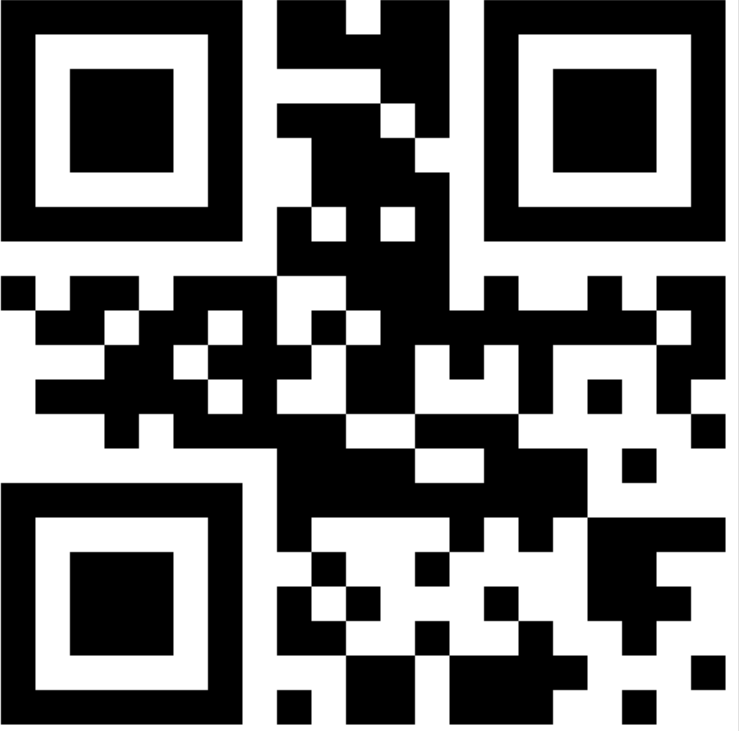
添加标志
在第二个示例中,我们将查看一个用例,即公司希望在其二维码中添加 logo,这是我们现在常见的做法。 通过使用 QRCodeWriter.CreateQRCodeWithLogo 方法,在下面的代码示例中您可以看到这有多简单。
:path=/static-assets/barcode/content-code-examples/tutorials/csharp-qr-code-generator-2.cs
using IronBarCode;
using IronSoftware.Drawing;
// You may add styling with color, logo images or branding:
QRCodeLogo qrCodeLogo = new QRCodeLogo("visual-studio-logo.png");
GeneratedBarcode myQRCodeWithLogo = QRCodeWriter.CreateQrCodeWithLogo("https://ironsoftware.com/", qrCodeLogo);
myQRCodeWithLogo.ResizeTo(500, 500).SetMargins(10).ChangeBarCodeColor(Color.DarkGreen);
// Logo will automatically be sized appropriately and snapped to the QR grid.
myQRCodeWithLogo.SaveAsPng("myQRWithLogo.png");
Imports IronBarCode
Imports IronSoftware.Drawing
' You may add styling with color, logo images or branding:
Private qrCodeLogo As New QRCodeLogo("visual-studio-logo.png")
Private myQRCodeWithLogo As GeneratedBarcode = QRCodeWriter.CreateQrCodeWithLogo("https://ironsoftware.com/", qrCodeLogo)
myQRCodeWithLogo.ResizeTo(500, 500).SetMargins(10).ChangeBarCodeColor(Color.DarkGreen)
' Logo will automatically be sized appropriately and snapped to the QR grid.
myQRCodeWithLogo.SaveAsPng("myQRWithLogo.png")
此示例将Visual Studio徽标添加到条形码中。 它会自动将其调整为适当的大小,以保持纯代码的可读性,并将该徽标与QR码方格网对齐,使其看起来更合适。 下一行代码将条形码着色为深绿色。 然而,它不会使标志褪色。
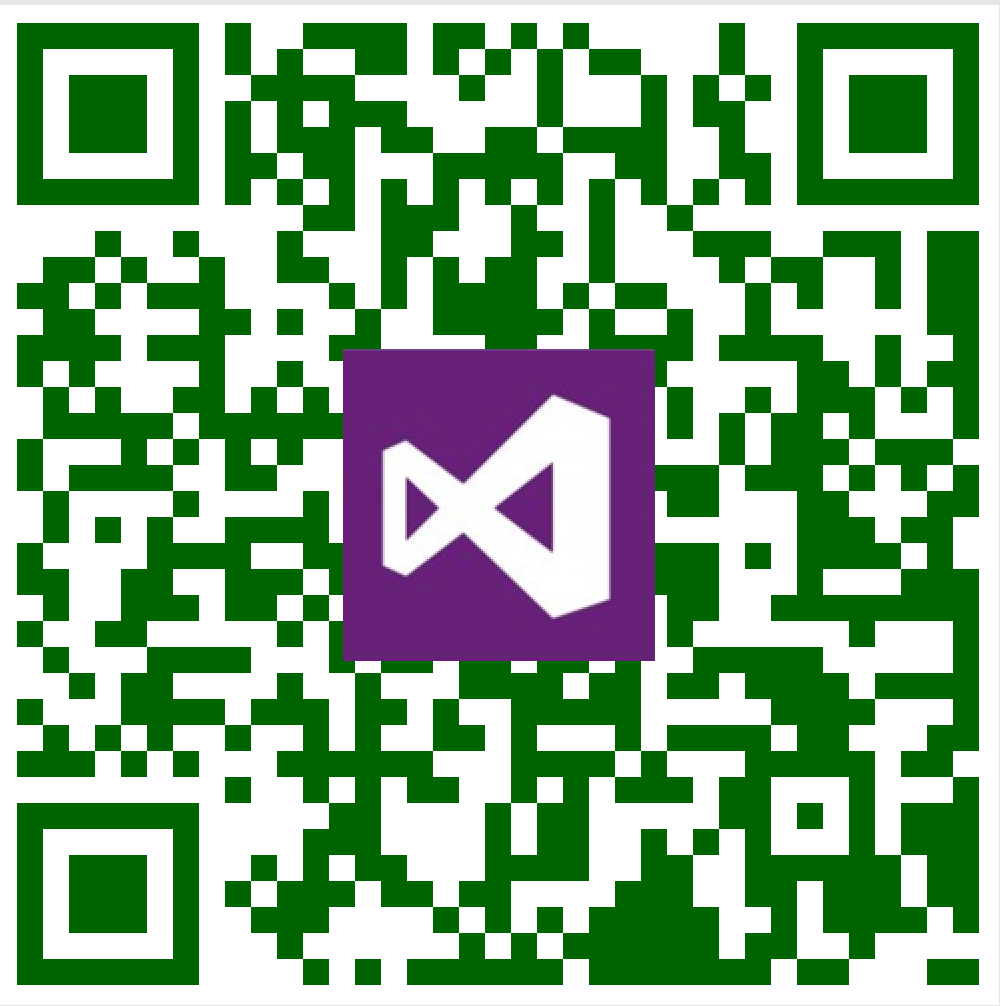
将文件保存为图片、PDF 或 HTML
最后,我们将该二维码保存为PDF。 最后一行代码将为方便起见在您的默认PDF浏览器中打开PDF。
:path=/static-assets/barcode/content-code-examples/tutorials/csharp-qr-code-generator-3.cs
using IronBarCode;
// You may add styling with color, logo images or branding:
QRCodeLogo qrCodeLogo = new QRCodeLogo("visual-studio-logo.png");
GeneratedBarcode myQRCodeWithLogo = QRCodeWriter.CreateQrCodeWithLogo("https://ironsoftware.com/", qrCodeLogo);
myQRCodeWithLogo.ChangeBarCodeColor(System.Drawing.Color.DarkGreen);
// Save as PDF
myQRCodeWithLogo.SaveAsPdf("MyQRWithLogo.pdf");
// Also Save as HTML
myQRCodeWithLogo.SaveAsHtmlFile("MyQRWithLogo.html");
Imports IronBarCode
' You may add styling with color, logo images or branding:
Private qrCodeLogo As New QRCodeLogo("visual-studio-logo.png")
Private myQRCodeWithLogo As GeneratedBarcode = QRCodeWriter.CreateQrCodeWithLogo("https://ironsoftware.com/", qrCodeLogo)
myQRCodeWithLogo.ChangeBarCodeColor(System.Drawing.Color.DarkGreen)
' Save as PDF
myQRCodeWithLogo.SaveAsPdf("MyQRWithLogo.pdf")
' Also Save as HTML
myQRCodeWithLogo.SaveAsHtmlFile("MyQRWithLogo.html")
验证二维码
在向二维码添加徽标和更改颜色时,我们要确保我们的二维码仍然可读。
通过使用GeneratedBarcode.Verify()
方法,我们可以测试我们的条形码是否仍然可读。
在以下代码示例中,我们将看到将二维码改为浅蓝色实际上会使其无法读取。 我们在代码中检测到这一点,并且更喜欢深蓝色。
:path=/static-assets/barcode/content-code-examples/tutorials/csharp-qr-code-generator-4.cs
using IronBarCode;
using IronSoftware.Drawing;
using System;
// Verifying QR Codes
QRCodeLogo qrCodeLogo = new QRCodeLogo("visual-studio-logo.png");
GeneratedBarcode MyVerifiedQR = QRCodeWriter.CreateQrCodeWithLogo("https://ironsoftware.com/", qrCodeLogo);
MyVerifiedQR.ChangeBarCodeColor(System.Drawing.Color.LightBlue);
if (!MyVerifiedQR.Verify())
{
Console.WriteLine("\t LightBlue is not dark enough to be read accurately. Lets try DarkBlue");
MyVerifiedQR.ChangeBarCodeColor(Color.DarkBlue);
}
MyVerifiedQR.SaveAsHtmlFile("MyVerifiedQR.html");
// open the barcode html file in your default web browser
System.Diagnostics.Process.Start("MyVerifiedQR.html");
Imports Microsoft.VisualBasic
Imports IronBarCode
Imports IronSoftware.Drawing
Imports System
' Verifying QR Codes
Private qrCodeLogo As New QRCodeLogo("visual-studio-logo.png")
Private MyVerifiedQR As GeneratedBarcode = QRCodeWriter.CreateQrCodeWithLogo("https://ironsoftware.com/", qrCodeLogo)
MyVerifiedQR.ChangeBarCodeColor(System.Drawing.Color.LightBlue)
If Not MyVerifiedQR.Verify() Then
Console.WriteLine(vbTab & " LightBlue is not dark enough to be read accurately. Lets try DarkBlue")
MyVerifiedQR.ChangeBarCodeColor(Color.DarkBlue)
End If
MyVerifiedQR.SaveAsHtmlFile("MyVerifiedQR.html")
' open the barcode html file in your default web browser
System.Diagnostics.Process.Start("MyVerifiedQR.html")
最后几行代码将二维码导出为独立的 HTML 文件,不含任何资产,然后在默认浏览器中打开该 HTML 文件。
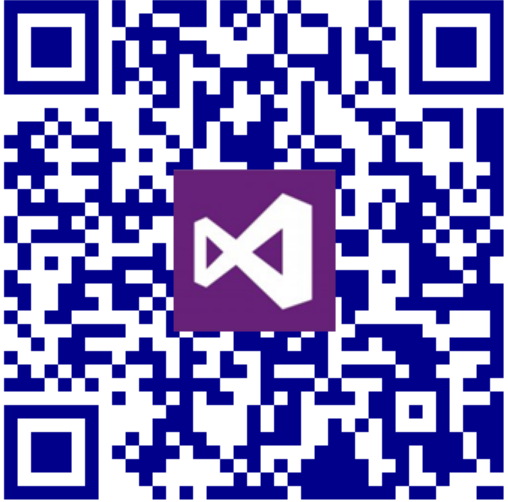
读取和写入二进制数据
QR 码是处理二进制数据的绝佳格式。 有时候,二进制数据比处理文本更节省空间或更合适。
在这个例子中,我们将从一个字符串编码一些二进制数据,将其写入QR格式的条形码,然后将其读回为二进制数据的位数组。 您会注意到这个功能在许多条码库中并不常见,这使得IronBarcode在这方面具有独特性。
:path=/static-assets/barcode/content-code-examples/tutorials/csharp-qr-code-generator-5.cs
using IronBarCode;
using System;
using System.Linq;
// Create Some Binary Data - This example equally well for Byte[] and System.IO.Stream
byte[] BinaryData = System.Text.Encoding.UTF8.GetBytes("https://ironsoftware.com/csharp/barcode/");
// WRITE QR with Binary Content
QRCodeWriter.CreateQrCode(BinaryData, 500).SaveAsImage("MyBinaryQR.png");
// READ QR with Binary Content
var MyReturnedData = BarcodeReader.Read("MyBinaryQR.png").First();
if (BinaryData.SequenceEqual(MyReturnedData.BinaryValue))
{
Console.WriteLine("\t Binary Data Read and Written Perfectly");
}
else
{
throw new Exception("Corrupted Data");
}
Imports Microsoft.VisualBasic
Imports IronBarCode
Imports System
Imports System.Linq
' Create Some Binary Data - This example equally well for Byte[] and System.IO.Stream
Private BinaryData() As Byte = System.Text.Encoding.UTF8.GetBytes("https://ironsoftware.com/csharp/barcode/")
' WRITE QR with Binary Content
QRCodeWriter.CreateQrCode(BinaryData, 500).SaveAsImage("MyBinaryQR.png")
' READ QR with Binary Content
Dim MyReturnedData = BarcodeReader.Read("MyBinaryQR.png").First()
If BinaryData.SequenceEqual(MyReturnedData.BinaryValue) Then
Console.WriteLine(vbTab & " Binary Data Read and Written Perfectly")
Else
Throw New Exception("Corrupted Data")
End If
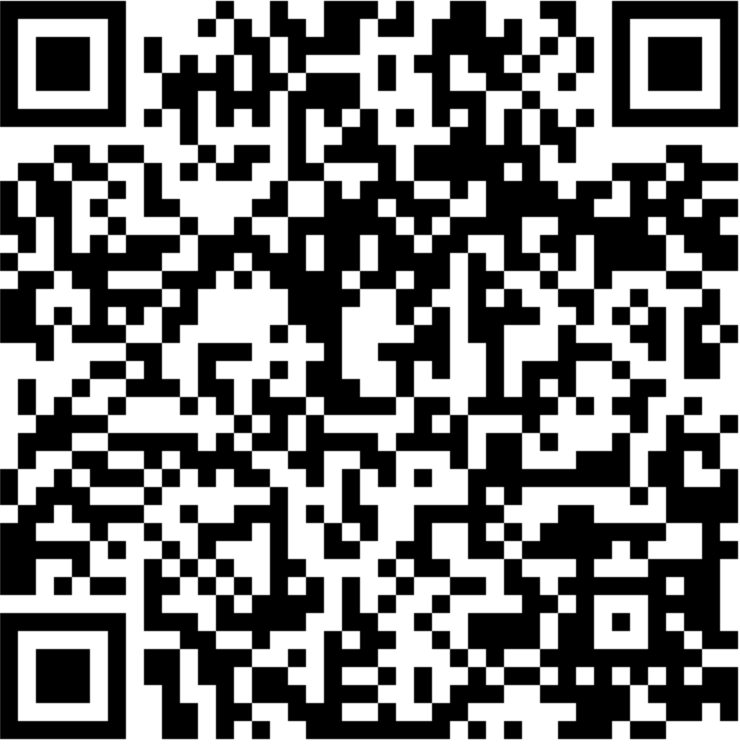
总之,Barcode C# Library 是专为实际QR码使用而设计的。 不仅可以快速准确地读取QR码,还允许我们对它们进行样式设计,添加标志,验证条形码,并用二进制数据进行编码。
读取二维码
为了避免您在教程之间跳转,我将添加一个代码示例,介绍我最喜欢的使用IronBarcode读取QR码的方法。
:path=/static-assets/barcode/content-code-examples/tutorials/csharp-qr-code-generator-6.cs
using IronBarCode;
using System;
using System.Linq;
BarcodeResults result = BarcodeReader.Read("QR.png", new BarcodeReaderOptions() { ExpectBarcodeTypes = BarcodeEncoding.QRCode });
if (result != null)
{
Console.WriteLine(result.First().Value);
}
Imports IronBarCode
Imports System
Imports System.Linq
Private result As BarcodeResults = BarcodeReader.Read("QR.png", New BarcodeReaderOptions() With {.ExpectBarcodeTypes = BarcodeEncoding.QRCode})
If result IsNot Nothing Then
Console.WriteLine(result.First().Value)
End If
还有一种更高级的形式,具有更多的开发人员控制功能:
:path=/static-assets/barcode/content-code-examples/tutorials/csharp-qr-code-generator-7.cs
using IronBarCode;
using System;
using System.Linq;
BarcodeReaderOptions options = new BarcodeReaderOptions
{
Speed = ReadingSpeed.Faster,
ExpectMultipleBarcodes = false,
ExpectBarcodeTypes = BarcodeEncoding.All,
Multithreaded = false,
MaxParallelThreads = 0,
CropArea = null,
UseCode39ExtendedMode = false,
RemoveFalsePositive = false,
ImageFilters = null
};
BarcodeResults result = BarcodeReader.Read("QR.png", options);
if (result != null)
{
Console.WriteLine(result.First().Value);
}
Imports IronBarCode
Imports System
Imports System.Linq
Private options As New BarcodeReaderOptions With {
.Speed = ReadingSpeed.Faster,
.ExpectMultipleBarcodes = False,
.ExpectBarcodeTypes = BarcodeEncoding.All,
.Multithreaded = False,
.MaxParallelThreads = 0,
.CropArea = Nothing,
.UseCode39ExtendedMode = False,
.RemoveFalsePositive = False,
.ImageFilters = Nothing
}
Private result As BarcodeResults = BarcodeReader.Read("QR.png", options)
If result IsNot Nothing Then
Console.WriteLine(result.First().Value)
End If
向前发展
要了解有关此示例以及在C#中使用QR代码的更多信息,我们鼓励您在我们的GitHub页面上fork它或从我们的网站下载源代码。
源代码下载
下载此C# QR码生成器教程和DLL。
更多文档
您可能还对QRCodeWriter和BarcodeReader 类在API参考中感兴趣。
他们记录了IronBarcode的完整功能集,这是一个VB.NET条码生成器,不可能全部装进一个单一教程中。